removechild() JavaScript
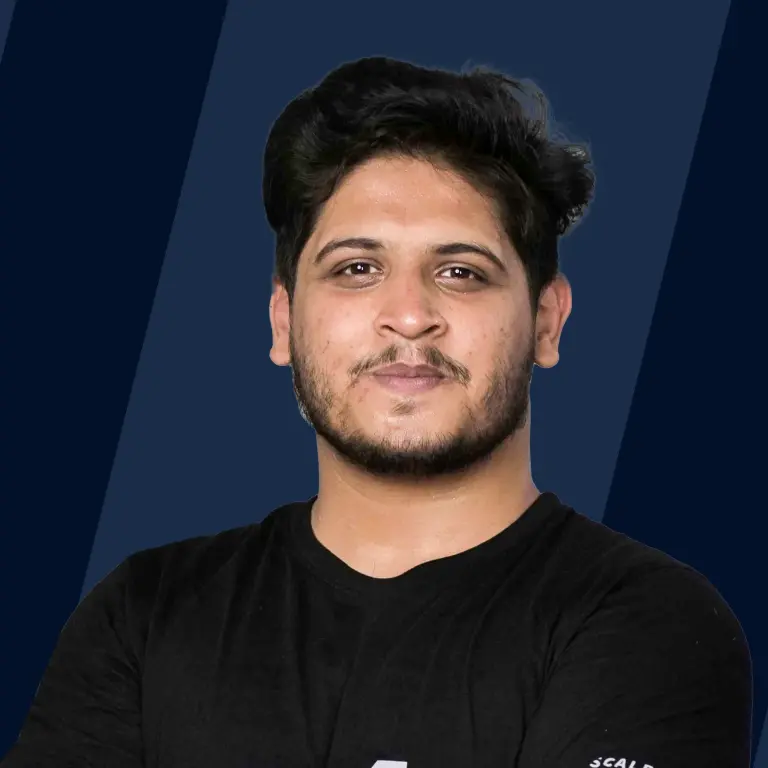
Overview
In the DOM (Document Object Model), the JavaScript removeChild() method is used to remove a child element from an existing parent element. In the JavaScript Node interface, the JavaScript removeChild() method is defined. The removed child can be inserted at a later time because we can store the reference returned by the method in a variable and then access it later.
Syntax
The syntax of the method is as follows:
Parameters of JavaScript removeChild()
The removechild in Javascript accepts only one parameter, child, which must be provided. It represents the node child that needs to be removed.
Return value of JavaScript removeChild()
The removechild in Javascript returns the removed node as a node object. In case the node doesn't exist, it returns null.
Exceptions of JavaScript removeChild()
There is no exception for removeChild() in JavaScript.
Example
Let's take an example to understand removechild in Javascript where we have a nested div inside a div element in our HTML document with some JavaScript.
Code
In the above example, we can use JavaScript removeChild() method to remove the child element inside the parent element.
The below code represents a parent element and a child element inside it.
Let's see the JavaScript code to remove the child element:
If we execute the above code, it gives the following output:
As you can see in the output above, the child element that was inside the parent element has been deleted. And at last, only the parent div will remain as <div id="parentID"></div>.
The child element and any other elements nested within the child element will be removed.
What is JavaScript removeChild()?
We have learned about JavaScript nodes. When we create a node, we refer to it as the root node, and the nodes that come from it are called child nodes.
It is possible to remove a child node from its parent node using JavaScript removeChild() method. Therefore, The JavaScript removeChild() method is used for removing a child node from its parent node.
There are several ways to remove a child element from the parent node. Let's look at them:
- Clearing innerHTML - Because this approach invokes the browser's HTML parser, it may not be suitable for high-performance applications.
- Looping to remove every lastChild - If it's faster to check for firstChild than lastChild, the loop continues to check for the firstChild.
More Examples
The following examples describe how the removeChild() method is implemented in practice.
Example 1: Remove some specific element
If you want to remove some specific element from the parent node, you can use the bracket notation in the childNodes property.
Code
Output
Explanation
In the above example, we have a list of languages. The getElementById() method returns an Element object from which you can retrieve the element whose id property matches the string you provide. We then use the removeChild() to remove the first child in the given list.
Example 2: Remove the last child element
Use the lastElementChild property of the parent node to remove the last child element.
Code
Output
Explanation
In the above example, we have a list of languages. The getElementById() method returns an Element object from which you can retrieve the element whose id property matches the string you provide. We then use the removeChild() to remove the last element in the list using lastElementChild.
Example 3: Remove the First Child Element
Use the firstElementChild property of the parent node to remove the first child element.
Code
Output
Explanation
In the above example, we have a list of languages. The getElementById() method returns an Element object from which you can retrieve the element whose id property matches the string you provide. We then use the removeChild() to remove the first element in the list using firstElementChild.
Example 4: Remove all child nodes
To remove all child nodes of a parent element, follow the below procedure.
Suppose we have the following elements in our HTML document:
Code and Explanation
You can use the while statement to remove all the <li> elements nested inside the <ul> element as shown below:
When you execute the code, it gives the following output:
The entire code can be found below:
Supported browsers
Here is a list of the browsers that support DOM removeChild():
Browsers | Supported versions |
---|---|
Safari | 1.1 and above |
Google Chrome | 1 and above |
Internet Explorer | 5 and above |
Firefox | 1 and above |
Edge | 12 and above |
Opera | 7 and above |
Conclusion
- JavaScript removeChild() method is used to remove a child node of a parent node.
- It takes one parameter i.e., the child node to be removed, which is mandatory.
- It returns the removed node and in case the node doesn't exist, it returns null.
- There are various properties of the parent node which can be used to remove a specific child of the parent node.