Remove Elements from a JavaScript Array
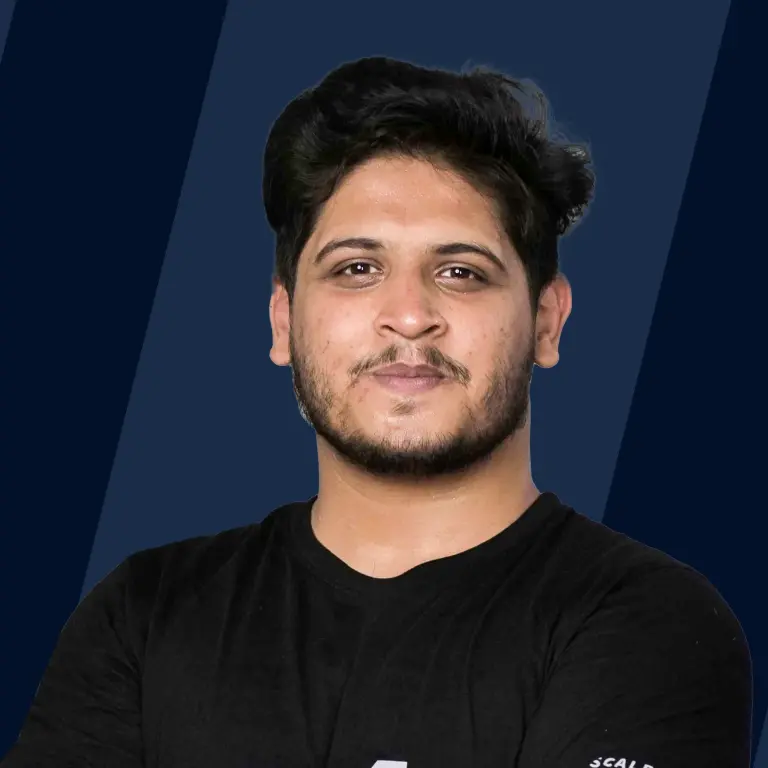
To remove an element from an array in JavaScript, use methods like pop to delete the last item, shift for the first item, and splice for any position. For more selective removal, the filter method creates a new array excluding unwanted elements, showcasing versatile ways to manage array content.
Methods to Remove Elements from JavaScript Array
Remove Last Element from Array using pop() Method
The pop() method is used to removes the last element from an array in JavaScript. This operation modifies the original array, reducing its length by one.
Example:
Output:
In this example, pop() is used to remove 'Cherry' from the fruits array. This method is part of the essential toolkit for manipulating arrays in JavaScript, especially when the task is to remove elements from an array.
Remove First Element from Array using shift() Method
To remove the first element from an array in JavaScript, the shift() method is used. It modifies the original array by removing the first item and returns that item. This method is particularly useful when managing data structures like queues.
Example:
Output:
- The first element, "Apple", is removed from the array.
- The modified array now contains ["Banana", "Cherry"].
This example illustrates the shift() method in action, showcasing a straightforward approach to remove element from array JavaScript.
Remove Element from Array at any Index using splice() Method
To remove an element from an array at any specific index in JavaScript, the splice() method is highly versatile. It alters the original array by removing or replacing existing elements and/or adding new elements in place.
Syntax:
- start: The starting index at which to start changing the array.
- deletenumCount: An integer indicating the number of elements to remove.
- item_1, item_2 ...: The elements to add to the array, if any.
Example:
Output:
- The element "Blue" is removed from the array.
- The modified array is now ["Red", "Green", "Yellow"].
This example demonstrates the splice() method's ability to remove element from array JavaScript at any given position, making it an essential tool for array manipulation.
Remove Element from Array with Certain Condition using filter() Method
To remove elements from an array based on some specific condition in JavaScript, the filter() method is an effective choice. It filters an array by applying a provided function to each element and returning a new array with all the elements that pass the test. effectively allowing for the removal of certain items.
Syntax:
- The callback function takes an element from the array as an argument and optionally the index of the element and the array arr itself.
- thisValue (optional) is a value to use as this when executing the callback.
Example:
Output:
- The new array contains elements [3, 4, 5], having removed numbers less than 3.
This example showcases how the filter() method can be used to remove element from array JavaScript when certain conditions are met, making it a powerful tool for conditional manipulation of array contents.
Remove Array Elements with Index using Delete Operator
Removing array elements by their index using the delete operator in JavaScript is technically possible but generally discouraged for array manipulation. The delete operator is used to removes a property from an object, and since arrays in JavaScript are objects with indices as properties, delete can remove elements. However, it leaves behind undefined at the removed element's index, creating sparse arrays (arrays with empty slots), which can lead to unpredictable behavior.
Syntax:
Example:
Output:
- "Banana" is removed, leaving undefined in its place, not shortening the array or shifting the indices of subsequent elements.
Why to Avoid Using delete for Arrays:
- It creates holes in the array, making it sparse.
- It may lead to confusions & errors in code, as the array length remains unchanged.
- Methods like filter(), splice(), or even setting elements to undefined explicitly are more predictable and maintain the integrity of the array structure.
For javascript remove element from array tasks, it's better to use methods specifically designed for arrays, like splice() or filter(), which adjust the array's length and maintain dense arrays without empty slots.
Remove Array Elements using Clear and Reset Approach
To entirely remove all elements from an array in JavaScript, the clear and reset approach offers a straightforward method. This technique resets the array to an empty state, effectively removing all its contents. It's particularly useful when you need to reuse the array variable without retaining any of its previous data.
Example 1 - Reassignment:
Example 2 - Setting Length to 0:
Another effective way to clear an array is by setting its length property to 0. This method modifies the original array instead of creating a new one.
Using these techniques allows you to remove element from array JavaScript in a manner that is both efficient and straightforward, ensuring the array is ready for new data without the need to create additional variables.
Remove Element from Array using for() loop and new array
Instead of using an inbuilt method of javascript, we can program a method to remove an element ourselves using the for loop.
Example:
1. Removing BMW from carBrands Array
Output :
Remove Array Element using the Lodash Array Remove Method
The Lodash library offers a convenient _.remove method for removing elements from an array based on a condition. This method changes the original array by removing all elements that satisfy the provided condition and returns an array of the removed elements. It's a powerful utility for operations that require conditional removal of array items.
Syntax:
- The function parameter is a predicate that is invoked with three arguments: (value, index, array).
- The predicate determines which elements should be removed based on the return value (true for removal).
Example:
Let's use Lodash to remove all even numbers from an array of integers.
Output:
- The original array numbers is modified to only include odd numbers [1, 3, 5].
- The removed array contains the even numbers [2, 4, 6] that were removed.
The Lodash _.remove method is particularly useful for remove element from array JavaScript tasks where elements need to be removed based on specific criteria, offering both modification of the original array and access to the removed items.
Conclusion
- Utilize pop(), shift(), and splice() for direct removal of elements from JavaScript arrays, tailoring to specific positions.
- Apply the filter() method for condition-based removal, crafting arrays with only desired elements.
- Explore using Lodash's _.remove for enhanced, condition-specific element exclusion, showcasing flexibility in how to remove element from array in javascript.
- Avoid the delete operator to prevent creating sparse arrays, ensuring cleaner and more predictable array structures.
- Clear entire arrays or reset them efficiently, demonstrating versatile strategies in javascript remove element from array practices for clean slate data handling.