Send Email using Python
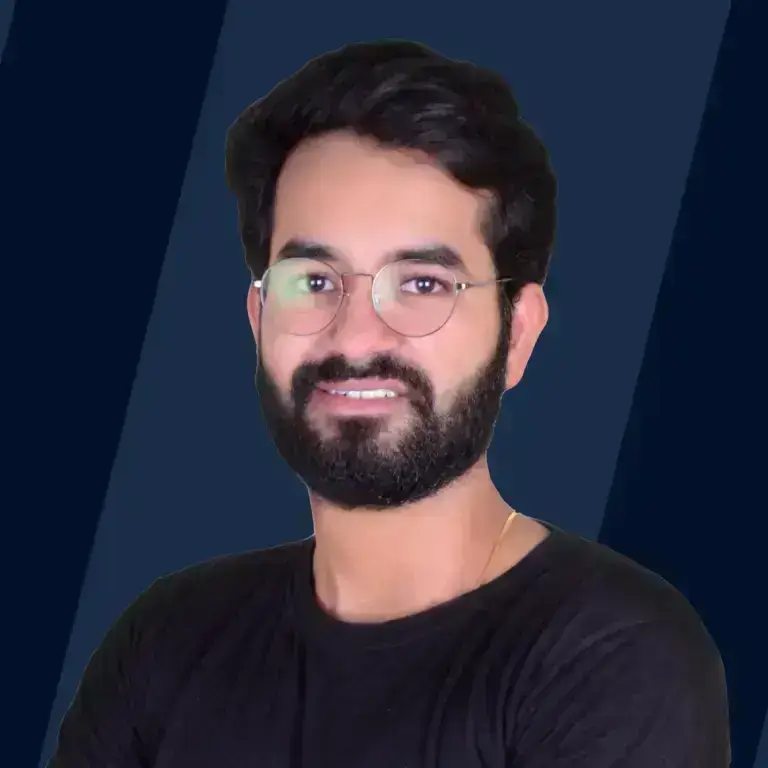
Sending emails is one of the essential tasks in today's corporate world. Therefore, in Python, to send and receive mail, we use a protocol known as SMTP (Simple Mail Transfer Protocol). In this article, we will be learning how to send email using python.
How can you send Emails using Python?
Step 1: Import Necessary Modules
Start by importing the necessary modules from Python's standard library:
- smtplib is used to set up the SMTP server connection.
- email.mime.text and email.mime.multipart is used for creating the email message with both text and potentially other parts (like attachments).
Step 2: Set Email Credentials and Recipient
Define the sender's email address, the recipient's email address, and the SMTP server details:
Replace your_email@example.com, receiver@example.com, and smtp.example.com with your actual email address, the recipient's email address, and your email provider's SMTP server address, respectively.
Step 3: Create the Email Message
Create a MIMEMultipart object to represent the email and fill in the basic details:
Step 4: Add the Email Body
Create a MIMEText object to represent the email body and attach it to your message:
Step 5: Connect to the SMTP Server
Now, it will establishing a secure connection to the SMTP server:
If your server uses STARTTLS, you might need to use smtplib.SMTP() and then enable STARTTLS with server.starttls().
Step 6: Send the Email and Close the Connection
Send the email and then close the connection to the SMTP server:
Step 7: Test Your Script
Run your script. If everything is set up correctly, the recipient should receive the email you've sent. Ensure you've entered the correct SMTP server details and that the email credentials are accurate.
Important Notes
- Security: Do not hard-code your password in the script for production use. Consider using environment variables or secure vaults.
- Email Provider Settings: Some email providers require you to enable settings to allow access via less secure apps or to set up an app-specific password.
- Troubleshooting: If the email is not sent, check your email provider's documentation for SMTP settings and ensure your script matches those settings. Following these steps should help you successfully send an email using Python. Remember to adhere to best practices for handling sensitive information like passwords.
Sending Email to Multiple Recipients using Python
Let's learn how to send email using python for multiple recipients:
-
Import Libraries: Ensure you have the necessary libraries imported in your Python script. This script already includes the required imports for sending emails.
-
Define Function Parameters: Replace the placeholder values in the function send_email() with your actual email details:
- sender_email: Your email address from which you want to send the email.
- recipients: A list of email addresses of the recipients.
- subject: The subject of the email.
- body: It contains the normal text in the email.
- smtp_server: The SMTP server address.
- smtp_port: The SMTP server port.
- smtp_username: Your SMTP username (if required by the SMTP server).
- smtp_password: Your SMTP password (if required by the SMTP server).
-
Example Usage: In the example usage section:
- Replace 'sender@example.com' with your actual sender email address.
- Replace ['recipient1@example.com', 'recipient2@example.com'] with a list of recipient email addresses.
- Modify the subject and body variables with your desired email subject and content.
- Replace 'smtp.mailtrap.io', 2525, 'your_username', and 'your_password' with your SMTP server details.
-
Run the Script: Save the script with the modifications and run it using Python. You should see a message indicating whether the email was sent successfully or if there was an error.
Example of send email using python:
This Python script allows us to send an email to multiple recipients simultaneously using the SMTP protocol. Simply provide the sender's email address, a list of recipients, the email subject, body content, and SMTP server credentials. The script handles the process of connecting to the SMTP server and sending the email efficiently.
Sending Fancy Emails: emails with HTML content and attachments
With HTML Content
Here's a step-by-step guide with code examples and descriptions for sending an email with HTML content using Python's smtplib and email.mime modules:
-
Import Modules:
Description: Import the necessary modules. smtplib is used for connecting to the SMTP server, while MIMEMultipart and MIMEText from email.mime are used for creating the email message.
-
Set Credentials:
Description: Define the sender's email address and password. Also, specify the recipient's email address to whom you want to send the email.
-
Compose Message:
Description: Create a MIMEMultipart object to represent the email message. Set the subject, sender, and recipient addresses in the message headers.
-
Craft HTML Content:
Description: Define the HTML content of the email. You can include formatted text, links, and other HTML elements as needed.
-
Attach HTML Content:
Description: Create a MIMEText object with the HTML content and attach it to the email message. Set the content type to "html".
-
Connect to SMTP Server:
Description: Establish a secure connection to the SMTP server. Use "smtp.gmail.com" as the server address and 465 as the port number for SSL/TLS encryption.
-
Login:
Description: Authenticate with the SMTP server by logging in using the sender's email address and password.
-
Send Email:
Description: Send the email by using the sendmail() method of the SMTP server. Provide the sender's email address, recipient's email address, and the email message converted to a string using message.as_string().
-
Close Connection: The connection to the SMTP server is automatically closed when exiting the with block, so there's no need for explicit closing.
Following these steps will send an email with HTML content using Python. Make sure to replace placeholders with your actual email credentials and customize the HTML content as needed.
With Attachments Using the email Package
To send email in python with attachments using the email package in Python, you can follow these steps:
-
Import Necessary Modules: Import the required modules from the email.mime library:
-
Compose the Email: Create an email message using the MIMEMultipart class. Specify the sender, recipient, subject, and body of the email. Include both plain text and HTML content if needed:
-
Attach File: If you want to attach a file to the email, open the file in binary mode, encode it, and add it as an attachment:
Replace 'path/to/attachment/file.pdf' with the actual path to your attachment file.
-
Connect to SMTP Server: Connect to the SMTP server of your email provider and login:
-
Send the Email: Use the sendmail() method to send the email:
-
Close Connection: After sending the email, close the connection to the SMTP server:
That's it! You've successfully sent an email with attachments using the email package in Python. Make sure to replace 'sender@example.com', 'recipient@example.com', 'smtp.example.com', 587, 'your_email@example.com', and 'your_password' with your actual email addresses, SMTP server details, username, and password.
Yagmail
- Yagmail is a library that simplifies the process of send email using python in gmail.
- It abstracts the complexities of configuring SMTP settings.
- It provides a straightforward interface for sending emails.
- Below is an example of how to use Yagmail to send an email:
Make sure to install Yagmail before running the script:
Yagmail simplifies the process of sending email using Python and Gmail, making it an ideal choice for developers who want a hassle-free email solution. Whether you need to send plain text emails, HTML content, or attachments, Yagmail provides a convenient and reliable solution.
Transactional Email Services
- Transactional email services are platforms that help you in sending automated emails.
- These types of services are mainly used for commercial and business purposes.
- These emails are highly personalized and include messages like order confirmations, verifications, etc.
Here are a few popular transactional email services:
-
SendGrid: SendGrid offers a reliable cloud-based email delivery platform with features such as customizable templates, real-time analytics, and scalability to handle high volumes of emails.
-
Amazon SES (Simple Email Service): Amazon SES is a cost-effective email service provided by Amazon Web Services (AWS). It offers features like email sending, receiving, and monitoring and can scale based on demand.
-
Mailgun: Mailgun is an email automation service designed for developers. It provides APIs for sending, receiving, and tracking emails and features like email validation and analytics.
-
Postmark: Postmark focuses on transactional emails and boasts high deliverability rates. It offers detailed analytics, message tagging, and webhook support for tracking email events.
-
Brevo: Brevo is a transactional email service provider offering a free plan allowing users to send up to 300 emails per day.
-
Mailjet: Mailjet is a cloud-based email service provider that provides transactional and marketing email solutions. It provides features such as email automation, segmentation, A/B testing, and real-time analytics.
-
Sendinblue: Sendinblue is a comprehensive marketing and transactional email service provider. In addition to email, it offers SMS marketing, marketing automation, and CRM tools. It also provides an intuitive interface, advanced reporting, and deliverability optimization features.
-
SparkPost: SparkPost is an enterprise-grade email delivery platform trusted by leading companies worldwide. It offers features such as predictive email intelligence, real-time analytics, and advanced deliverability tools to ensure emails reach the inbox efficiently.
Conclusion
- We learned how to send email in Python using the smtplib module.
- We also learned how to establish a connection using the SMTP function, which returns an object that can be used to send email in Python using the send mail function.
- We are using the email.mime library to send email in Python with HTML content and attachments.
- Businesses can use Transactional Email Services to send personalized emails.