Simple Java Program
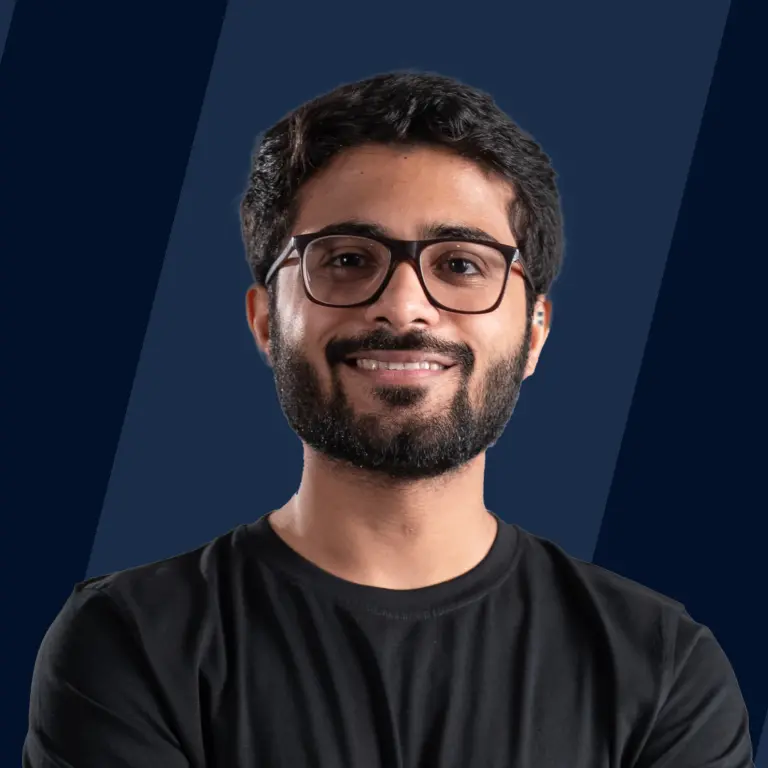
Overview
Java is a simple, object-oriented, robust, platform-independent, concurrent, and secured programming language for application development by James hosling and publicly released on May 23, 1995.
In this article, we will be learning about simple java programs and the fundamentals of java.
What is a Simple Java Program ?
To create a simple java program, follow the following steps :
- Create a file and copy the code given below in the file named “firstprog.java” (you can name the file anything, just make sure that public class name matches the file name).
- Compile it by using the keyword “javac” and the filename: javac firstprog.java.
- Execute the program by using the “java” keyword along with the filename: java firstprog.
Output :
This is a simple java program. Some observations in the java program are:
- If there is some syntactical error the compiler will help us to remove the error by displaying the appropriate message.
- Class and function should be given names else compilation fails.
- The function should have a return type else compilation fails.
- The main() method must return a value of type void for JVM to execute the program.
- If we don't write public or static or both we get the error: Error main() method not found in the class firstprog.
- The public and static keywords can be interchanged.
- String args[] can be written as String[] args/ String[] args/String args[]/ String …args, and it is used for accessing command line arguments.
- While compiling the program we should give a java extension but while running the program .java/ .class/ .main extension should not be used.
Parameters Used in the Java Program
Understanding basic code structure :
- Class definition:
to create a new class in java, the class keyword is used. - firstprog:
It is the name of a class also known as an identifier. - main() method:
The main() method is the point of start of execution and should be included in every java program. - public:
Methods declared as public can be accessed from anywhere. - static:
This is written so that the main() method can be called using the class name without the need of an object of firstprog class. - void:
The void return type means that the main() method does not return anything. - String[] args:
The main method accepts the command line arguments as an array of strings. - System.out.println() :
It is used for printing the text in the output window. System is a predefined class used for accessing the system, and out is the variable used for the output stream which is connected to the console. - Command Line Arguments:
It refers to the arguments which are sent while executing the Java compiled(.class) file.
Examples of Simple Java Programs
Calculator Program in Java
In this, we will be creating a simple calculator which will perform basic operations like addition, subtraction, division, and multiplication according to the user's choice.
Output :
Explanation :
In this, two double data type numbers are taken from the user along with the type of operator. Once this is done, a switch case is used for identifying the operator and performing the operation to be performed. If any other operator, other than (+,-,*,/) is used then the default statement of the switch case gets executed.
Factorial Program Using Recursion
In this example, we will learn how to find a factorial of a number using a recursive method.
Output :
Explanation :
To find the factorial of a number, we first have to check if the number is not equal to zero. If not, then we will use the formula (nfactorial(n-1)) to calculate the factorial recursively. And if it is 0 then since the factorial of 0 is 1 we return it.
Fibonacci Series Program
Output :
Explanation :
In this, 0 and 1 always remain constant and the loop starts from 2. For calculating the Fibonacci series, we first iterate over a loop and calculate the resultant number by adding two numbers before the current number.
For example, to calculate the 3rd number which is one, we calculate it by adding 0+1 which is equal to 1. This is how the Fibonacci series is calculated.
Palindrome Program in Java
A palindrome number is a number, which on reversing is the same number as that of the original number. For example, 545 on reversing is 545 (same) hence it is a palindrome number.
Output :
Explanation :
For checking, if the number entered by the user is palindrome or not, follow the steps given below:
- Take the number as input that is to be checked.
- Hold the input number in a temporary variable.
- Reverse the number.
- Compare the new number (temporary) with the original number.
- If both the numbers are the same then palindrome else not.
Permutation and Combination Program
- Permutation refers to the number of distinct ways of arranging r elements (selected out of n elements).
- Combination on the other hand, refers to the number of distinct ways of selecting r elements out of n elements.
Mathematical formula:
-
Permutation :
-
Combination:
Output :
Explanation :
To find the permutation and combination, we first have to find the factorial of the involved numbers. Once we define a function to compute the factorial of a number, for finding permutation use the formula, whereas for finding a combination of a number, use the formula .
Pattern Programs in Java
Pattern programs are for making some kind of patterns at the output. For instance, here are some basic patterns in java.
Pattern :
Code :
Explanation :
In this code, the first loop is for the row and the second loop is for the column. The colored box is the space. For each value of , the inner loop(with variable ) runs times from 0 to (inclusive). Thus,
- For i = 0, inner loop runs once.
- For i = 1, inner loop runs twice.
- For i = 2, inner loop runs thrice.
- For i = 3, inner loop runs four times.
And each time the inner loop runs, * is printed.
String Reverse Program in Java
Java program to reverse a string :
Output:
Explanation:
For reversing the string, we first have to take input for the original string. To reverse the string, initialize the new variable to empty (““). Loop over the input string character by character and add it to the new string (which is empty) at the front of it. This operation will basically reverse the input string. Once the loop is over, print the new string.
Mirror Inverse Program in Java
An array is mirror inverse if the array elements swapped with their corresponding indices are equal to themselves.
Code :
Output :
Approach for it can be :
- Traverse the array.
- If array[array[i]] is equal to index then true.
- Else false.
Conclusion
- Java is a simple, object-oriented, robust, platform-independent, concurrent, and secured programming language for application development by James hosling.
- To create a simple java program, follow the following steps :
- Create a file and copy the code in the file named “firstprog.java” (you can name anything)
- Compile it by using the keyword “javac” and the filename: javac firstprog.java
- Execute the program by using the “java” keyword along with the filename: java firstprog.
- Parameters used in the java program.
- Class definition :
to create a new class in java, the “class” keyword is used. - Main method :
it is the main method in the java program and should be included in every java program. - public :
the method is initialized as public so that it can be accessed from anywhere. - static :
This is written so that the main method can be called by using an object. - void :
it is the returns type of the method and void means that the main method does not return anything. - main() :
it is the name configured in JVM. The compiler executes the code starting from the main method. - String[] :
the main method accepts input as arrays of strings as an argument. - System.out.print() :
It is used for printing the text in the output. The system is a predefined class used for accessing the system, and out is the variable used for the output stream which is connected to the console.