What is the Size of a Pointer in C?
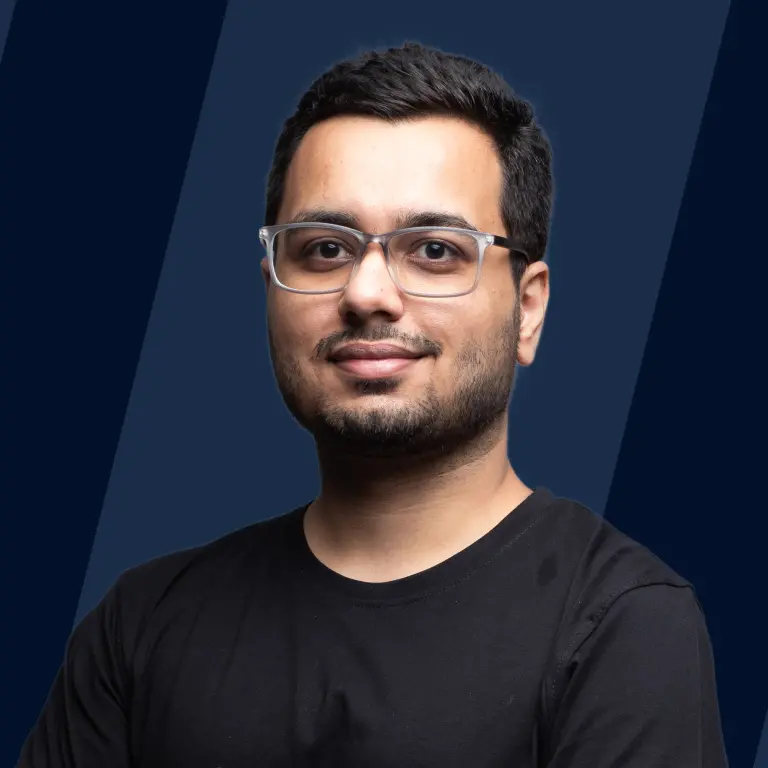
Pointer in C is just a variable that could store the address of the other variable. In C size of a pointer is not fixed as it depends on Word size of the processor. In general a 32-bit computer machine then size of a pointer would be 4 bytes while for a 64-bit computer machine, it would be 8 bytes.
Syntax
In syntax, we represent the variable name after an asterisk *
Example
Observe the below example
Here, ptr is the name of the variable, which is of type Integer (int). Also, note that an asterisk is used as a prefix before the variable name. asterisk is mandatory to add as a prefix to the variable name, if we don't add then it will not be treated as a pointer variable.
What is the Use of Size of Pointer in C?
Size of Pointer in C is useful for determining the memory space occupied by the machine.
It is useful as it can specify how many bytes of memory is occupied in memory.
Factors on Which the Size of Pointer in C Depends
The size of a pointer in C depends on the factors like Processor word size and CPU Architecture.
Processor Word Size is the amount of data that internal data registers of the CPU can capture and process the data at a time.
To keep it simple, Processor word size determines the size of the pointer. As a matter of fact, the Processor word size is the same for a particular computer machine, and the size of the pointer in C also remains the same. So, the size of the pointer is purely dependent on the word size of the processor, irrespective of the data type of variable whose address is being stored.
How to Print the Size of Pointer in C?
To print the size of a pointer in C, we use a sizeof() operator.
Syntax:
For example, let us consider a pointer variable as int *ptr;. As ptr is the pointer variable here, we can determine its size by sizeof(ptr);.
Size of Pointer in C of Different Data Types
Size of Character Pointer
As we already discussed, the size of a pointer variable is purely dependent on Processor Word Size, let's explore what the size of a character pointer in a 64-bit processor is.
So, we already know sizeof() operator to find the size of a pointer variable. Here, let's make use of it to find out the size of a pointer variable of type char.
Output:
In the above program, we initialized a character variable and assigned its address to a pointer variable of type char. Later, we used sizeof() operator to find the size of this pointer variable.
Size of Double Pointer in C
Here, let's find out the size of a double pointer in C using our sizeof() operator itself. Let's use 64-bit processor to find out the size of the Double Pointer in C using sizeof() operator.
Output:
Size of Pointer to Pointer
To mention again, the size of a pointer in C is purely dependent on the word size of the processor, so when we talk about pointer to pointer, it should also reflect the same values.
So, even if we talk about pointer to pointer, the values should be 8 bytes for 64-bit processor, 4 bytes for a 32-bit processor, 2 bytes for a 16-bit processor
Output
Size of Pointer to an Array
Size of Pointer to an array also remains the same as the size of the pointer variable as they are purely dependent on the processor's word size.
Let us check the size of pointer to an array using a C program
Output:
So, From the output of the above we can surely conclude that size of a pointer to an array is 8 bytes itself as it depends on the Processor Word Size.
C Program to the Size of Pointers to All Data Types is Same
Any kind of pointer variable would occupy the same size of memory as a pointer value is a memory address which is an unsigned integer. But, the variables would be occupying different sizes of memory blocks according to their data type.
Output:
C Program to Find Out the Size of the Different Data Types
As we know there are multiple data types in C programming language, here let us see the size of each and every data type by implementing a C program.
We will use sizeof() operator to find the size of data type and we will use printf to print it on the screen.
Output:
Conclusion
By this time, you might have got a good idea about What is Size of pointer in C, to quickly recollect what we discussed, let’s see a few points.
- We use asterisk (*) symbol to denote a pointer variable
- Size of Pointer in C depends on word size of the processor
- sizeof() operator is used for printing size of a pointer variable
- Size of pointer variable is same for every data type. It would be 8 bytes for 64-bit processor, 4 bytes for 32-bit processor, 2 bytes for 16-bit processor.