Segmentation Fault in C/C++
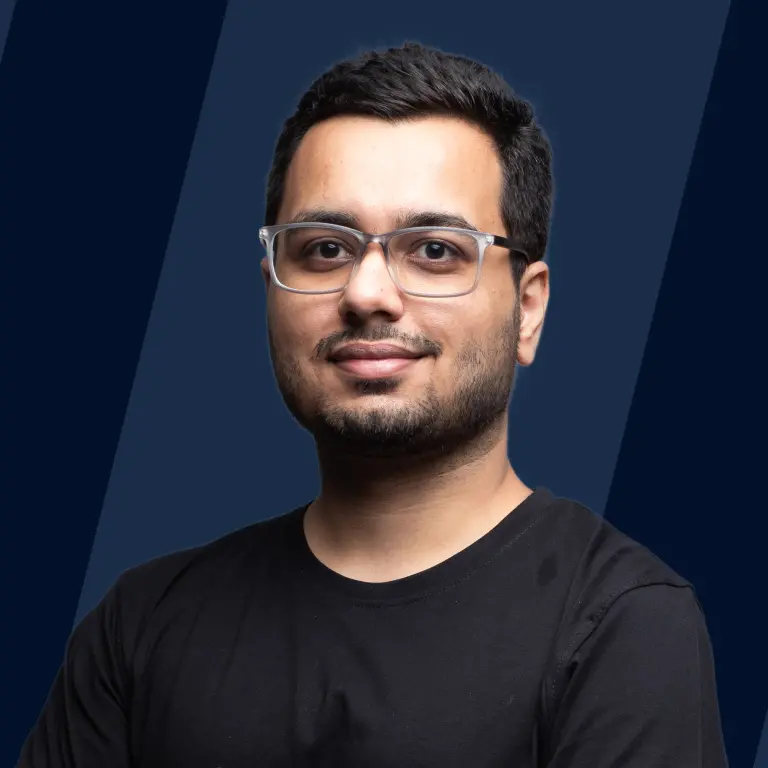
Overview
Segmentation Fault, sometimes known as segfault, is a common error in C and C++ programming. Consider your program a neighborhood, with each memory segment representing a house. A segmentation fault occurs when a program attempts to access a memory location that it is not permitted to access, resulting in a crash. This might be caused by dereferencing a null pointer or accessing memory outside the limits of an array. Your program must respect memory boundaries to keep these unwanted guests out.
Common Causes of Segmentation Fault in C/C++
Segmentation faults, often known as segfaults, are feared failures in C/C++ programming that may leave even the most experienced coders scratching their heads. These errors arise when a program attempts to access a memory region it is not permitted to access, resulting in an abrupt termination. In this section, we'll solve the enigma of segmentation errors by delving into their core causes in general terms.
-
Dereferencing a Null Pointer:
Dereferencing a null pointer is among the most prevalent causes of segmentation problems. A null pointer points to nothing and holds memory addresses. When you try to access memory referred to by a null pointer, the system throws a segfault, prohibiting you from accessing invalid memory.
-
Buffer Overflow:
Buffer overflows occur when a program puts more data into a buffer than it can hold. This can overwrite neighboring memory regions, including critical control data, resulting in unexpected behavior and, eventually, a segmentation failure.
-
Stack Overflow:
A stack is used by every program to keep track of function calls and local variables. A stack overflow occurs when a function call or variable declaration utilizes more stack space than is available. As a result of the program's foray into prohibited memory areas, a segmentation fault occurs.
-
Accessing Out-of-Bounds Memory:
In C/C++, arrays and pointers lack built-in bounds checking. Accessing memory outside of an array's allotted space might cause a segfault. This is most commonly seen when iterating through an array and exceeds its size.
-
Read-Only Memory Access:
Attempting to change read-only memory (constants, string literals, etc.) results in a segmentation fault. This protection prevents unintentional alteration of data that should not be altered.
-
Uninitialized Pointers:
Using uninitialized pointers or addressing de-allocated memory might cause a segfault. Before accessing a pointer, it should always be allocated a proper memory address.
-
Mismatched Memory Management:
Combining various memory allocation and de-allocation routines (for example, malloc with free or new with delete) might result in memory corruption and, ultimately, a segmentation fault.
-
Shared Library Issues:
Segfaults can also be caused by inconsistencies or incompatibilities in shared library versions. If your program uses external libraries, ensure they are compatible to avoid unexpected crashes.
-
Hardware Violations:
Hardware-related problems such as incorrect instructions or memory protection methods occasionally cause segmentation failures. While uncommon, these cases necessitate further examination.
-
Recursion without Base Case:
Omitting a base case in a recursive function can result in indefinite recursion and eventually stack Overflow, resulting in a segfault.
Common Segmentation Fault Scenarios
Let's look at some of the most typical segmentation fault scenarios developers experience to help us solve the problem.
Null Pointer Dereferencing
Attempting to access memory through a null pointer is a common problem. A segmentation fault occurs when a program attempts to read or write to an address with no value. This is frequently caused by failing to correctly initialize pointers before usage or accessing objects that have been destroyed or never created.
- Example: Dereferencing a null pointer, such as *ptr, is trying to access an address labeled nowhere, causing a runtime error or crash due to the absence of valid data at that location.
Array Index Out of Bounds
Arrays provide organized data storage, but accessing items beyond their assigned boundaries can be disastrous. Segmentation faults frequently occur when the program attempts to read or write to memory locations outside the array range. Vigilance in array indexing is crucial to prevent these errors.
Stack Overflow
The stack, a memory area utilized for function calls and local variables, has a finite space. A stack overflow might occur due to erroneous recursion or excessive local variable allocations. When the capacity of the stack is exceeded, the program receives a segmentation fault.
-
Example:
Buffer overflows occur when data written to a buffer exceeds its allocated size, encroaching into adjacent memory regions. These regions might hold critical data or code. When overwritten, data corruption occurs, leading to unintended program behavior, crashes, or even security vulnerabilities due to the execution of malicious code injected into adjacent memory areas.
Uninitialized Pointers
Using pointers that haven't been assigned a valid memory address can lead to unpredictable behavior, including segmentation faults. Avoiding such circumstances is made easier by properly initializing pointers before using them.
Read-Only Memory Access
To avoid unintentional change, modern operating systems frequently label particular portions of memory as read-only. A segmentation error occurs when a program attempts to write to a read-only memory area.
Shared Libraries and Dependencies
Memory access conflicts caused by mismatched or incompatible versions of shared libraries or dependencies can result in segmentation faults. Such problems may be avoided by using the correct library versions and dependencies.
Memory Leaks
Memory leaks can gradually deplete memory, resulting in segmentation errors. Unreleased memory fragments might cause the program to become unstable and crash.
Buffer Overflow
Buffer overflows occur when data written to a buffer surpasses its allocated size, spilling into adjacent memory regions. This can corrupt nearby data or overwrite critical control structures, potentially leading to crashes or security vulnerabilities. Exploiting this vulnerability, malicious actors can inject malicious code and gain unauthorized access or control over a program or system.
How to Fix Segmentation Faults?
While segmentation errors might be irritating, understanding their origins and adopting methodical debugging approaches can help you handle them efficiently.
Check for Uninitialized Pointers and Variables
Uninitialized pointers or variables are frequently the source of segmentation errors. Before attempting to access memory through pointers, ensure they are all correctly initialized. It's also critical to initialize variables to avoid problems.
Memory Allocation and De-allocation
Memory allocation and de-allocation errors can result in segmentation problems. Ensure that memory is adequately allocated using functions such as malloc or new and de-allocated using free or delete when no longer required. Using de-allocated memory might lead to unpredictable behavior and segmentation errors.
Array Bounds and Buffer Overflow
Accessing arrays outside of their limits can result in segmentation problems. Always check that array indices are in the correct range. Avoid buffer overflows, which can cause memory corruption and segmentation problems. To avoid these problems, use functions like strcpy and memcpy with caution.
Null Pointers and Dangling Pointers
Attempting to access or alter memory using null or dangling pointers might result in segmentation faults. Check for null pointers regularly and guarantee that pointers do not point to de-allocated memory.
Stack and Heap Confusion
Segmentation faults can also be caused by improper stack and heap memory management. Returning pointers to stack-allocated memory from a function should be avoided since this memory is invalidated when the function finishes. Heap memory, on the other hand, persists until explicitly de-allocated.
Debugging Tools
When a segmentation failure occurs, use debugging tools such as gdb (GNU Debugger) to examine the status of your program. These tools give useful information about the call stack, variable values, and memory contents at the moment of the issue, which aids in determining the root cause.
Debugging tools like gdb play a crucial role in diagnosing issues by allowing programmers to inspect memory contents, track program execution, and analyze variables. They provide a deeper understanding of program state, aiding in identifying errors and optimizing code for more efficient and reliable software development.
Comment Out Sections
If you need to figure out which portion of your code generates the segmentation error, consider gradually commenting out bits of code and reintroducing them. This elimination method can aid in identifying the troublesome code fragment.
Print Debug Statements
Strategically placed print statements can aid in locating the source of the problem. Outputting variable values and markers throughout your code might lead you to a failure point.
It offers crucial insights into program execution by displaying variable values and execution paths. These statements serve as valuable tools for identifying errors, tracking program flow, and validating code assumptions during the debugging process.
Examples of Common Segmentation Faults
Let's dive into a few real-world scenarios with code-based examples.
1. Null Pointer Dereference
In this except, the pointer ptr does not point to a valid memory address, but we are attempting to assign a value. This causes an immediate segmentation fault.
2. Buffer Overflow
The strcpy function writes more characters to the buffer than it can handle, resulting in a memory overflow. A segmentation fault may result from this overwrite of contiguous memory.
3. Uninitialized Pointer
Operating on an uninitialized pointer, such as uninitializedPtr, produces unpredictable behavior, frequently resulting in segmentation failures when the program attempts to access memory without appropriate initialization.
4. Out-of-Bounds Array Access
It is accessing an index outside the array's limits, as seen with arr[5], which attempts to read memory not allocated for the array. This results in a segmentation fault.
5. Writing to Read-Only Memory
In this instance, we are attempting to alter a string literal stored in read-only memory, such as roStr, which results in a segmentation fault owing to the read-only memory protection mechanism.
Conclusion
- Segmentation faults are mostly caused by a program attempting to access a memory region outside its permitted bounds. This can occur while working with pointers or arrays.
- Dereferencing a null pointer will always result in a segmentation fault. Before using pointers, make sure they are correctly initialized.
- Understanding the difference between stack and heap memory is critical. Stack overflow, frequently caused by excessive recursion, can result in segmentation failures.
- Using variables without first initializing them might result in unexpected behavior and segmentation errors. Make it a practice to initialize variables as they are declared.
- Overwriting contiguous memory with more data than a buffer might cause segmentation faults. When working with strings and arrays, use caution.
- Buffer overflows occur when data written to a buffer surpasses its allocated size, spilling into adjacent memory regions.
- Memory leaks can gradually deplete memory, resulting in segmentation errors.