Round in C++
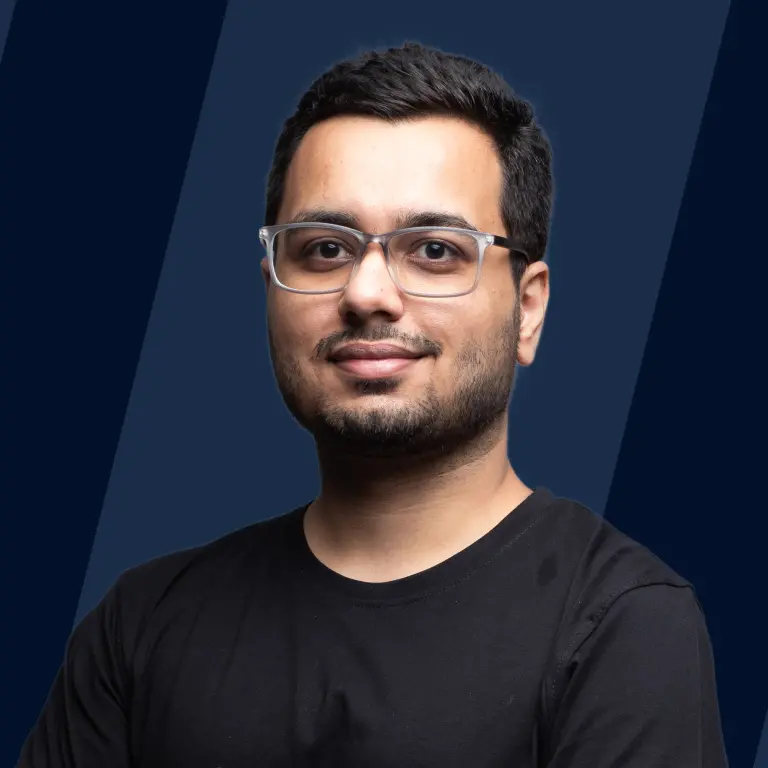
Overview
The round() function is declared in the <cmath> header file in C++. It is used to find the nearest Integer to the provided Real Number(technically floating point numbers).
Syntax of round() in C++
The syntax of isdigit() is given below,
Parameters of round() in C++
It accepts a floating point number of double data type.
Return Value of round() in C++
It returns the integral value of the double data type.
Note: As a beginner, you might get confused with the return type of this function. Please skip this section according to your knowledge. If the function is returning the nearest integer, then what is the significance of the double return type? And What does the term Integral value of double data type mean?
See this snippet,
Output:
Explanation:
- Although 99 is an integer its data type is double, d stands for double in C++. This is the integral value of the double data type.
- The function's return type is double because of its much greater range as compared to int. Although the return value is an integer we can't store the round of any double number in the int type.
Example of round() in C++
Output:
Explanation:
- We have passed 14.86 to the round function and the nearest integer to this function is 15 which is being returned according to our expectation.
- Similarly, 95 is the closest integer to 95.33.
What is round() in C++
<cmath> header consists of several functions for mathematical calculations and manipulations of numbers.
The round() function finds the nearest integer to the provided number,
There are a few other functions related to the round function which differ in the data type of parameter and return type. We can use this syntax according to the data type of our input number.
Syntax:
Comparison with Related Functions:
A few functions related to round() are shown below.
round() | ceil() | floor() | trunc() | |
---|---|---|---|---|
Description | Returns the nearest integer to the provided number | Returns the nearest integer greater than or equal to provided number | Returns nearest integer less than or equal to provided number | Returns the integer value by truncating the fractional part. |
Sample Input 1: 14.85 | 15 | 15 | 14 | 14 |
Sample Input 2: 18 | 18 | 18 | 18 | 18 |
Sample Input 3: -38.33 | -38 | -38 | -39 | 39 |
More Examples
Possible Applications
Handling the Mismatch between Fractions and Decimal
The decimal equivalent of some fractions contains many digits or even never-ending digits after the decimal point. But, generally, in calculations, we need only two, three, or four digits after the decimal point so, we can round up to find the decimal value of any fraction whose equivalent is too long. For Example, 1/3 is equivalent to 0.33.
Changing Multiplied Result
We can round up the extra digits of fractional number multiplication result, for example, if we multiply 0.86 and 0.32 The result will be 0.3132, so if we don't want extra decimal digits in the result we can do rounding.
Output:
Fast Calculation
With integral values, calculations are faster since floating-point numbers, namely signs, mantissa, and exponents, are internally stored in three different blocks. The computation of manipulating the mantissa and exponent is also complex, so rounding the result makes calculations faster.
Getting Estimate
Rounded Figures provide an estimate by avoiding a lengthy process.
Output:
Overloaded Versions of Round in C++
Additionally, there are a few overloads of the round function that differ in the parameter and return types.
Float Round( float F )
This function accepts a float type and also returns the float type after rounding the number to an integer value.
Output
Explanation
- f denotes the float.
Long Double Round( long double LD )
Similar to the last one, this function takes a long double and returns the long double value.
Output
Explanation
- e denotes the long double.
Double Round ( T var )
This is an extra overload of round functions in which we can pass any integral type i.e., int, unsigned int, short int, long int, etc.
Output:
C++ Code to Demonstrate the Use of Round in C++
Input:
Output:
Conclusion
- Round function is used to round the floating point numbers to the nearest integer.
- It returns always an integer value.
- It is declared in <cmath> header file.
- Rounding the number has several use cases, it provides an estimation of fractional expression, calculations becoming faster, etc.
- This function has several overloads and related functions, which we can use according to our requirements.
See Also
- ceil()
- floor()
- trunc()
- nearbyint()