Second Largest Number in Python
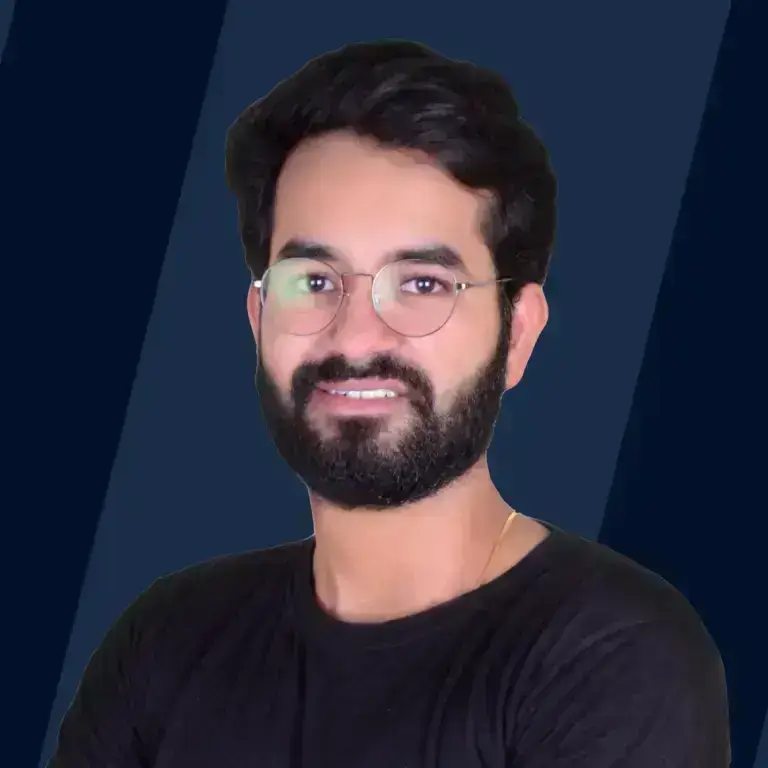
Overview
When we have multiple elements in a list, we may think of finding the largest or the smallest number in the list. Lists are ordered set of elements enclosed in square brackets ‘[]’ used to store multiple elements, where elements can be accessed with their indices. This article will discuss some algorithms to find the second-largest number in Python.
How to Find the Second Largest Number in Python?
There are many approaches to finding the second-largest number in Python. Some of the approaches are mentioned below:
- By sorting the list and then printing the second largest element.
- By removing the maximum element.
- By traversing the entire list.
- By using a max heap.
Now we will discuss all the methods one by one.
Method 1: By Sorting the List and Then Printing the Second Largest Element
This method is the easiest way to find the second-largest number in the list. It is so because we need to sort the list and then print the element on the second last index.
Syntax
Parameters
There is only one parameter i.e. the list itself.
Example
Output
Explanation
First, we will declare a list named ‘lst’, then apply a for loop and take the input of elements. At the time of the loop, we will append the input to the list every time we take input. Then we will pass the list to a function.
In the function, we will sort the array, and then we will print the element on the second last index i.e., using negative indexing.
Method 2: By Removing the Maximum Element
In this method, we will remove the max element from the list. Then we will again take the max element and print it.
Syntax
Parameters
Again the list is the only parameter required.
Example
Output
Explanation
Now let us see the explanation of the above code.
Like method 1 we will declare a list, filled with inputs taken by the user, and pass it to a function named ‘method 2’ as a parameter.
Inside method 2 we will use the max function to get the max element from the list, and then we will store it to a variable and remove it from the list using the ‘list.remove’ function.
Now that the max element is removed, we will print the max element, which is the second last element in the list.
Method 3: By Traversing the Entire List
In this method, we will traverse the entire list and maintain two variables to find the second largest element.
Syntax
Parameters
Same parameters as used in above methods.
Example
Output
Explanation
Let us see the explanation for the 3rd method.
Declare a list and fill it with elements using for loop. Declare a variable named 'L_Val' for storing the largest element and initialize it with an element on the first index. Declare another variable named SL_Val for storing the second largest element and repeat the process.
Traverse each element and compare it and find the largest element and update it to variable 'L_Val'. Repeat this, and this time compare the 'SL_Val' variable with 'L_Val' and find the second largest variable.
Finally print the variable 'SL_Val'.
Method 4: By Using a Max Heap
In this method, we will build a max heap from the elements of the list and will pop the root exactly one time. After that, we will print the new root which is the second largest element in the list.
Syntax
Parameters
List is only the required parameter in the function.
Example
Output
Explanation
In the above code, we first need to import 'heapq' then we will implement maxheap and build it from the elements of the list.
Then we will pop the root '1' time, store the maxheap's top value in a variable, and return it.
Then finally, we will call the function 'Method4', store its return value in a variable, and print it.
Some More Examples
Code
Output
Code
Output
Code
Output
Code
Output
Conclusion
- Lists are an ordered set of elements enclosed in square brackets ‘[]’ used to store multiple elements, where elements can be accessed with their indices.
- There are many approaches to finding the second largest number in Python. Some of the approaches are: Sorting the list and then printing the second largest element, removing the maximum element, and traversing the entire list, by using a max heap.
- In method 1, we just need to sort the list and then print the element on the second last index.
- In method 2, we will take the max element from the list and remove it. Then we will again take the max element and print it.
- In method 3, we will traverse the entire list and will maintain two variables to find the second largest element in the list.
- In method 4, we will build a max heap from the elements of the list and will pop the root exactly one time. After that, we will print the new root which is the second largest element in the list.