readlines() in Python
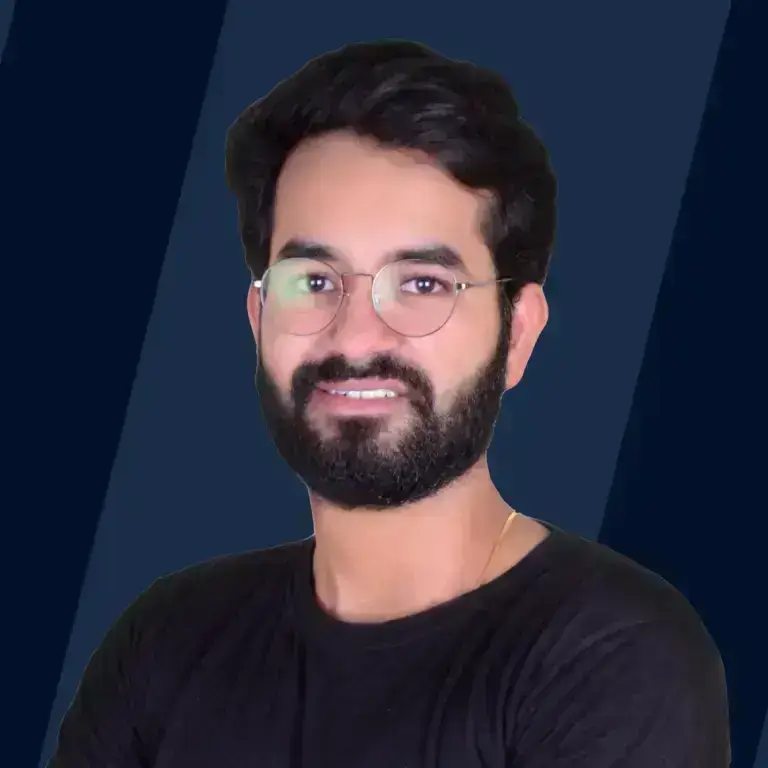
Overview
readlines() is a function in Python that helps us read all the lines simultaneously and return them as a string element. If we have to get the contents of any file as a string, readlines() will be the function of choice.
Syntax of readlines() function in Python
The syntax for readlines is
Parameters of readlines() function in Python
- There is only one hint parameter in the readlines() function; normally, we don't use the hint parameter, as it is reserved for special conditions.
- If more bytes are returned than the hint number while using hint, no additional lines will be returned. Every one of the lines will be returned if the value is set to default(-1).
Return Values of readlines() function in Python
- Let's say that there's a file in your computer called "myfile.txt". The readlines() function will return every line in the text file.
- Each line of the file is returned as a list by this function.
Exceptions of readlines() function in Python
- readlines() is one of the few functions in Python that don't have a lot of exceptions in runtime.
- The only exception that people sometimes find is the IOError.
- IOError stands for input/output error. When a file, file path, or OS activity we're referring to doesn't exist, it happens.
- IOError covers both the file not existing and inadequate permissions.
IOError for readlines() looks something like this:
Examples of readlines() function in Python
Example 1: Using readlines() function to read lines of file
In this example, we'll try to write into an empty file and then use the readlines() function to print the lines written from the file.
Code:
Output:
Example 2: Using the readlines() function to read lines of a text file
In this example, we will read lines from a text file.
Output:
What is readlines() function in Python?
The function readlines() reads every line in one go and returns each line as a string element in a list. By using readlines(), we can go over each element in the list and strip characters using the split() method. Small files can be utilized with this method since it reads the entire file's contents into memory before dividing them into distinct lines. In this article, we will go over the syntax, parameters, return values, and more regarding readlines().
readline() vs readlines()
- Unlike readlines(), readline() function only reads a single line from a file and returns it in the form of a string.
- This is how readline() works:
- This is how readlines() works:
- Both of them take an optional parameter, hint. The function will return the entire line if the hint is set to its default value of -1.
- The number of bytes to return can be specified using hint.
- Let's assume that we have a text file called file1.txt, with the following matter:
- Working with Python is very easy and reliable, as it is very robust in its working.
- Learning Python from Scaler Topics is amazing.
- Python syntax is very similar to the English language.
Using readline():
Code:
Output:
Using readlines():
Code:
Output:
Working with Python is very easy and reliable, as it is very robust. Learning Python from Scaler Topics is amazing.
Python syntax is very similar to the English language.`
Conclusion
In this article, we have covered the following topics:
- A basic overview of readlines() is and what it does.
- Syntax of readlines(). We also reviewed the hint parameter and how it affects readlines().
- IOError exception that arises in readlines().
- Code examples where we used readlines() on a text file.
- Difference between readline() and readlines().
Related functions
- readline()
- open()
- with()