Program to Reverse a Number in Java
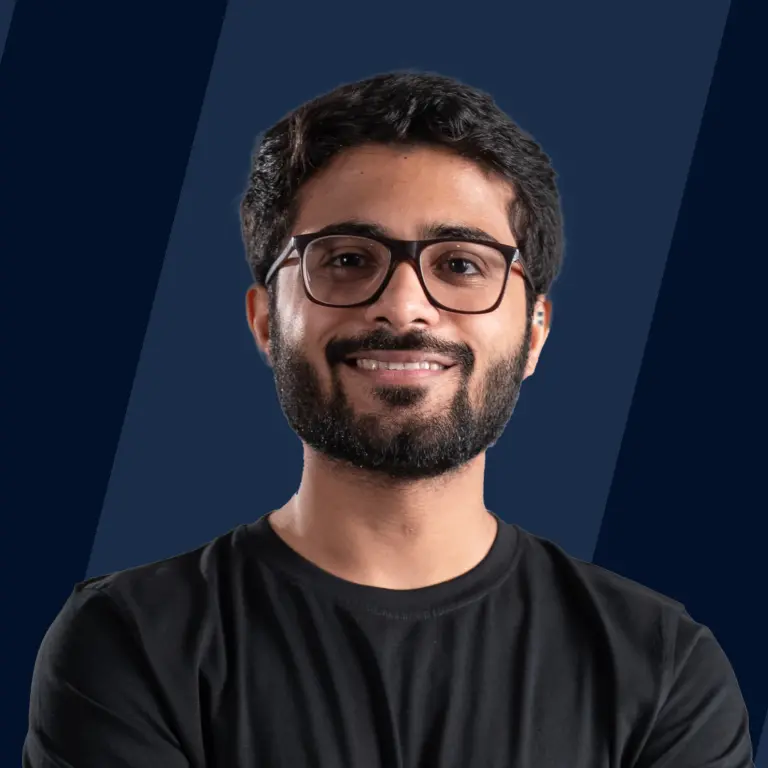
Overview
To reverse a number in Java means the digit at the unit place should be swapped with the digit at the highest or first position and the second digit should be swapped with the second last and on until the middle digit is left or swapped.
Reversing a Number in Java
To reverse number in Java involves swapping of digit at the first with the digit at the last and, the second digit with the second last until we finish swapping the numbers in the middle.
Example:
Note: In the second example after swapping we get 0054 but leading zeros must not be considered and thus, this edge case must be resolved.
Algorithm
To reverse a number in Java digit by digit, we can have a new variable reverse_number which will store the reversed value and apply the below algorithm to the number.
- Iterate through each digit of the given number.
- Take the modulus of the number and store it in a variable mod.
- Multiply the reverse_number by 10 and add mod to the reverse_number.
- The digit at the unit's position is reserved so now we can divide the number by 10.
Methods to Reverse a Number in Java
There are three commonly used methods to reverse a number in Java.
- Using While Loop
- Using Recursion
- Using StringBuilder class
Using While Loop
Description
To write a reverse number program in java we can apply the algorithm explained above using a while loop until the number becomes zero to the reversed number. Below given is the implementation of the above algorithm using while loop.
Implementation
Output
Explanation
In the above code function reverse() takes an int value and returns an int value. It takes the modulo of 10 and stores it in variable rem. The variable reverse_number is multiplied by 10 and rem is added to it in each iteration until the number equals zero. Here, the case of leading zeros is already handled as we'll be adding 0 which won't change the output and we get the reverse number in Java.
Complexity
Time complexity depends on the number of digits in a number. Space complexity is constant as we are not utilizing any external space.
Time complexity
Space Complexity
where D is the number of digits in the user input number n
Using Recursion
Description
Instead of using iteration to find the reverse of a number in Java, we can also make use of recursion. The code becomes simpler but the algorithm remains the same.
- The base condition is if the number becomes zero we terminate the recursion and we get the reverse number in Java.
- If the number is not zero then we take the digit at the unit's place using modulo.
- Multiply reverse_number with 10 and add modulo.
- Finally divide the number by 10 and call the function for the new number formed.
Implementation
Output
Explanation
We are using the global variable rev to store the reverse number in Java. The recursive function stores the reverse number in the rev variable. It uses the same algorithm used above to calculate the reverse number. Again the edge case of leading zeros is handled as we are using the addition of 0 which will not affect the output. Complexity
Time Complexity
where D equals the number of digits in the given number n.
Space Complexity
Space complexity to reverse a number in Java increases as we are using auxiliary stack space for storing local variables of the recursive calls for the function.
Using StringBuilder Class in Java
Description
All the above approaches on how to reverse a number in Java require a lot of calculation, logic, and time and space for reversing the number. We can do it lazily and Java provides inbuilt methods to do so in 2 simple lines of code. This is done using StringBuilder Class and its reverse() method. This makes the code self-explanatory and improves readability.
Implementation
Output
Explanation
The StringBuilder converts the Integer to a string in order to reverse a number in Java. This string is stored as a StrigBuilder type. The StringBuilder class has two functions that can help reverse a number in Java. The first one is reverse() which reverses the string. The toString() function converts the StringBuilder back to a string and we use Integer.parseInt() to convert this string back to the integer.
We are using a while loop to remove leading zeros. Unlike the above algorithms, this case is not handled and requires explicit implementation.
Complexity
- Time complexity
- Space Complexity
As we are using an object of the String Builder class it occupies size proportional to the number of digits. The reverse() function also takes time equal to the length of the string which is proportional to the number of digits in our case.
FAQs
Q: What are the common methods to reverse a number in Java?
A: There are three common methods to reverse a number in Java:
- Using a while loop to extract digits and build the reversed number.
- Using recursion to reverse the digits of the number.
- Converting the number to a string, using a StringBuilder to reverse the string, and then converting it back to an integer.
Q: Can negative numbers be reversed using these methods?
A: Yes, all three methods (while loop, recursion, and StringBuilder) can be used to reverse both positive and negative numbers. The minus sign remains at the beginning after reversal.
Q: Are there any limitations to these methods in terms of the size of the input number?
A: The size of the input number is usually limited by the available memory and data type limits. For very large numbers, you might need to consider using libraries that handle arbitrary precision arithmetic.
Conclusion
- The reverse number program in Java involves swapping of most significant digit with the least significant digits until we reach the midpoint.
- There are multiple ways to implement reverse number in Java algorithms. We can make use of the iteration, recursion, and StringBuilder class.
- The while loop method involves repeatedly dividing the number by 10 to extract the last digit which is added to the reversed number after multiplying the current reversed number by 10 This process continues until the number becomes zero.
- The same logic is used in the recursive method, the only difference is we are storing the reversed number as a global variable.
- The StringBuilder method involves converting the number to a string and then to a StringBuilder object. The reverse() method of the StringBuilder reverses the characters in the string. The reversed string is then converted back to an integer using toStirng() and Integer.parseInt().