Reference Variable in Java
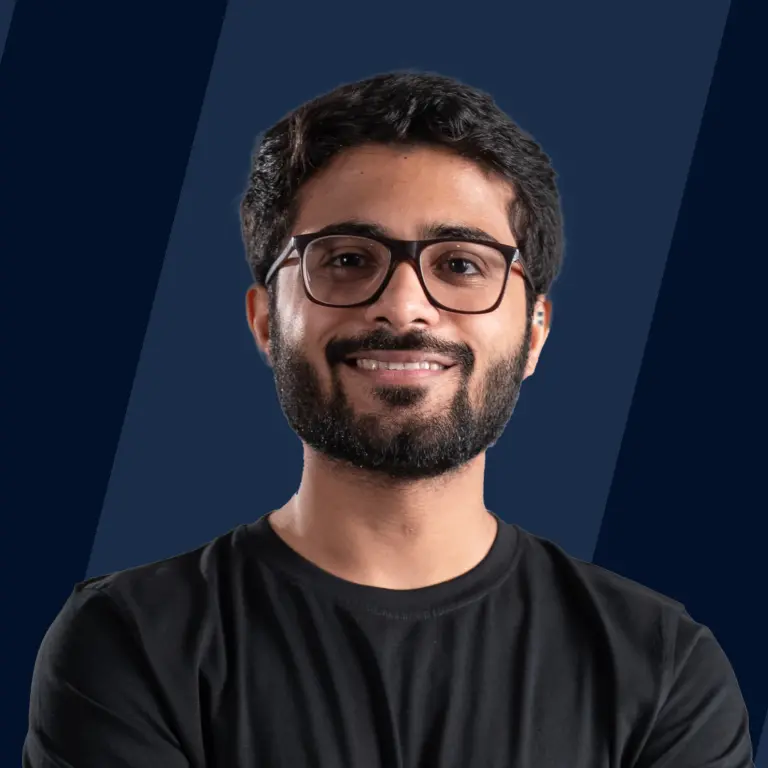
Overview
Every variable declared in Java has a data type like integer, float, string, etc. implies Java is a typed language. Now some of these types are primitive such as interger, character, boolean, etc. and the rest are reference data types which are derived from primitive data types. The variables that are declared as the reference type, therefore, are known as reference variables.
Object Creation and Reference Variables Basics
Every variable in Java is declared with a data type for example int num = 50. Here, int is the data type for the variable num which implies Java is a typed language. The declaration with data types helps in proper memory allocation and catching bugs in the early stages. Some languages like Python and JavaScript are dynamically typed and don't require explicit type declaration as Java or CPP.
Some of the data types are primitive and the rest are non-primitive. All the non-primitive data types are derived from primitive data types and are also known as reference types. The variable declared with non-primitive data types is known as reference variables.
Let us define a reference variable of the user-defined class. We will create a class called Car which has properties and functions.
The class defined has three properties name, model, and year. It has a constructor to initialize the Car object and a method displayInfo() to display information about the car.
Now we will create an object(instance) of the above class.
Output:
Explanation:
In the above example, we have created an object with various properties such as model, year, etc. This illustrates the concept of the reference variable in Java where myCar is a reference variable that points to the Car class in memory.
The object of the Car class is stored in the heap memory and the myCar variable just stores the reference to this instance of the Car class.
What is a Reference Variable in Java?
In Java, we have 8 primitive data types namely, char, boolean, byte, short, int, long, float, and double. Java has hundreds of built-in reference data types like strings, arrays, linked lists, etc. Reference data types can be inbuilt as well as user-defined.
When we declare a variable with primitive data type it stores the actual value inside the variable. But when we declare it with reference variables it stores the reference to objects or instances and therefore, these are called reference variables.
This property is visible when we create another variable by copying the existing variable. When we assign a reference variable to another variable, the other variable is simply pointing to the same object in the memory. The changes made to the other variable will also be reflected in the original variable.
This doesn't happen when we create a new variable of primitive type using an existing variable as it holds the value and not the reference. Hence, it copies the value, and any changes made are variable-specific, the original variable is unaware of any of the changes.
Reference variables can also be assigned null value, this indicates that right now they don't contain a reference to any object. This is often used to initialize the reference variable in Java before assigning actual values to it. It is always a safe choice to check if it is not null to avoid NullPointerException which is thrown when we attempt to access any null values.
On summarizing reference variables:
- Reference variables in Java point to the objects/instances.
- Various reference types in Java are classes, interfaces, arrays, enumerations, etc.
- These are derived from primitive data types.
- These are capable of holding a null value as well.
- We can access objects of various classes using their reference variables.
- On creation of an object using an existing object, rather than creating an entirely new object with similar properties, it simply copies the reference to an existing object in the heap memory.
Let us now see an example of the reference variable in Java.
Example 1
Output:
Example 2
As we discussed earlier reference variables can be null. It is very important to handle such edge cases using exception handling. The program must not crash in any condition is a key factor for a better user experience.
Let us see another example of a reference variable for the same class as we used above with NullPointerException.
Output:
To avoid this we can modify the code and add a try-catch block as follows:
Output:
In the example above, we access the object in the try block. In case an exception occurs catch block will be executed and the program will not crash.
Step-by-Step Analysis of the Example
Let us now see what is actually happening when we run the above code of
Example 1:
-
When we call new Student("Alice") the constructor that takes a single string as an input is called. This constructor creates an object of the Student with the provided values and returns its reference. This reference is stored in the variable student1.
-
Inside the constructor we are using the this keyword. The this keyword refers to the current object throughout the class.
-
The variables student1 and student2 stores the reference i.e. address to these objects. When we call some method or property on the variables as student1.name, using the reference it gets the object and then it fetches the value of the name property of the object.
-
Initially, student1 and student2 both reference the same Student object in memory. When we change the name of student1 to "Bob" using student1.name = "Bob";, this change is reflected in the object they both point to because they share the same reference.
Reference Variable as Method Parameters
We know that Java allows only the pass by Value mechanism for parameters passed to a method even when working with reference variables.
Primitive variables store the actual data value, while reference variables store references to the objects. When we pass a variable to the method parameter, the parameter value is copied and stored in the stack along with the method. This copy is then accessed by the method.
This implies that when a variable is primitive a new copy is passed and any changes made to it won't affect the original variable. But when the reference variable is passed to the method's parameter, its value i.e. address of the object is copied and this address is passed to the method parameter.
Note: Instead of creating a copy of the object whose reference is passed it simply creates the copy of the object's reference. This means that both the original reference variable and the method parameter point to the same object in memory. These are distinct copies of the same address.
Now, reference variables store the reference to objects, but when we pass such variables to methods any operations performed by the methods on these objects directly affect the object itself. Thus, this may result in data loss or modification.
FAQs
Q. How do reference variables differ from primitive variables in Java?
A. Reference variables store references to objects, while primitive variables store actual data values. Reference variables are used with objects and classes, while primitive variables are used with basic data types like int, char, etc.
Q. Can reference variables point to null in Java?
A. Yes, reference variables can be assigned the value null, indicating that they do not currently reference any object. This is often used to initialize reference variables before assigning them to actual objects.
Q. How is memory allocated for reference variables and objects in Java?
A. Reference variables are typically stored on the stack, while objects are stored on the heap. The reference variable stores the memory address of the object on the heap.
Conclusion
- Reference variables are the variables that store the reference to an instance of a class. Unlike primitives, these don't store a value instead they store an address.
- Reference variables can be of inbuilt data type or can be user-defined class instances. Reference objects are derived from primitive data types.
- Reference variables can hold null values as well. Assigning the value of one reference variable using another doesn't create a new copy instead both the original as well as new variable hold the reference to the same object in the memory.
- Reference variables are typically stored on the stack, while objects are stored on the heap. The reference variable stores the memory address of the object on the heap.
- Reference variables as method parameters in Java allow you to pass a copy of an object's memory address, not the copy of the object itself. This enables you to modify or access the object's state within the method while maintaining the original reference's integrity outside the method. Java follows "pass by value" even with reference variables.