Return Statement in Java
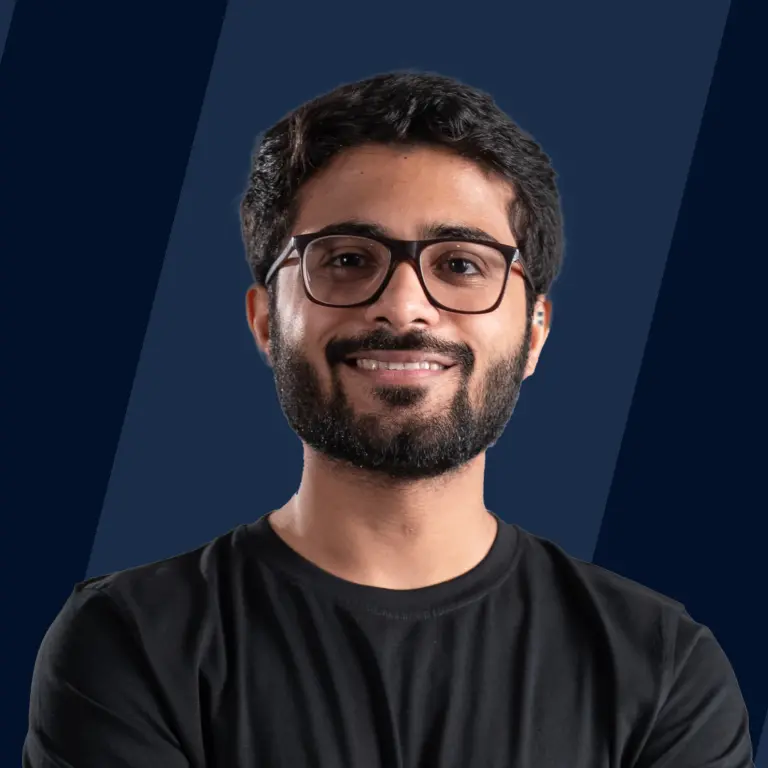
Overview
In programming, the return statement is quite crucial for directing the execution of code and for returning values from methods. It is an effective tool for creating well-structured, modular programs. Programmers need to understand return statements because they provide a way for functions to communicate and share data with other parts of the program. They are used to send a value back from a function to its caller which is a crucial part of any programming language. In this article, we will study about the return statement in Java along with examples.
Return Statement in Java
In Java, return is a keyword that is used to exit the program whenever encountered. Return statement in Java is used to return a certain value from a code block when the execution n of that block is completed. Moreover, it is also used in a loop to stop the program from running further. If a loop encounters the return statement, the program execution gets stopped and further statements are ignored by the compiler. The return statement is particularly useful when you want to provide a result or outcome from a method's operation.
The Structured programming approach places a strong emphasis on using structured control flow techniques to make code more understandable and effective. Return statements and structured programming work well together because they offer a clear framework for creating well-formed, manageable, and testable code. By enabling functions to encapsulate certain functionality and transmit results to the calling code, return statements play a vital role in achieving modular design.
Note: The return statement not only allows functions to return the results to the calling code but also serves as a mechanism to gracefully exit functions and, ultimately, the entire program.
Use Cases of Return Keyword in Java
There are two usages of return keyword in Java -
Methods which return a value
The methods that define a return type in the function definition must specify the return statement with a return value. However, if the return type is void in the function definition, then the return statement could be avoided or is not required to be followed by a return value.
Let us understand the return keyword with a return value with the help of a code example.
Output:
In the above code example, we have implemented a function to calculate the sum of two values. The function sum returns an integer value, therefore a return statement is followed by a return value of the sum.
Methods which does not return a value
There are also methods that do not return any value. However, for methods, two cases arise-
-
Method not using return statement with a void return type
In this type of method, instead of returning anything the program prints the answer in the method only and the program ends there. Therefore, there is no need to use a return statement.
Let us understand it with the help of a code example.
Output:
In the above code example, we have implemented a sum function which has a void return type. Inside the function, we have used a print statement to print the results instead of returning them.
-
Method using return statement with a void return type
In these types of methods, the return statement is used to exit the program.
How to Return a Value from a Method in Java
In Java, every method has a return type such as int, double, void, string, etc. If the return type of a function is anything except void, then the method has to return a value. One thing to remember is that the value that is returned from the method must be of the same type as the return type of the method.
However, for a void return type, the method does not return a value.
Syntax
The syntax for returning a value from a method is as follows:
As you can see in the above syntax, it is just the return keyword followed by the value to be returned.
Illustrations
Let us see some examples that will demonstrate how to return a value from a method in Java.
Output:
In the above code example, we have implemented a method of greatest number between the two numbers. The method greatest has a return type of int, therefore, at the end of the program, we have returned the integer number which will be greatest among the two.
Returning an Object (Class) in Java
The return type of a method can be a class as well. However, in that case, the value returned must have a type of the object of that class.
Similar is the case for an interface, an interface can be a return type but in that case, the returned object must implement the methods of that interface.
Syntax
The syntax for returning an object in Java is as follows:
As you can see in the above syntax, the return keyword will be followed by the name of the object of the class.
Illustrations
Let us see some examples that will demonstrate how to return a value from a method in Java.
Output:
As you can see in the above code example, we have implemented a class Shapes in which we have a constructor and two methods of display(). In the main method, we have called a display() function. Inside the display2() method, we have made a call to the other display function in which we have returned an object of the class Shapes. Further, we have displayed this returned object value to the console.
FAQs
Q. What does the void return type mean in a method definition?
A. It means that the method will not return anything.
Q. Can the return statement be used in loops and conditional statements?
A. Yes, the return statement can be used in the loops and conditional statements to exit the program.
Q. What is the use of return statements in Java?
A. The return statement is used to exit the program execution or return a value from a method.
Q. Can a method return an object in Java?
A. Yes, a method can return an object of a class in Java. This is particularly useful when you want to provide more complex results to the caller.
Conclusion
- The return is a reserved keyword that is used to exit the program execution.
- The return statement is used to return a value from a method.
- The values that are returned from a method should be of the same type as the return type defined in the method signature.
- There is no need to use a return statement when the return type of the method is void.
- Return statements could be used in loops and conditional statements to stop the execution of the program. The statements after return would be ignored by the compiler.
- There should be a return statement when the return type of a function is anything except void.
- A return statement can also return an object of a class from a function.
- The return statement can return only one value from a function.