How to Remove Duplicates from JavaScript Array?
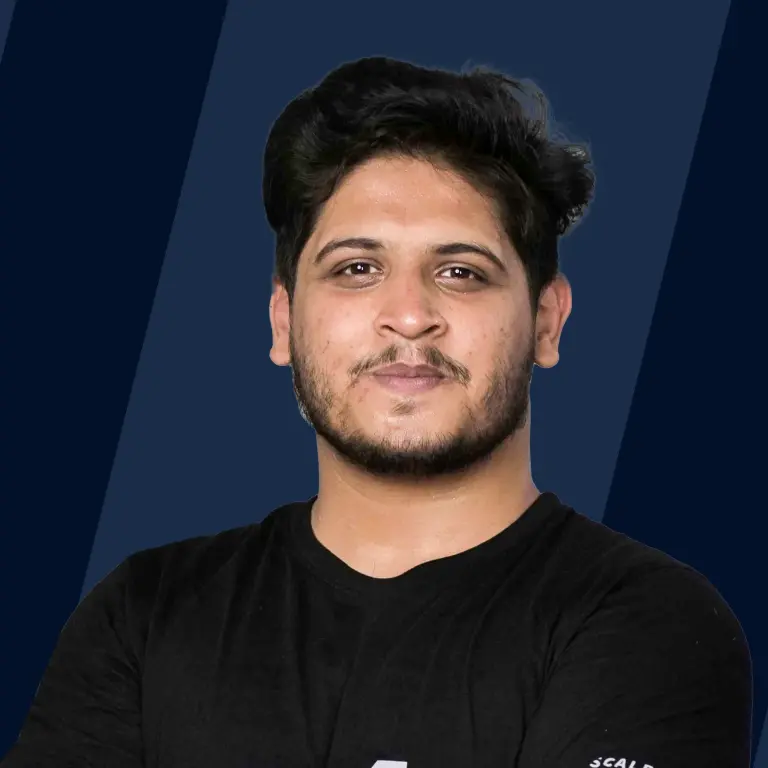
Overview
In this article, we will learn about how to Remove duplicates from array javascript. In an array, duplicates refer to elements that appear more than once. So, we'll see a lot of methods to eliminate duplicates from an array.
Introduction
Assume you have a list of student records, and two students have accidentally duplicated their records in a form. As a result, the duplication must be removed.
So let's see some ways to remove the duplicates from the array. We will use the following methods:
- indexOf() and push() Method
- filter() and indexOf() Method
- Set() Method
- forEach() and includes() Method
- reduce() and includes() Method
Using indexOf() and push() Method
The indexOf() method returns the first index of an array element occurrence. We can iterate over the array's elements, pushing them into the new array if they don't already exist in the resultant array.
Some of the key points are given below:
- The indexOf() method returns the position of the first occurrence of a value in a string.
- If the value cannot be retrieved, the indexOf() function returns -1, which means the element is not yet present in the array.
- The indexOf() method is case sensitive.
- The indexOf() method is immutable.
Syntax
Parameters
Parameter | Description |
---|---|
search_value | It is Required. The String you want to search |
start | It is Optional. Default value : 0 |
Return Value
Returns a number:
- The search-value appears in the first position.
- -1 if it never occurs.
The indexOf() method returns the index of an element's first appearance in an array.
For instance:
Output:
Let's look at a code to show how to remove duplicates from array javascript.
Output:
In the above program, the duplicate element is removed from the array:
- The forEach loop is used to iterate elements in the array.
- Then, every element is checked in the new array, if it is present, then it returns the index of the array, and if it is not present, it returns -1, as a result, the element is pushed in the array using push() method.
- The indexOf() method is immutable.
Using the filter() and indexOf() Method
The filter() method returns a new array of elements that satisfy the given condition. Only those elements for which true is returned will be included in the array. By just modifying our condition, we may remove duplicate values from the array.
Some of the key points are given below :
- The filter() method returns a new array containing elements that pass a function's test.
- For empty elements, the filter() method does not run the function.
- The original array is not changed by the filter() method.
- The filter() method is immutable.
Syntax
Parameter
Parameter | Description |
---|---|
function() | It is required. For each array entry, a function to perform. |
current_Value | It is required. This is the current element's value. |
index | It is optional. The index of the current element. |
arr | It is optional. The array of the current element. |
this_Value | It is optional. Default undefined A value passed to the function as this value. |
Return Value
Returns an Array:
- The items that pass the test are contained here.
- If no elements pass the test, the array is empty.
Let's look at a code to show how to remove duplicates from array javascript.
Output:
In the above program, the duplicate element is removed from the array :
- Here, we have used arrow function to define the function.
- Current Index -> indexOf(),
For value “A”, we have : 0 -> 0, 4 -> 0. (Equality doesn’t hold).
For value “B”, we have : 1 -> 1, 2 -> 1. (Equality doesn’t hold).
For value “C”, we have : 3 -> 3. (Equality holds a unique element). - Then filtered these unique items and stored them in a unique array, and returned them.
Suppose you want to find the duplicate element, you need to only reverse the condition.
Output:
Using a Set() Method
A set is a grouping of distinct values. The Set allows you to store unique values of any type, including primitive values and object references. To eliminate duplicates from an array, do the following:
- To begin, create a Set from an array of duplicates. Duplicate elements will be removed automatically by the new Set.
- The set should then be converted back to an array.
To remove duplicates from array javascript, use a Set in the following example :
Output:
Note:
- The spread operator ... is used to add all of the elements of the Set to a new array one by one.
- Set() is immutable.
The Various Set Methods are :
Method | Description |
---|---|
new Set() | A new set is created |
add() | Adds a new element to the set |
delete() | Removes an element from the set |
has() | Returns true if the value exists |
clear() | Removes all of the set's items. |
forEach() | For each element, a callback is triggered. |
values() | Returns an Iterator that iterates across all of the values in a set () |
Keys() | It is same as values() |
entries() | Returns an Iterator that iterates across the [value,value] pairs in a Set. |
Using the forEach() and includes() Method
We can iterate over the elements in the array using the forEach() method, and if the element does not exist in the array, we will push it into a new array.
Syntax
Parameters
Parameter | Description |
---|---|
function() | Required. For each array entry, a function to perform. |
current_Value | Required. This is the current element's value. |
index | Required. The current element's index number. |
arr | Optional. This is the current element's array. |
this_Value | Optional. Default undefined. A value is passed to the function as this value. |
Return Value
Syntax of includes()
Parameters
Parameter | Description |
---|---|
search_value | Required.The string to search for. |
start | Optional. The position to start from, the Default value, is 0. |
Return Value
Type | Description |
---|---|
A Boolean | true if the string contains the value, otherwise false. |
The following example iterates over the elements of an array, adding only the elements that aren't already there to a new array :
Output:
In the above program, the duplicate element is removed from the array:
- forEach() is used to iterate elements in the array.
- then includes() check whether the element is in the array or not and according to which it returns a Boolean value.
- Then push() method is used to add elements to the new array.
Using the reduce() and includes() Method
The reduce() method reduces the array's items and combines them into a final array using a reducer function that you pass.
Some Key Points are:
- For each array element, the reduce() method runs a reducer function.
- The reduce() method returns a single value: the total outcome of the function.
- For empty array members, the reduce() method does not run the function.
- The original array is not changed by the reduce() method.
- reduce() method is immutable.
Syntax
Parameters
Parameter | Description |
---|---|
function() | Required. For each element in the array, a function should be run. |
accumulator | Required. The function's initial_Value, or the value it previously returned. |
current_Value | Required. This is the current element's value. |
current_Index | Optional. The current element's index number. |
arr | Optional. The current element is part of the array. |
initial_Value | A value that will be used as the function's initial value. |
Return Value
To further understand the reduce() method, consider the following example :
Output:
As we can see now, the duplicate elements are removed from the array. The above example explains how we can remove the duplicates from the array using the reduce method in javascript.
Conclusion
- These methods can help us to remove duplicacy from the array.
- The indexOf() method returns the first index of an array element’s occurrence
- The filter() method returns a new array of elements that satisfy the given condition.
- A set is a grouping of distinct values. It can be used to eliminate duplicates from an array.
- We can iterate over the elements in the array using the forEach() method.
- The reduce() method reduces the array’s items and combines them into a final array.