Set in JavaScript
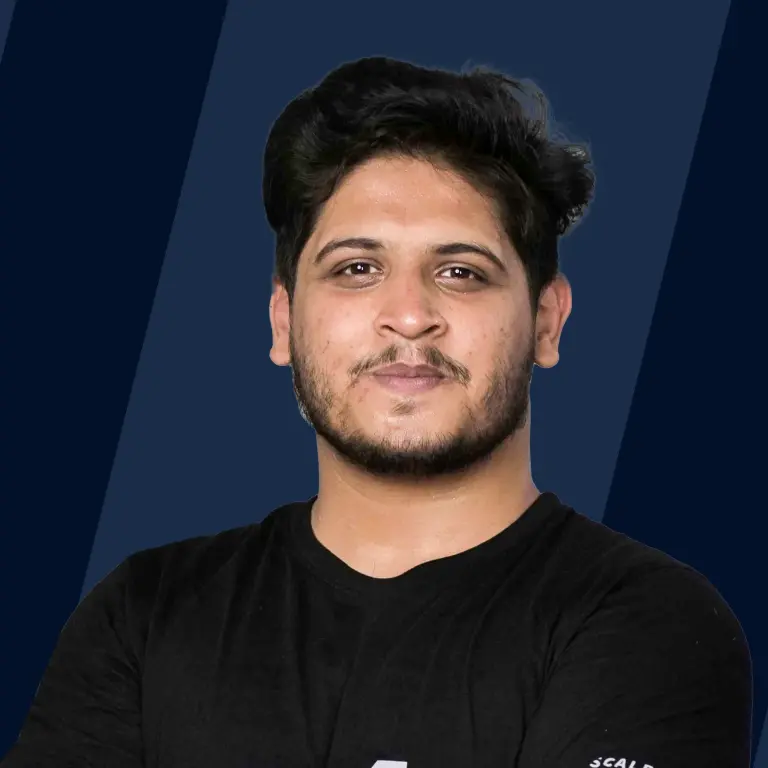
Overview
Javascript is an object-oriented language. As such it provides us with a lot of features in the form of objects which help in powerful data management. Set in Javascript is one such object used for the collection of data. Using a set allows us to store unique elements of any type.
What is Set in JavaScript?
Set in Javascript is a collection of unique elements. It can contain items of any data type. A set in javascript can be created by using the new Set() constructor and adding elements by the add() method, or by passing an array or string.
The set object is provided by ES6. It has a lot of built-in methods for managing data effectively. The set maintains the order of the elements during insertion. Thus we can iterate through a set in the same order in which elements were inserted.
Value Equality
To determine if two values are functionally identical in every possible context, we use Same-Value equality. Same-Value treats 0 and -0 as different. Value Equality is used to use SameValue algorithm.
Performance
The has method in the set is used to check if an element exists in the set. Considering an array of length equal to the number of elements in the set, the has method is faster than Array.prototype.includes(), which is used to determine whether an array contains a particular element.
Constructor
The Set() constructor is used to create new set objects in javascript.
The syntax is as follows:
Optionally we can pass an iterable object to a set constructor in the following way.
This is used to add all elements of the iterable directly to the set.
Static properties
get Set[@@species]
This constructor function allows us to create derived objects in javascript. The species property is used to return the default constructor function. As the default constructor function is a Set constructor for Set objects, species will be returning the default constructor for Set objects.
Set Property
Set in javascript contains the size property which helps us to see the size of the set. It is summarized in the table below:
Property | Description |
---|---|
size | Returns the size of the set, i.e the number of elements in the set. |
Set Methods
There are a lot of in-built methods for set in javascript that make life easier for the developer. Let us see those in the table below:
Method | Description |
---|---|
add() | Adds an element to the set |
delete() | Deletes an element from the set |
clear() | Deletes all elements from the set |
has() | Returns true if an element is present in the set |
forEach() | Invokes callback function for each item present in the set |
new Set() | Used to create a new set |
values() | Returns iterator with all the values present in the set |
entries() | Returns iterator containing an array of [value, value] for each element in the set. |
keys() | Same as values |
Set Operations
Some of the operations that we can perform using a set include subset, union, intersection, and difference. Their descriptions are provided in the table below:
Property | Description |
---|---|
subSet | Returns true if one set is the subset of the other |
union | Returns a set containing a union of two sets |
intersection | Returns a set containing the intersection of two sets |
difference | Returns a set containing the difference between two sets |
Examples
Using the Set Object
In this example, we shall see how to use the set object in javascript. We shall create a set using the new Set() method. Next, we shall see how to use the add(), has(), and delete() methods in set.
Explanation:
We create a new set called mySet. We then add various elements to the set using the add() method. The above example proves that a single set can contain elements of different data types since we have added both numerals and alphabets in our newly created set. We add the alphabets a,b,c,d and the number 1,2,5,9 in our set.
Next, we check if certain elements exist in our set using the has() method. It returns true when we search for the exact case-sensitive elements which we had added to our set, and returns false otherwise.
We then use the size() property of the set in javascript to get the size of the set. Initially, we had added 7 elements in our set, thus size() property returns 7. Next, we perform a deletion operation, and then while again checking the size of the set we get back 6.
Iterating Sets
Let us now see the different methods in which we can iterate through a set in javascript.
Method 1
In this method, we shall see how to iterate the above set using for method.
Output:
The above method prints the elements of the set in the order in which they were inserted.
Method 2
In this method, we shall see how to iterate the above set using the keys of the set.
Output:
The above method prints the elements of the set in the order in which they were inserted.
Method 3
In this method, we shall see how to iterate the above set using the values of the set.
Output:
The above method prints the elements of the set in the order in which they were inserted.
Method 4
In this method, we shall see how to iterate the above set using the key, and value pairs in the set.
Output:
The above method prints the elements of the set in the order in which they were inserted.
Method 5
In this method, we shall see how to iterate the above set using the forEach() method.
Output:
The above method prints the elements of the set in the order in which they were inserted.
Implementing Basic Set Operations
SuperSet A set is said to be a superset of another set if it contains all elements of the other set. Suppose we have two sets, setA, and setB. Here we are checking if setA is a superset of setB. Thus for every element of setB we are checking if it is present in setA. If the above condition is satisfied, setA is a superset of setB. Now we shall see how to implement the superset operation in set in javascript.
SubSet A set is said to be a subset of another set if the other set contains all elements of this set. Suppose we have two sets, setA, and setB. In this example, we shall see how to implement the subset operation in set in javascript.
Explanation:
In the above example, we are checking if setB is a subset of setA. Thus for every element of setB we are checking if it is present in setA. If the above condition is satisfied, setA is a superset of setB.
Union
A union of sets returns a common set containing all elements of both sets. Here is the implementation of the union operation in set in javascript. Suppose we have two sets, setA, and setB. We take these two sets ad add all their elements to a new set. The resultant set is the union of the two sets. We call that the unionSet. Let us see the code snippet for the union of two sets in Javascript.
Difference Suppose we have two sets A and B. The difference between these two sets A and B returns all the elements of set A that are not in set B. We add all elements of setA in the differenceSet. Then we delete the elements of setB from the differenceSet. Now we shall see how to implement the difference of sets in Javascript.
Relation with Array Objects
Conversion of a set to Array
In this example, we shall convert a set to an array.
Explanation: We create a set using the new Set() method. We then create an array from the above set using Array.from() method.
Conversion of Array to Set
In this example, we shall convert an array to a set.
Explanation:
We create an array containing certain elements. We then create a set from the above array using the new Set() method and pass the above array as a parameter to the method. We then use the has() method in set in javascript to assert if the set contains the same elements as contained in the original array.
Remove Duplicate Elements from the Array
In this example, we shall see how we can use a set to remove duplicate elements from an array.
Explanation: We created an array containing duplicate elements. We then created a set from that array. Thus all the duplicate elements from the array got stored only once in the set. In this way, we removed the duplicates from the array.
Relation with Strings
This example shows how to create a set from a string.
Explanation: We construct two strings and pass them as parameters while creating new sets. The resultant sets have the same size as the number of unique elements in the strings.
Use Set to Ensure the Uniqueness of a List of Values
The most common usage of a set is to ensure the uniqueness of a set of values. In this example, we are given an array, and we shall determine whether it contains unique elements or not, using a set.
Explanation:
At first, we create an array containing duplicate elements. We then create a set from the already created array. As a set contains unique elements only, the duplicated elements get inserted only once in the set. As such the size of the set is not the same as the size of the array. From this, we can conclude that the array does not contain unique elements.
We then create an array containing distinct elements. When converted to a set, no element is lost since all elements are unique. As such, the size of the set remains the same as the size of the array. From this, we can conclude that the given array contains unique elements.
Let us go through some examples to demonstrate the usage of commonly used set operations:
Example 1: SuperSet
In this example, we shall see how to implement the superset operation in set in javascript.
Explanation: In the above example, we are checking if setA is a superset of setB. Thus for every element of setB we are checking if it is present in setA. If the above condition is satisfied, setA is a superset of setB.
Example 2: SubSet
In this example, we shall see how to implement the subset operation in set in javascript.
Explanation: In the above example, we are checking if setB is a subset of setA. Thus for every element of setB we are checking if it is present in setA. If the above condition is satisfied, setA is a superset of setB.
Example 3 : Union
In this example, we shall see how to implement the union operation in set in javascript.
Explanation: In the above example, we take two sets ad add all their elements to a new set. The resultant set is the union of the two sets.
Example 4: Difference
In this example, we shall see how to implement the difference of sets in Javascript.
Explanation:
We add all elements of setA in the differenceSet. Then we delete the elements of setB from the differenceSet.
Browser Compatibility
Set in Javascript is compatible with all major browsers including:
- Chrome
- Edge
- Firefox
- Opera
- Safari
- Chrome Android
- Firefox for Android
- Opera for Android
- Safari on IOS
- Webview Android
- Samsung Internet
- Deno
Conclusion
- Set is an object in Javascript used to store unique elements.
- There are various built-in methods, properties, and operations in the set that makes life easier for the developer.
- Sets are mutable, i.e can be changed after creation.
- Sets can contain elements of any data type.