JavaScript String replaceAll() Method
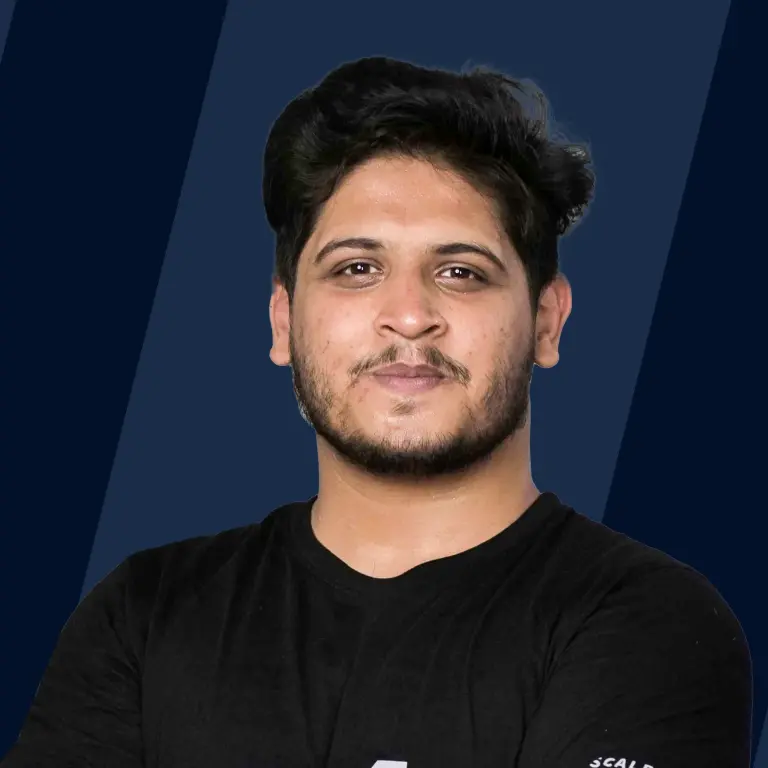
Syntax of ReplaceAll() in JavaScript
The Syntax of String replaceAll() is:
Here,
- finalString is our required string returned by the right-side expression.
- initialString is the string on which we want to perform replacements.
Let’s see the parameters which we have to pass in the replaceAll() function:
Parameters of ReplaceAll() Method in JavaScript
For replaceAll in javascript, we are going to pass two parameters first one is the pattern and the other is the replacement and the replaceAll() function is going to replace every pattern with replacement in the given string.
pattern:
For replaceAll in javascript the pattern can be a string or a regular expression which replaceAll in javascript function is going to find in the given initialString and replace with replacement the another given parameter.
replacement
For replaceAll in javascript the replacement can be a string or a function cthat will return a regular expression or a string and invoked at each match of the pattern with any substring.
Return Value of ReplaceAll() in JavaScript
replaceAll() function creates a new copy of the given initial string and returns it after performing replacements over it. So, replaceAll in javascript returns a string object.
Exceptions of ReplaceAll() Method in JavaScript
replaceAll in javascript doesn't throw any error for the string as a pattern to replace, but if we have a regular expression or ‘regex’ pattern then we must have to make it with a global flag otherwise this function will throw an error.
Example:
Output:
The above code shows us the same that we cannot pass a regular expression as a pattern if it is with a non-global flag.
Example of ReplaceAll() in JavaScript
Let’s see the working of the replaceAll() function by an example:
Output:
In the above code, we declared an initial string, pattern to replace, and replacement for the pattern. By using the replaceAll() method on initalString we got our finalString in which all the patterns are replaced by the given replacement. At last, we printed the finalString. replaceAll in javascript is case sensitive as we can see all the ‘word’ are replaced and don’t matter whether there are any suffixes or prefixes present. Also, there is no effect on ‘Word’ due to case sensitiveness.
What is String replaceAll() in Javascript?
String replaceAll() function in javascript is used to replace all the occurrences of the given pattern (could be a string or regex) with a given replacement that could be possibly a string or a function which is invoked at every match of pattern with substring and this function returns a string. replaceAll() function doesn’t affect our initial string and creates a copy of it and returns it after performing modifications.
ReplaceAll() function is case sensitive (as we have seen in the above example) but we can perform case insensitive modifications/replacements also by using regex with the ‘i’ switch.
ReplaceAll function in javascript starts finding substrings from the left side of the initial string and when a substring matches a given pattern it replaces that substring and starts finding from the next index and doesn’t visit the same index again, let’s take an example to understand better:
Let us have a string “ababa” and we want to replace the substring “aba” with replacement “sad”. Here we have two substrings from the initial string equal to pattern “aba” that are starting from index 0 and 2 of length three. But in the final string, we will only get the replacement of substring starting from index 0, because it will be encountered first and changed, so after the first change we will get “sadba” and now we are going to check from index 3 because we have passed the index 2 in previous change.
There is another case where after replacement a substring could be formed equal to pattern due to replacement as its index is passed we cannot include it, for example:
Let us have a string “abcbc”, pattern “abc”, and replacement “aba”, in this case after the first replacement we will get “ababc” now substring from 2nd index is equal to the pattern but it will not be considered because index 2 is already checked/passed.
Specifying a String as a Parameter
We have to pass replacement as an argument to replaceAll() function to replace the desired pattern. replaceAll in javascript can have a replacement be a string or a function. In this section let’s look over the special type of strings that have a special type of behavior.
Replacement | Replacement we will get |
---|---|
"$$" | This will only insert one "$" as a replacement |
"$&" | This will replace the same matched substring or we can say the pattern itself the number of times we add this |
"$'" | This will replace the current string with the portion of the sub-string from its right to the last index |
"$" | This will replace the current string with the portion of sub-string from the first index to just the left index of the matched pattern |
"$n" | If we have a regular expression as the pattern which to replace then we can specify that substring in nth parentheses will work as a replacement. Here n can go up to 100 and it’s 1-indexed |
Let’s see an example of the above-discussed replacements for better understanding: |
Output:
In the above example, we have passed all the special strings given in the above table as an argument to the replaceAll() function.
- First, we passed the string “\$\$” as a replacement but in the final string only a single "\$" we got.
- Secondly, we passed "$&" as replacement three times, and every pattern that is "cat" got replaced by “cat cat cat” that is three times repeated.
- In the third case, we passed “$'” as a replacement, and the first “cat” is replaced by a substring that follows it (“cow cat pig”) and the second “cat” is replaced by a substring that follows it (“pig”)
- In the fourth case, we passed “$” as the replacement and the first “cat” is replaced by the substring which precedes it (“dog”) and the second “cat” is followed by its preceded substring that is “dog cat cow”.
- In the fifth case, we created a pattern of regular expression and passed replacement as “$n” by taking n equals 2. As here nth element is indexed-1 . So we will get a second string that is “a” as replacement.
Specifying a Function as a Parameter
We can pass the function as a replacement argument to the replaceAll() function, but there is one more thing that we can pass some special arguments to the passed function itself. As we have discussed earlier every match function is invoked, so we can get some special results at every call. Let’s discuss the parameters of the replacement function first:
Argument | Description |
---|---|
match | match is the sub-string from the initial substring which matches with the current value of the pattern |
p1, p2, .. | For this argument of the replacement function, the first argument of the replaceAll() function must be a regex. For example if /(a)(b)+/ is given, p1 is (a) and p2 is (b). |
offset | offset gives the starting index of the matched substring |
string | string is just the complete initial string on which we are doing modifications |
The number of the arguments of the replacement function depends upon the pattern we passed to the replaceAll() function.
Let's see an example to see the working of the above-defined arguments:
Output:
In the above code, we created a regular expression with g and i modifiers to get global search and case-insensitive searching. As per our pattern when a substring of that starts with ‘C’ or ‘c’(due to ‘i’ modifier) followed by ‘a’/‘A’ and then followed by any substring which contains only lowercase alphabets (that is [a to z]) or uppercase alphabets (again, due to case-insensitiveness). Our replacement function was called three-time, two times when ‘Cat’ is matched and one time when ‘cam’ is matched. Each time it printed the respective ‘match’, ‘p1’, ‘p2’, ‘p3’, offset, and string value.
More Examples
-
Example 1
In the below given example, we will take a string and regex as a pattern to replace and a string as a replacement.
Output:
In the above example, we declared a string on which we will perform replacements. After that, we have taken a normal string and a regular expression with global mode as the pattern and after using the replaceAll() function over the initial string printed the final strings.
Example 2
In the below given example, we are going to perform Case-Insensitive Replacement in a string.
Output:
In the above code, we have defined the pattern as a regular expression with global as well as i mode which leads to having a case-insensitive replacement. We have used a simple string as a replacement also to compare the result.
Example 3
In this example, we are passing Function as a Replacement
Output
In the above code, we have used a function as a replacement which will be invoked when our pattern will match the particular digit, and at every call, it will replace it will a random digit.
Note: In the above function we are using the random function to replace, so the result may vary with each call.
Conclusion
- ReplaceAll() is a function used to replace all the substrings that match a given string or regular expression (pattern) with the provided string (replacement)
- ReplaceAll in javascript function makes a new copy of the initial string and after performing replacements over it, it returns the final string while the initial string remains the same.
- If we pass a regular expression as a pattern then it must be defined with a global mode otherwise it will through an error.
- Usually, the replaceAll() function performs case-sensitive replacement, but if the pattern is a regular expression with i mode then it will perform the case-insensitive replacement.
- If the replacement is a function then it will be invoked at every match and we can pass it some special types of arguments to get the index it matched the current substring to match, and many more things.
See Also
- replace(): Return the string after replacing the first substring which matches the pattern.
- Match(): Retrieves the result of matching a string against a regular expression.
- exec(): Executes a search for a match in a specified string.
- test(): Executes a search for a match between a regular expression and a specified string.