Smith Number in Java
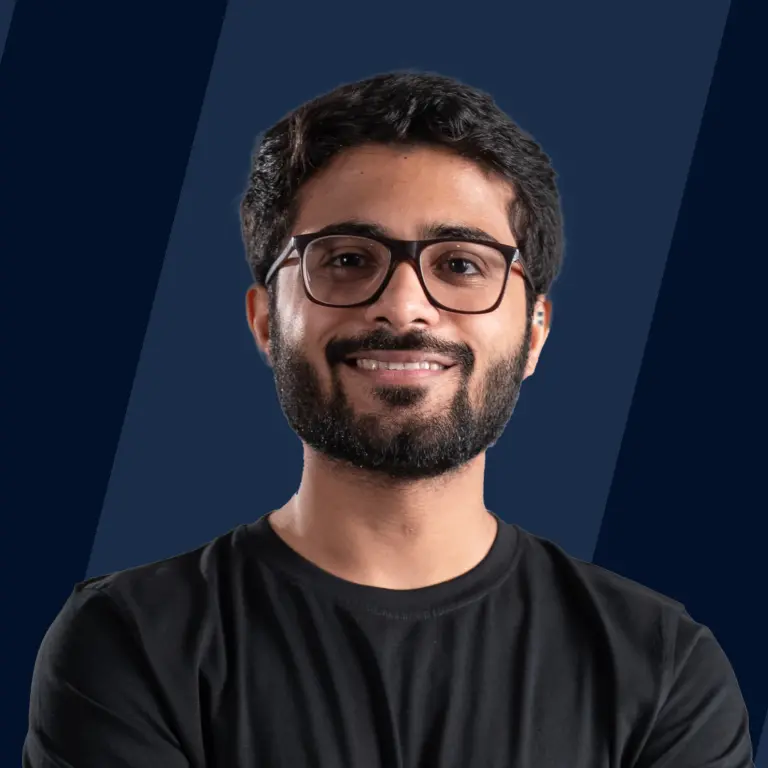
A composite number is a positive integer greater than 1 which can be represented as the product of prime numbers. This is called factorization. Composite numbers have at least one positive divisor other than 1 and itself.
Smith number in Java is a composite number whose sum of the digits equals to the sum of digits of its prime factors including 1. Smith numbers are also called Joke NUmbers and are listed in OEIS A006753.
Sum of digits of NUMBER = Sum of digits of PRIME FACTORS of NUMBER
To check if the given number is a Smith Number or not, we will have to find the sum of the digits of the given number. Next, we will find the sum of the digits of all the prime factors of the number. Finally, if these two sum values are the same then we can say that the given number is the Smith number.
We may barely use the Smith number, but implementing it in code improves our understanding of language along with mathematics. This helps in building strong problem-solving fundamentals too which will be useful in our programming journey.
Smith Number Example
Let us see some examples to better understand Smith Number in Java. We will find all the prime factors and find the sum of their digits as well as the sum of the digits in the number itself.
Example 1:
Example 2:
Example 3:
How to Check Smith Number in Java?
Let us now see the algorithm to check if the given number is a Smith Number in Java or not.
-
Let us begin with the easiest task i.e. to find the sum of digits of the given number.
- This can be simply done as follows:
- take a variable sum = 0.
- Now repeat the below steps until the number is greater than 0 using a while loop.
- Get the digit at the unit's place by taking the modulus with 10 and adding it to the sum. Now, divide the original number by 10 to get the rest of the digits.
-
Next we will find the prime factors of the given number.
-
We know that the maximum prime factor is always less than or equal to the square root of the given number.
- Every number can be expressed as the product of two factors F and G (say 56 => 7 * 8 or 14 * 4 or 28 * 2 or 56 * 1).
- Now, the square root of 56 is 7.48. In all the pairs we saw above, each contains a number less than 7.48 and a number greater than it. Thus, if F > root(N) then G has to be greater than root(N).
- Thus, the prime factor can never be greater than the root(N). The greater factor will always either be equal to the root(N) or will be the product of primes.
-
So starting from i = 2 (as 1 is neither prime nor composite and 1 is always a divisor of a number) we will loop till the square root of the given number.
-
In each iteration the number i is checked if it is divisible by our number. If it is divisible we run another loop until the i is divisible.
-
Inside this inner loop we divide our number by i as well as we get the sum of digits of i using the previous function and add it to the sum.
-
-
Finally, we compare the two sums. If the sums are equal we declare our number as Smith Number or Joke Number else not.
Smith Number Java Program
In the driver method or main method we will call the function isSmithNumber() which returns a boolean value. This function takes an integer value as input and returns true if it is a Smith number, else it returns false.
Output:
Note that code is divided into several methods rather than clustering everything into one method. This is a good programming practice as it enhances the readability of the code and provides reusability (the sum of digits is used in the isSmithNumber as well as primeFactorSum).
Let us perform a dry run for the number 85.
-
Input number: 85
-
Main method calls isSmithNumber(85) isSmithNumber(85) => Calls digitSum(85) and primeFactorSum(85)
-
digitSum(85) digitSum(85) =>
-
1st Iteration:
- 85 % 10 => 5
- sum = 0 + 5 => 5
- 85 / 10 => 8
-
2nd Iteration:
- 8 % 10 => 8
- sum = 5 + 8 => 13
- 8 / 10 => 0
-
Sum of digits: 13
-
-
primeFactorSum(85)
- primeFactorSum(85) => primeFactorSum(5 * 17)
- => digitSum(5) + digitSum(17)
- => 5 + (1 + 7)
- => 5 + 8
- => 13
- Sum of prime factors' digits: 13
-
Compare digit sum and prime factor sum isSmithNumber(85) => 13 (digit sum) == 13 (prime factor sum)
-
Result: The number 85 is a Smith number.
Conclusion
- A Smith Number in Java is defined as a number in which the sum of its digits is equivalent to the sum of the digits of all its prime factors.
- Two very important, useful logical concepts are covered in this problem i.e. prime factorization of a number and calculating the sum of digits of a given number.
- Implementing logic for Smith number helps us to understand implementations of mathematical concepts in programming, and improves algorithmic skills, as well as by providing a coding challenge it allows us to identify patterns and characteristics.
- This requires us to calculate the sum of digits of the given number and compare it will the sum of (sum of digits) of all the prime factors of the given number.