Reverse String in JavaScript
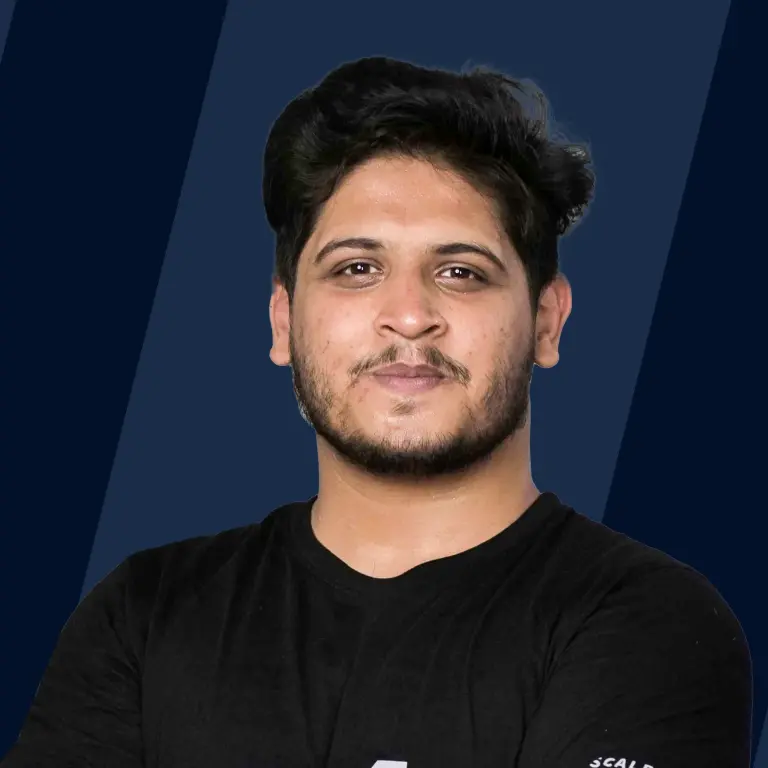
Overview
A string is a collection of alphabets, words, or other characters. Reversing a string is the technique in which all the characters are reversed, i.e., the first character becomes the last character and vice-versa. One of the uses of reversing a string is to find out whether the string is a palindrome or not.
Introduction
When we reverse a string in javascript, the first character of the string becomes the last character, the second character becomes the last but one character, and so on.
For example, if the string is "Hello!" then the reversed string is "!olleH". This can be done using built-in methods by converting the string into an array of characters, later reversing the elements in the array, and finally joining the elements of the array to form a string. We can also use for loops or recursion.
Methods to Reverse a String in JavaScript
A method is a function that is a property of an object in JavaScript. Methods contain a function definition. To reverse a string, we will make use of a few of the methods such as split(), reverse() and join().
Let us look at each of the methods one by one:
split()
The split() method in JavaScript splits a string into substrings. The syntax of the split() method is as follows :
The split() method takes two parameters, namely separator and limit. The separator is nothing but a string or regular expression to use for splitting.
Example:
Output:
In the above example, we can see how a string is converted into an array. As the parameter passed is "", every character in the string is split. The limit parameter is optional, and limit is an integer that limits the number of splits.
reverse()
The reverse() method reverses the sequence of elements in the array. The syntax of the reverse() method is as follows :
Example:
Output :
In the above example, oarray represents the old array, and narray represents the new array. We will use the reverse() method on oarray. We can see that in the output, the array elements are printed in reverse order.
join()
The join() method returns an array as a substring. The syntax of the join() method is as follows :
Example :
Output :
In the above example, we can see how a string is formed using the elements of the array.
Combining the Built-in Methods to Reverse a string in JavaScript
The simplest way to reverse string javascript is to split a string into an array, reverse() the array, and join() the array back into a string.
Let us look at an example to understand this better:
Code :
Output:
Explanation:
In the above code, we created a function named stringReverse which will reverse the string. In the stringRevese function, we will :
- First, convert the given string into an array that contains all the characters in the string as the array elements using the split() method.
- Next, we will use the reverse() method to reverse the order of elements in the array.
- At last, we will use the join() method to combine all the elements of the array into a string.
Using a for Loop to Reverse a string in JavaScript
Code:
Output:
Explanation:
In the above code:
- we will create an empty array.
- We know that strings are indexable. We will find out the length of the string using .length.
- We will iterate a decremented for loop running from the index of the last character in the string to the first element of the string.
- During each iteration through the loop, we will add the character from the string being iterated (input string) to the new empty string which we created.
- When the loop terminates, we will have a reversed string.
Using Recursion to Reverse a string in JavaScript
Recursion is a process in which a function calls itself until a condition is met. The substr() method returns the characters in a string beginning at the specified location, and The charAt() method returns the specified character from a string.
Code:
Output:
Explanation:
In the above code, initially, we will not have an empty string. Therefore, the else part will be executed.
We will try to analyze the program when "spoon" is the string to be reversed.
Iteration | Recursive call to reverseString(str) fuction | resulting str.substring(1) | resulting str.charAt(0) |
---|---|---|---|
1st Iteration | reverseString("spoon") | reverseString("poon") | "s" |
2nd Iteration | reverseString("poon") | reverseString("oon") | "p" |
3rd Iteration | reverseString("oon") | reverseString("on") | "o" |
4th Iteration | reverseString("on") | reverseString("n") | "o" |
5th Iteration | reverseString("n") | reverseString("") | "n" |
When the condition str becomes "", the recursion will travel back its depth, and the string is added to reverse(''), which results in the string and it continues until we get noops that states, the sting is reversed.
Reverse a string in JavaScript Using Conditional (Ternary) Operator
The syntax of ternary operation is as follows:
Here the exprIfTrue is executed if the condition is true; else, the expyIfFalse is executed.
Code :
Output :
Explanation :
The above code is similar to the code which we saw earlier but instead of if-else, we will make use of a ternary operation. Use this Free Online JavaScript Formatter to format your code.
Conclusion
- A string can be reversed using methods like split(), join(), reverse(), loops, or recursion.
- Using the split() method (Creates an array of substrings of the string), reverse() method (Reverses the order of the array), and join() method (Creating an array from the elements of the array) we can reverse a string.
- Decremented for loop can also be used to reverse the string
- A string can be reversed by making a recursive call to the function and using the .substr() and .charAt() methods.