Rename a File in Python
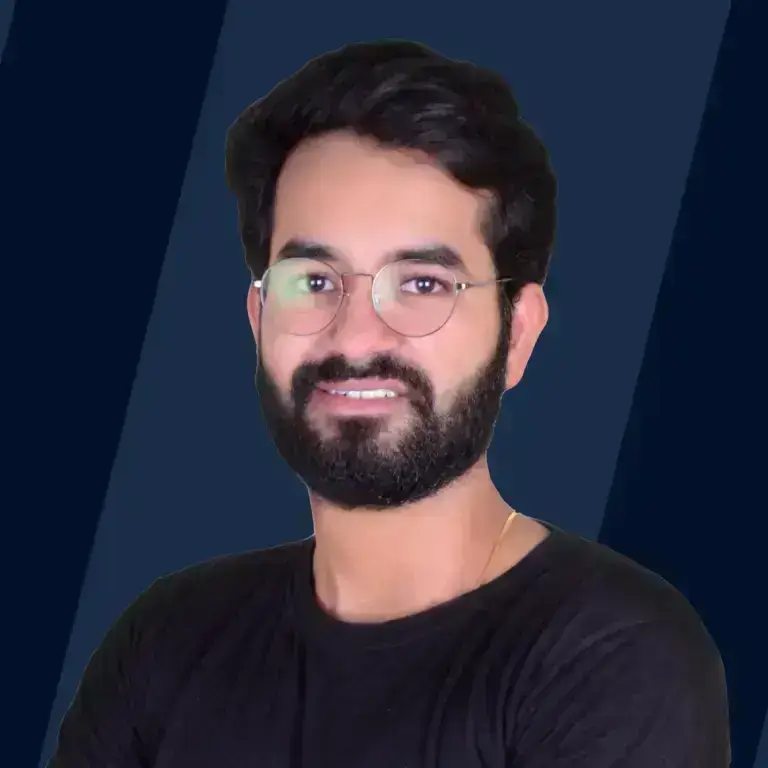
Often, programmers label a document or a file with something they might regret later and decide to modify. While it may seem more complex than simply renaming a folder on the computer, learning how to rename a file in Python is surprisingly straightforward.
There are various methods by which we can approach how to rename a file in Python. In this article, we will explore the different techniques on how to rename a file in Python, ensuring you have the knowledge to update file names efficiently and effectively.
How to Rename a Single File in Python?
Renaming files is a common task in file handling, and Python provides straightforward methods to accomplish this. Let's explore two primary ways on how to rename a file in Python: using the os.rename() method and leveraging the shutil module.
Using the os.rename() Method
The os.rename() method is a key technique for how to rename a file in Python; it changes the name of the source file or directory to the specified destination. It offers a direct way to rename file in Python but does not support moving files across different filesystems or storage devices. Always ensure the destination path does not exist, as this method can overwrite existing files without warning.
Syntax:
Parameters:
- src (string): The name of the file or directory to be renamed.
- dst (string): The new name of the file or directory.
Return Type:
The os.rename() method does not return any value.
Example:
Using the shutil Module
While os.rename() works well for renaming files within the same filesystem, the shutil.move() function can be used to rename files and has the added advantage of being able to move files between different filesystems. It's essentially like moving the file to a new path with a new name.
Syntax:
Parameters:
- src (string): The path to the source file.
- dst (string): The destination path. This can be a directory or a path with a new filename.
Return Type:
shutil.move() returns the path of the file after moving it.
Example:
How to Rename Multiple Files with Python?
Batch renaming of files can streamline the organization of file systems or prepare files for processing in automated workflows, highlighting the importance of knowing how to rename a file in Python. Below, we delve into methods using os.listdir() combined with a for loop and the glob module for efficiently renaming multiple files.
Using The os.listdir() Function With a For Loop
This method involves listing all files in a directory and then iterating over them with a for loop. Within the loop, you can apply renaming logic, such as adding a prefix, changing file extensions, or incorporating sequential numbers. It's a straightforward approach that gives you full control over the renaming criteria.
Syntax:
Parameters:
- path (string): The path to the directory whose files you want to list.
Return Type:
os.listdir() provides a list of names for all entries located within the directory specified by path.
Example:
Using The Glob Module
Using the glob module allows for pattern matching when selecting files to rename, which can be particularly useful when you only want to rename file in python of a certain type or matching a specific pattern. This method combines the flexibility of pattern matching with the power of Python's file manipulation capabilities.
Syntax:
Parameters:
- pattern (string): The pattern rule, which can include wildcards, for matching file names.
- recursive (bool): Option to match the pattern recursively in subdirectories (set to True to enable).
Return Type:
glob.glob() returns a list of pathnames that match the given pattern.
Example:
More examples
Renaming Files That Match a Pattern
To rename files matching a specific pattern, such as changing file extensions from .txt to .md, you can use the glob module for pattern matching and os.rename for the renaming process.
Code:
Output:
Every .txt file within the given directory has been renamed to have a .md extension.
Renaming All the Files in a Folder
If you need to rename all files in a folder, perhaps adding a prefix to each, you can iterate through all files using os.listdir() and os.rename().
Code:
Output:
Every file in the directory now starts with the prefix 'prefix_'.
Renaming Only the Files in a List
To rename only specific files listed in a Python list, check if the current file is in your list before renaming it.
Code:
Output:
Only 'file1.txt', 'file2.txt', and 'file3.txt' are renamed to start with 'renamed_'.
Renaming and Moving a File
To rename and move a file to a new directory, you can use shutil.move(), which handles both actions simultaneously. This is useful for organizing files into new locations.
Code:
Output:
The file 'old_name.txt' is moved and renamed to 'new_name.txt' in a new directory.
FAQs
Q. How do I rename a directory in Python?
A. To rename a directory in Python, you can use the os.rename() function from the os module. This function works for both files and directories. Ensure the target directory name does not exist to avoid overwriting.
Example:
This operation renames the directory from 'old_directory' to 'new_directory', without producing a direct output.
Q. Can I use pathlib to rename a file?
A. Yes, the pathlib module, introduced in Python 3.4, provides an object-oriented interface for working with filesystem paths. The Path.rename() method can be used to rename a file.
Example:
The file 'old_file_name.txt' is renamed to 'new_file_name.txt'. No direct output is produced, but the operation is reflected in the filesystem.
Q. How Can I Change File Extensions in Python?
A. To change the file extension of files in Python, you can use the os module to list files and then rename them with the new extension. For a more pattern-based approach, the glob module can be particularly useful.
Example using os and os.path:
This code snippet changes the extension of all .txt files in the specified directory to .md. There's no direct output, but the changes are applied to the files in the directory.
Conclusion
- Python offers multiple methods for renaming files, catering to various needs—whether it's a single file, multiple files, or complex patterns.
- The os and shutil modules provide simple yet powerful tools for file renaming, demonstrating Python's ease of use for file system operations.
- For more complex renaming tasks, such as changing file extensions or selecting files by pattern, the glob module is invaluable.
- The pathlib module offers an object-oriented interface for file system paths, allowing for elegant and expressive file manipulation.
- These methods can easily be adapted to suit a wide range of file management tasks, making Python a go-to choice for automating and streamlining file handling.