Runnable Interface in Java
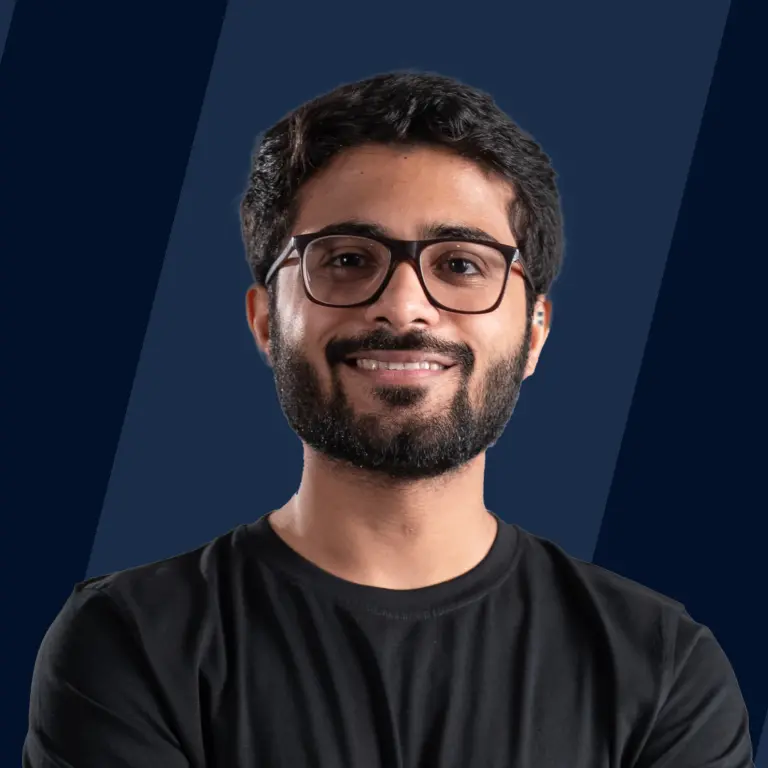
Overview
Runnable interface in Java is used to make a class instance run as a thread by implementing it. It is mainly used in multi-threaded programming where we want to execute the same class instance using multiple threads. The Runnable interface is provided by the java.lang package.
What is Runnable Interface in Java?
Consider that we have a class that process the given data and produce some result. If the incoming data is huge in volume, we can split the data into several chunks and process it parallely using the class. This can be achieved by making the class implement the Runnable interface to execute its instances in different threads. The Runnable interface has a single method, run(), that will be executed by Java when the thread is started. The run() method should be overridden by the class that implements the Runnable interface. The skeleton of the Runnable interface is:
run() Method of the Runnable Interface
The Runnable interface comes with a single method, run(). The run() method will be executed by Java when a thread is started with the class instance that implements the Runnable interface. The run() method is treated as the starting point of the class being executed by a thread.
Steps to Create a New Thread Using Runnable Interface in Java
We can create a new thread using the Runnable interface by following the steps below:
- Create a class that implements the Runnable interface.
- Override the run() method of the Runnable interface in the class.
- Create a Thread object in the main class by passing the class object that implemented the Runnable interface.
- Call the start() method of the Thread object.
Implementation of Runnable Interface in Java
Consider we have a class MessageScheduler that prints the given message after the specified delay in seconds. We can schedule any number of messages using the MessageScheduler, and each message should be scheduled in a different thread. We can achieve this using the Runnable interface as specified below:
MessageScheduler.java
The MessageScheduler class accepts message and delay as constructor parameters and implements the Runnable interface. The run() method is overridden to print the given message after the given delay.
Main.java
In the main() method, we schedule five messages with a random delay of between 1 and 3 seconds.
Output
Explanation
We pass the message and delay while instantiating the MessageScheduler instance. The run() method will be executed whenever the thread.start() is executed. The messages will be printed in a different order for each program execution because the delays are generated randomly.
Exceptions Encountered When Implementing the Runnable Interface in Java
The overridden run() method cannot throw any checked exceptions (exceptions caught at compile time. Eg: Exception). It can throw only unchecked exceptions (exceptions occurring at runtime. Eg: RuntimeException). The run() method can only throw RuntimeException and all exceptions that extends the RuntimeException.
Checked Exception Inside run() Method
Consider the below example, where we throw Exception inside the run() method
Compiling the above class throws the below error because Exception is a checked exception, and the run() method cannot throw it.
Unchecked Exception Inside run() Method
The below code doesn't throw any compile-time errors because the run() method can throw unchecked exceptions.
Use Cases of the Runnable Interface in Java
- Runnable interface in Java can be used to serve requests parallelly using multiple threads in a web application.
- Runnable interface can be used to process a huge volume of data using multiple threads to reduce the processing time.
- Runnable interface can be used to download a big file faster by downloading multiple chunks parallelly using multiple threads.
Thread Class vs Runnable Interface
Thread | Runnable |
---|---|
Thread is a class in Java. | Runnable is an interface in Java. |
Thread class has several methods such as run(), start(), interrupt(), etc. | Runnable interface has a single method run(). |
A class that extends the Thread class cannot extend another class because Java doesn't support multiple inheritence. | A class that implements the Runnable interface can extend another class. |
Conclusion
- Runnable interface in Java is used to make a class instance run as a thread by implementing it.
- Runnable interface in Java is provided by the java.lang package.
- Runnable interface in Java has a single method run() that should be overridden by the class that implements the interface.
- The run() method of the Runnable interface in Java cannot throw checked exceptions. It can throw only unchecked exceptions.
- Runnable interface is used when we want to execute a logic using multiple threads in a multi-threaded environment.