removeAll() in Java
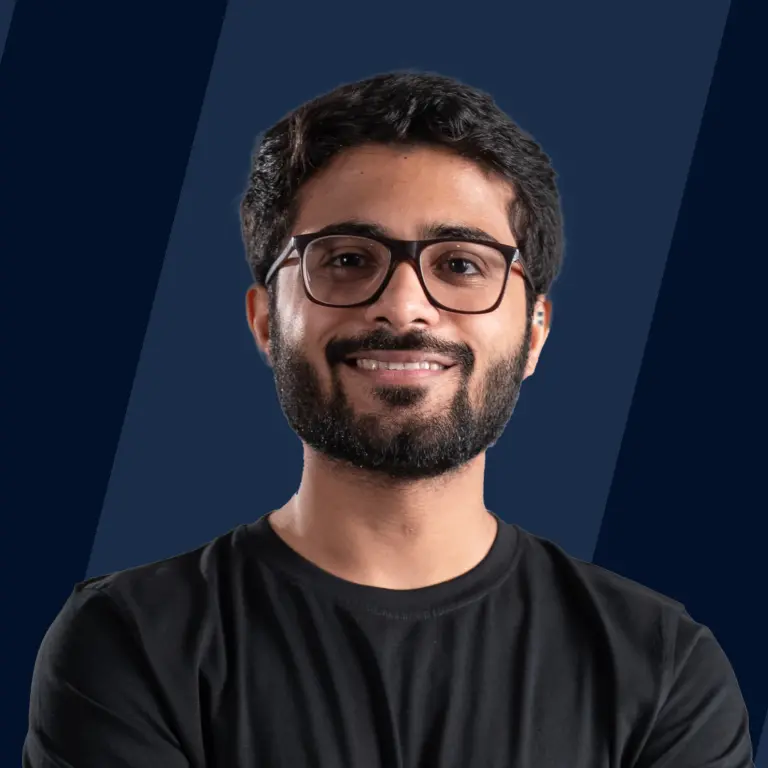
The removeAll() method in java removes the occurrence of all the elements in the ArrayList that are present in the specified collection. It belongs to the java.util.ArrayList class.
Syntax of removeAll() in Java
Parameters of removeAll() in Java
The removeAll() in java takes only one parameter:
- collection: It is a collection that contains elements to be removed from this list.
Return Values of removeAll() in Java
- The removeall() in java returns true if the elements are deleted from the ArrayList.
Exception for removeAll() in Java
- The removeall() in Java throws a ClassCastException when the classes of elements present in ArrayList and the class of elements in a specified collection are incompatible.
- The removeall() in Java throws a NullPointerException when the ArrayList that contains the null element and the collection passed as the parameter do not allow null elements.
Example of removeAll() in Java
Let us take a look at some examples to get a brief of removeAll():
Output:
Java ArrayList removeAll()
Imagine you are out with your friend to get groceries. Now, you've decided to divide the work to ease the tasks. You are supposed to get things from the first floor of the supermarket, and your friend will get things from the second floor.
After an hour, your friend arrives with the grocery items and a list with the names of the items he has. Thus, you decide to remove all those items from your main list to avoid duplication. Now, a question strikes. We've read that we can use ArrayList in Java to store a collection of items (e.g., grocery items). Is there a way to remove a certain collection from this collection of items? The answer is removeAll() in Java.
The ArrayList removeAll() method in Java removes all of the matching elements contained in the specified method argument collection. It removes every occurrence of matching elements, not just the first occurrence.
Following is the pictorial representation of removeAll() in java:
Time and Space complexity of removeAll() in Java
The removeAll() in Java determines which is smaller—the set or the collection. This is done by invoking the size() method on both.
- The time complexity of removeAll() if it searches in HashSet: O(n).
- The space requirement of removeAll() if it searches in HashSet: O(n).
If the collection has less number of elements than the given set, the replaceAll() method will iterate over the given collection with the time complexity of O(n). The replaceAll() checks if the given element is present in the set, and if the element is present, it removes it from the set using the remove() method of the set. Both of these operations are executed with the time complexity O(1). Thus, the overall time complexity is O(n).
If the set has less number of elements than the provided collection, the replaceAll() method will iterate over the given set using O(n). This will be followed by the replaceAll() method, which checks if each element is present in the collection by invoking its contains() method. If such an element is present, then the element is removed from the set. Thus, in this case, the complexity depends on the time complexity of the contains() method.
- The time complexity of removeAll() if it searches in ArrayList: O(n*m).
- The space requirement of removeAll() if it searches in ArrayList: O(1).
n is the total number of elements present in the ArrayList, and the lookup would happen in O(m) (m count of elements in the list).
Here, if the collection is an ArrayList, the time complexity of the contains() method is O(m), So for every element in n, we need to look at the element in the list you come up with O(n*m). Thus the overall time complexity to remove all elements present in the ArrayList from the set is O(n*m).
Example 1: Remove all elements from an ArrayList
Output:
Explanation of the example:
In the above example, we have created an ArrayList frameworks with three elements Spring, Spark, Vaadin. Now, the frameworks.removeAll(frameworks) will take frameworks as collection and remove all the elements in frameworks.
Example 2: For NullPointerException
Output:
Explanation of the example:
In the above example, we have created an ArrayList evenNumbers with four elements: 2, 4, 19, and 10. Now, the evenNumbers.removeAll(oddNumbers) will take oddNumbers as a collection. Since the value of oddNumbers is null, it will return java.lang.NullPointerException.
Example 3: Remove all Elements from an ArrayList Present in Another ArrayList
Output:
Explanation of the example:
In the above example, we are creating two ArrayLists, languages1 with four elements Java, English, C and Spanish and languages2 with three elements English and Spanish. Now, the languages1.removeAll(languages2) will take languages2 as collection and remove all the elements in languages1 that contain elements of languages2.
Example 4: Remove all Elements from an ArrayList Present in a HashSet
Output:
Explanation of the example:
In the above example, we are creating an ArrayList numbers with four elements 1, 2, 3 and 4 and a HashSet primeNumbers with two elements 2 and 3. The numbers.removeAll(primeNumbers) will take primeNumbers as collection and remove all the elements in numbers that contain elements of primeNumbers.
Conclusion
- The removeAll() is a java.util.ArrayList method.
- The removeAll() takes a collection as a parameter and removes all occurrences of the collection in the ArrayList.
- It returns true if the remove operation is completed successfully.
- It throws NullPointerException when the collection is null.
- It throws ClassCastException when the collection and ArrayList are incompatible.