Java String replaceAll()
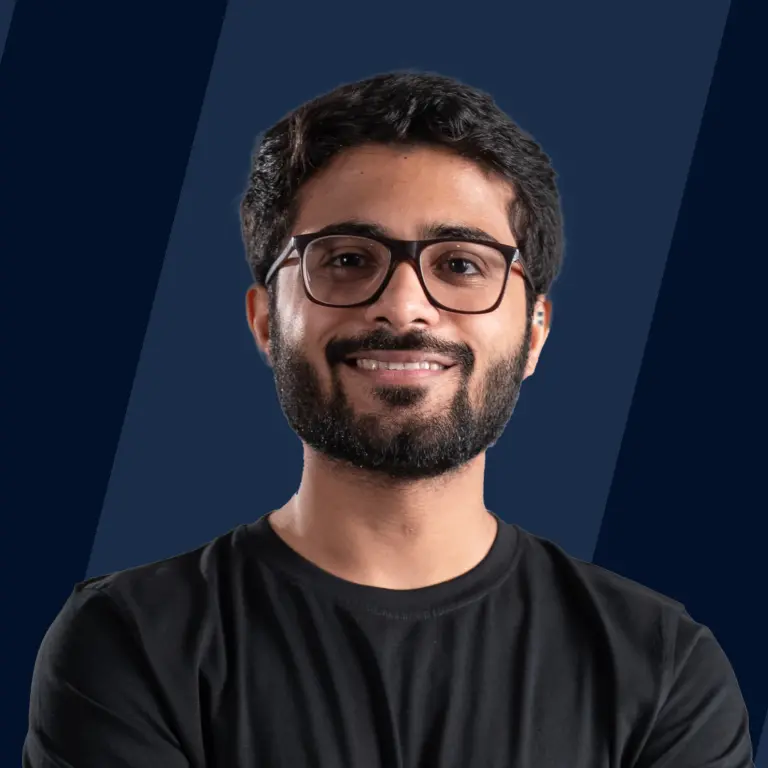
Overview
The replaceAll() in java is the method of String class that is used to replace each substring that matches the regex of the string with the specified text, another string passed as a parameter. It returns a new string with the changes done.
Syntax of replaceAll() in Java
Method signature for replaceAll() looks like:
Here String represents the String class of java, and regex and replacement are parameters.
Syntax:
Parameters of replaceAll() in Java
replaceAll() in Java takes two parameters:
regex: regular expression
replacement: replacement sequence of characters
Return Values of replaceAll() in Java
return type: string
The replaceAll method returns a new string with all the characters in the original string that match with regex replaced with the string passed as the parameter.
Exceptions of replaceAll() in Java
The replaceAll() method in java throws a PatternSyntaxException which is an Unchecked exception thrown to indicate a syntax error in a regular-expression pattern which is passed in the method as a parameter. This usually happens when the regex parameter that we're passing is syntactically incorrect.
Following are the four major ways to handle this exception :
- public String getMessage(): It contains the description of the exception.
- public int getIndex(): It will return the index for the error.
- public String getPattern(): It will provide us with the regular expression which contains the error.
- public String getDescription(): It will provide us with decryption with respect to the error.
Example:
Output:
Explanation of the example:
In the above example, all the occurrences of the character are replaced by the character .
What is replaceAll() in Java?
Imagine we are writing an article on java, and after the completion of the article, someone tells us to replace all the words 'java' with 'Java'. Now we can individually check all the words and replace them. But it will take a lot of time, right? To tackle this, most text editors have a feature to replace all the occurrences of a word with another word.
We know that in java, the text items are taken input as strings. Now, what if the above problem occurs with the java string? Well in such cases we can use the replaceall() in java.
The replaceAll() in java is the method present in the String class. It takes two parameters:
-
A regular expression: A regular expression is a sequence of characters that is used to denote a search pattern in the given string. They can be used to search, edit, or manipulate text and data. Java provides the java.util.regex package for pattern matching with regular expressions. Following
Regex Description \w Matches the word characters. \W Matches the nonword characters. \s Matches the whitespace. \S Matches the non-whitespace. \d Matches the digits. \D Matches the non-digits. \A Matches the beginning of the string. \z Matches the end of the string. -
A replacement string: This is a user-defined string that is used to replace the characters in the string which were looked up by the regular expression.
The replaceAll will match the string items with the parameter it takes. All the items that match the expression will get replaced by the input we pass to the method as a replacement string. Then the replaceAll in java will return a new string with all the characters of the string that match the first parameter replaced by the second parameter.
Following is a pictorial representation of the replaceAll function in java:
The replaceAll in java is present inside the String class (java.lang.String) package.
Example 1: Java String replaceAll()
Output:
Explanation of the Example
In the above example, the given two strings are "a string is traditionally a sequence of characters, either as a literal constant or as some kind of variable" and "My password is: 985630". For the first replaceAll function, "a" is given as the first parameter, which is a string, which will be replaced by "the"(for all occurances). Thus the replaceAll method in java will return a new string as output.
Similarly, the second replaceAll function is given \\d+ as the first parameter, which is the regular expression for sequence of digits, which will be replaced by "*". Thus the replaceAll method in java will return "My password is: ".
Example 2: Java String Remove White Spaces
Output:
Explanation of the Example
In the above example, the given string is "This is a sample string.". The replaceAll function is given "\\s" as the first parameter which is the regular expression for whitespace, which will be replaced by ""(no space). Thus the replaceAll method in java will return "Thisisasamplestring.".
Example 3: Java String Replace the Word
Output:
Explanation of the Example:
In the above example, the given string is "Jva is a programming language. Jva h..". The replaceAll function is given Jva as the first parameter which is the string that will be replaced by "Java". Thus the replaceAll method in java will return "Java is a programming language. Java has evolved from humble beginnings to power a large share of today’s digital world. Java was first released by Sun Microsystems in 1995.".
Escaping Characters in replaceAll()
As discussed earlier, the replaceAll in java takes regular expression as the first parameter. It is because a typical string in itself is a regex. These regular expressions have metacharacters.
A metacharacter is a character that has a special meaning during pattern processing of the regular expression. Metacharacters are used in regular expressions to define the search criteria and any text manipulations
Following are the metacharacters used in a regular expression:
Since these characters are not treated as general characters, thus In order to match the substring containing the metacharacters, we need to use either \ to escape these characters or use the replace() method in java.
Output:
Explanation of the Example:
In the above example, we are given the string "+first-+second". Now since we want to replace + which is a metacharacter thus we would use escaping characters. Thus the replaceAll method will output "#first-#second"
Conclusion
- The replaceAll in java is a method used to replace all the occurrences of a particular character in a string with other characters.
- The replaceAll in java is present inside the String class.
- replaceAll() takes two parameters regex and replacement strings and returns a new string with all the characters in the original string that match with regex replaced with the string(replacement) passed as the parameter.
- The replaceAll in java throws a syntax error: PatternSyntaxException when there is an error in the regular expression.
- The replaceAll in java is generally used when we have to replace all occurrences of a text with some other text piece.