Java SimpleDateFormat
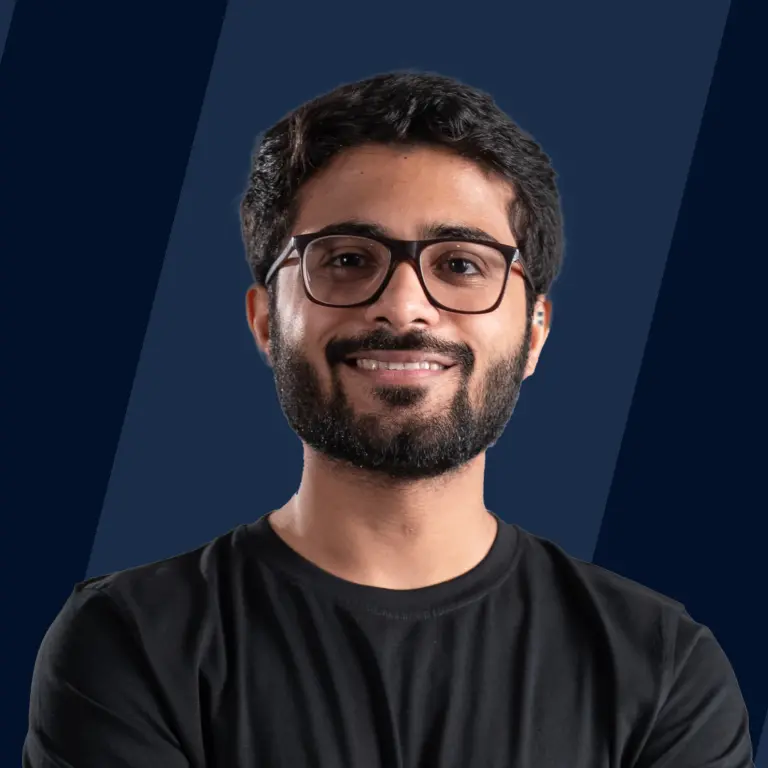
The java.text.SimpleDateFormat class formats and parses dates in Java, extending DateFormat. It supports custom date patterns and locales, enabling conversion between Date objects and their string representations.
Declaration
We can declare the SimpleDateFormat class in the following way. It extends the SimpleDateFormat class and it contains all the methods of the base class along with its own.
Constructors of the Class SimpleDateFormat
Sr. No | Constructors | Description | Examples |
---|---|---|---|
1 | SimpleDateFormat() | Constructs a SimpleDateFormat using the default pattern and date format symbols for the default locale. | SimpleDateFormat p = new SimpleDateFormat(); |
2 | SimpleDateFormat(String pattern) | Constructs a SimpleDateFormat using the specified pattern and the default date format symbols for the default locale. | SimpleDateFormat p = new SimpleDateFormat("yyyy MM dd"); |
3 | SimpleDateFormat(String pattern, DateFormatSymbols formatSymbols) | Constructs a SimpleDateFormat using the specified pattern and date format symbols. | DateFormatSymbols symbols = new DateFormatSymbols(new Locale("en", "US")); symbols.setAmPmStrings(new String[] {"AM", "PM"}); SimpleDateFormat p = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss a", symbols); |
4 | SimpleDateFormat(String pattern, Locale locale) | Constructs a SimpleDateFormat using the specified pattern and the default date format symbols for the given locale. | SimpleDateFormat p = new SimpleDateFormat("yyyy-MM-dd", Locale.US); |
Java SimpleDateFormat Examples
Example 1: Date to String
This example demonstrates formatting a date as a string using SimpleDateFormat.
File: FormatDate.java
Example 2: String to Date
This example shows how to parse a date string back into a Date object with SimpleDateFormat.
File: ParseDate.java
Read more about converting strings to dates in Java on Scaler.
Methods Summary
Sr. No | Method | Return data-type | Description |
---|---|---|---|
1. | parse() | Date | This method is used to parse text from a string to form and return the Date. |
2. | set2DigitYearStart(Date startDate) | void | This method is used to parse the date and set the date in the range startDate to startDate + 100 years. |
3. | get2DigitYearStart() | Date | This method is used to get the start of a period of 100 years set by the user during parsing. |
4. | toLocalizedPattern() | String | This method is used to return the date pattern String used in the formatter. |
5. | format(Date date) | String | This method is used to convert a Date to a string. |
6. | applyPattern(String pattern) | void | This method is used to set the given pattern to the Date Format. |
7. | toPattern() | String | This method is used to return a pattern describing the string. |
Methods in Detail
1. parse()
- Return type- Date
- Description- This method is used to parse text from a string to form and return the Date.
- Parameters- None
Example-
Output-
The above code demonstrates the use of the parse() function. We have created a SimpleDateFormat MM/dd/yy format, created a Calender instance parsed the string, and printed the date and time. Any error occurring is caught in the catch block.
2. set2DigitYearStart(Date StartDate)
- Return type- void
- Description- This method is used to parse the date and set the date in the range startDate to startDate + 100 years.
- Parameters- Date startDate
Example-
Output-
The above code demonstrates the use of set2DigitYearStar function. We have created a SimpleDateFormat MM/dd/yy format, created a Calender instance, and set a different year using set2DigitYearStart() function and printed the date and time. Any error occurring is caught in the catch block.
3. get2DigitYearStart()
- Return type- Date
- Description- This method is used to get the start of a period of 100 years set by the user during parsing.
- Parameter- None
Example-
Output-
The above code demonstrates the use of get2DigitYearStart() function. We have created a SimpleDateFormat MM/dd/yy format, created a Calender instance set a year using set2DigitYearStart() function, and printed the date and time. As the get2DigitYearStart() checks and returns the year set by the user, we get the year that was set by us previously. Any error occurring is caught in the catch block.
4. toLocalizedPattern()
- Return type- String
- Description- This method is used to return the date pattern String used in the formatter.
- Parameter- None
Example-
Output-
The above code demonstrates the use of toLocalizedPattern function. We have created an object using the default constructor and then created a calendar instance. The current date and time are printed and we used the format function to format the Date to a String and then printed the same.
5. Format(Date date)
- Return type- String
- Description- This method is used to convert a Date to a string.
- Parameter- Date date
Example-
Output-
The above code demonstrates the use of the format function. We have created a SimpleDateFormat and created an instance of a calendar object and then formatted the current time and displayed it.
6. applyPattern(String Pattern)
- Return type- void
- Description- This method is used to set the given pattern to the Date Format.
- Parameter- String pattern
Example-
Output-
The above code demonstrates the use of applyPattern() function. We have created a SimpleDateFormat and created an instance of a calendar object. Now as we want to apply a pattern, we first define it and pass it as an argument to the applyPattern function. The current date and the pattern are displayed to the user.
7. toPattern()
- Return type- String
- Description- This method is used to return a pattern describing the string.
- Parameters- None
Example-
Output-
The above code demonstrates the use of toPattern() function. We have created a SimpleDateFormat and created an instance of a calendar object. Now we want to apply a pattern, we first define it and pass it as an argument to the applyPattern function. We then print the pattern that has been applied earlier using the toPattern function. The current date and the pattern are displayed to the user.
Conclusion
- The Java SimpleDateFormat class transforms dates into readable strings and vice versa, bridging the gap between textual and date formats.
- It's a concrete utility for handling date-time representations, customizable with patterns, locales, and time zones.
- With various constructors and methods, SimpleDateFormat offers flexibility in date formatting and parsing operations.
- Practical examples demonstrate its ease of use and adaptability, from straightforward formatting to complex locale-specific conversions.