C++ iomanip Setprecision() Function
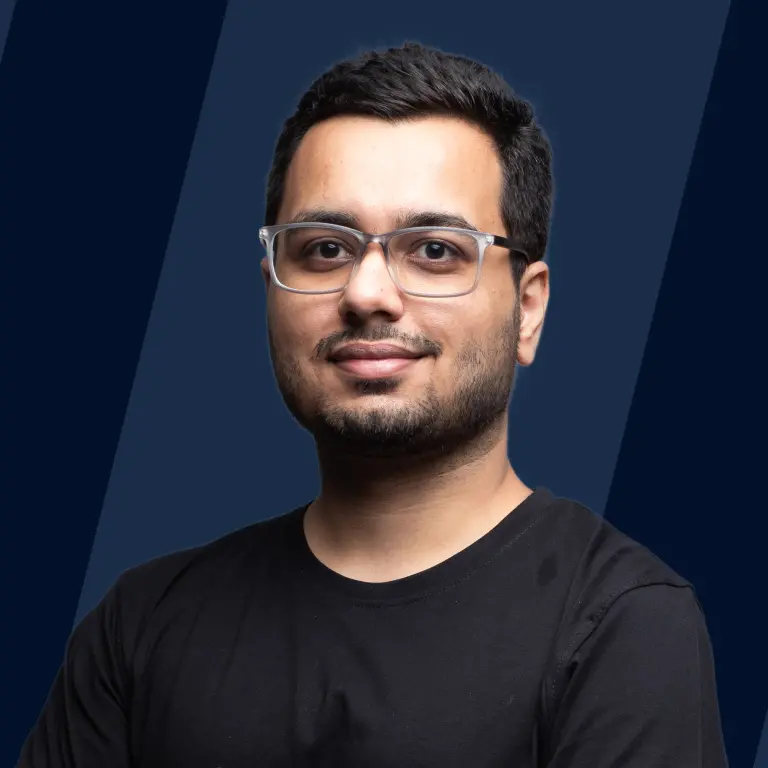
Overview
setprecision() method in C++ is an inbuilt method that is used to manipulate floating-point values. It is used to set the precision of the floating-point numbers after the decimal.
set precision in C++ is defined inside the <iomanip> header file.
Syntax of C++ iomanip setprecision() Function
Let's see how to write the setprecision() method inside our code:
Parameters of C++ iomanip setprecision() Function
The setprecision() function takes only one parameter i.e. n.
- n denotes the number which specifies the number of significant digits required in a floating point number. It is a necessary parameter. The parameter should be an integer.
Return Value of C++ iomanip setprecision() Function
The return value of setprecision() in C++ is unspecified. The manipulator method only acts as a stream manipulator.
Exceptions of C++ iomanip setprecision() function
The set precision in C++ function does not generally throw an exception. If any exception is thrown by the manipulator function i.e, setprecision then the output stream is in a valid state.
How Does C++ iomanip setprecision() Function Work?
The set precision in C++ manipulates the floating-point numbers by giving them a precise value after decimals up to n significant digits. It controls the number of digits after a decimal of a floating-point number in the output stream.
The set precision in C++ works to prevent information loss. For example, We store the value of pi, which contains an infinite number of digits. In 4-bit or 8-bit memory architecture, some digits after decimal get reduced automatically. So, with the help of the setprecision() function, we can obtain a precise value. For example, setprecision(3) will have 3 significant digits in the value of pi i.e, 3.14 without the loss of information.
For manipulating the decimal places that will be displayed in the output, use the fixed keyword before using setprecision(). The function will display the number of decimal places required in the output.
Examples
Now, Let's see the implementation of the setprecision() function with the help of some examples:
Example 1:
The following example is a simple implementation of set precision in C++ which will display the number of significant digits after decimal in a double type number.
Code:
Output:
Example Explanation:
In the above code, first, we initialized the number with a value equal to 3.14159, and then we printed the actual value of the number before setting up the precision. After that setprecision() method is used to set the precision up to different numbers of significant digits of the number. We have used setprecision(3) and setprecision(5) which are working fine and giving the desired output.
Example 2:
The following example is a simple explanation of the set precision in C++ in which we will display the default precision and maximum precision, and then we will use setprecision() till a significant digit.
Code:
Output:
Example Explanation:
In the above program, we have shown the value of pi in three different formats which are default precision, max precision, and setprecision(). The default precision prints the value till 6 significant digits and other digits are removed. The last format is maxed precision which is derived by using numeric_limits<double>::digits10, defined inside the <limits> header file. The other format is setprecision(8) which prints numbers to 8 significant digits.
Example 3:
The following program shows a simple implementation of the setprecision() function in which we will use fixed to set precision in decimal places.
Code:
Output:
Example Explanation:
In the above program, we have initialized a double variable 'number' which is equal is 3.141592. Then we set the precision to 6 and 9 significant digits and printed it. We have used a fixed keyword to print the default value of the number which displayed 6 digits after the decimal. So, when setprecision(9) is used, it will print 9 significant values with 0 as the remaining values.
Conclusion
- set precision in C++ is a manipulator used to set the precision of the floating-point number after the decimal in the output stream.
- setprecision() function is defined inside the
header file. - set precision in C++ works to prevent the loss of information.
- The setprecision() function takes one parameter i.e, the number of significant digits required in the output.
- For manipulating the decimal places which will be displayed in the output, use the fixed keyword before using set precision in C++.