JavaScript Scope
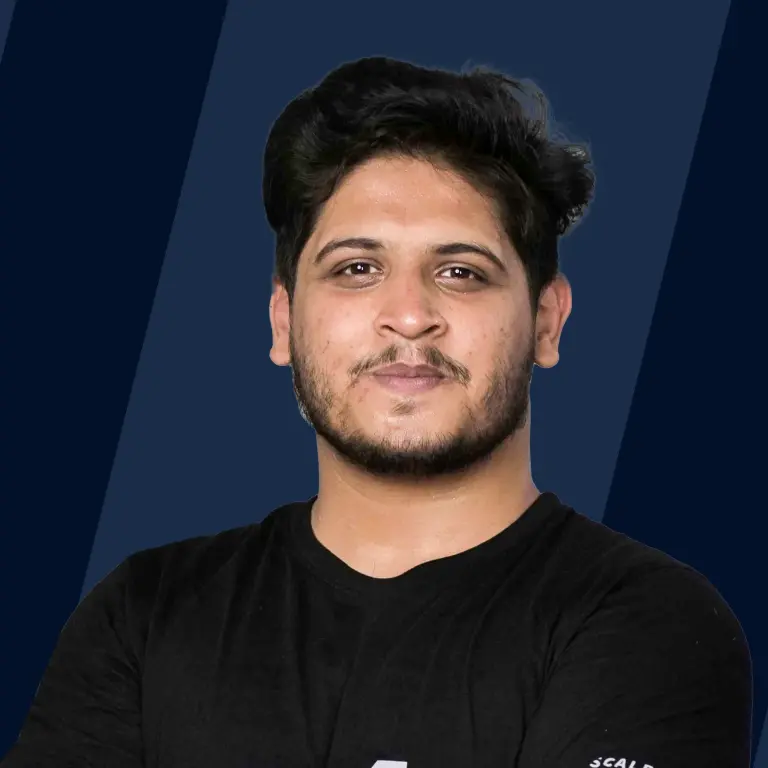
The concept of scope in JavaScript is crucial for controlling the availability of variables. The concept of global and local variables is defined by the scope, which is at the base of closures. The accessibility of variables, objects, and methods is defined by scope in Javascript.
JavaScript Variables Types of Scope
1. Block Scope
Block scope in javascript refers to the area within curly braces {}. Variables declared with let and const are confined to block scope, restricting their accessibility to the block where they're defined.
Example:
2. Function Scope
Function scope in javascript refers to the area within a function where variables are declared using var. Variables declared within a function are restricted to that function's scope and cannot be accessed elsewhere.
Example:
3. Module Scope
Module scope in javascript refers to the scope within a JavaScript module. Modules encapsulate code and provide a way to keep variables and functions private within the module unless explicitly exported.
Example:
4. Global Scope
Global scope in javascript refers to variables declared outside of any block or function. These variables are accessible from anywhere within the script.
Example:
5. Local Scope
Local scope in javascript refers to the scope within a function or block where variables are declared using let, const, or var. Variables declared within a local scope are only accessible within that scope.
Example:
Global JavaScript Variables
Global variables in JavaScript are variables declared outside of any function or block. These variables are accessible from anywhere within the script, including inside functions and blocks.
Example:
Automatically Global
In JavaScript, variables declared without the var, let, or const keywords inside a function or block are automatically assigned global scope. This can lead to unexpected behavior.
Example:
Strict Mode
Strict mode in JavaScript enforces stricter parsing and error handling rules to prevent common coding mistakes and silent failures. It's activated by adding "use strict"; at the beginning of a script or a function. This enhances code quality and facilitates debugging.
Example:
In this example, using x without declaration causes an error in strict mode, helping to catch potential bugs early.
Global Variables in HTML
In JavaScript, the global scope is typically associated with the environment in which the script runs. In HTML, the window object symbolizes the global scope within a browser environment.
Example:
In this example, globalVar1 is defined with var, attaching it as a property of the window object, while globalVar2 is declared with let, excluding it from the window object.
Warning
Creating global variables should be approached with caution to avoid unintended conflicts or overwrites with existing variables or functions in the global scope, such as those belonging to the window object. It's crucial to be mindful of potential naming collisions when defining global variables or functions, as any function, including the window object, can overwrite them.
The Lifetime of JavaScript Variables
- Global Variables: Persist throughout program execution, created at script start, and exist until completion.
- Local Variables: Exist within function or block scope, created upon execution, and removed when execution completes.
- Function Arguments: Behave like local variables, existing within the function scope and removed after function execution.
- Dynamic Variables: Created and destroyed based on scope, declared with var, let, or const.
- Global Object Variables: Declared without keyword, becoming properties of global object (e.g., window), existing until program termination, potentially leading to memory leaks.
Conclusion
- We have seen what is scope in JavaScript. It determines the visibility and accessibility of variables, objects, and methods.
- Scope of variables in javascript includes block scope, function scope, module scope, and global scope.
- Global variables are accessible from anywhere within the script and persist throughout the entire program execution.
- Local variables exist only within the scope of the function or block where they are defined and are removed from memory when the scope ends.
- JavaScript's strict mode enforces stricter parsing and error handling rules, enhancing code quality and debugging.