Snake Game in Python
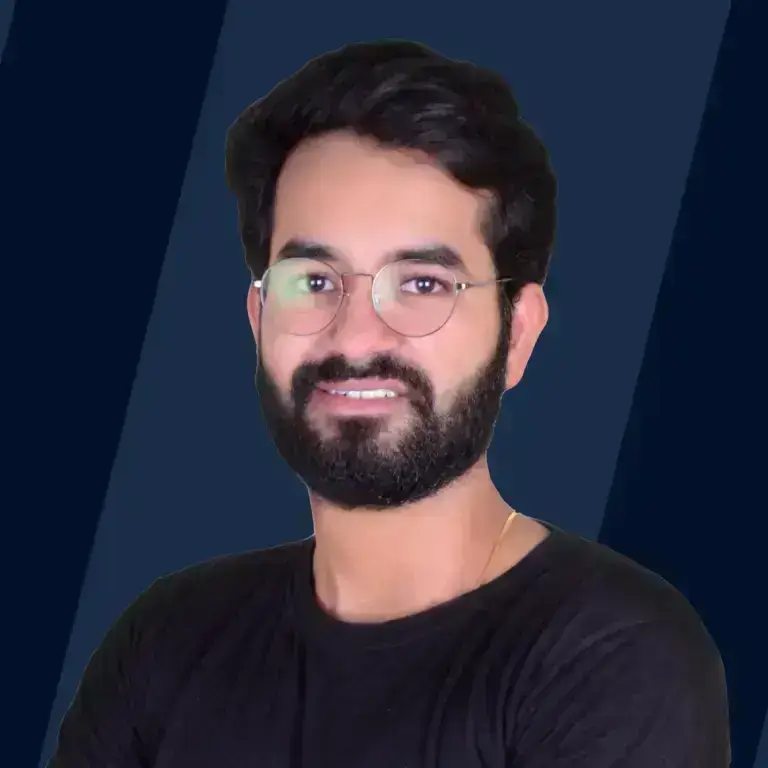
Overview
Building fun and interesting video game like Snake game in Python would not only help us learn, understand and explore the concepts in Python but will also be a good start for any beginner programmer to challenge themselves. The Snake game in Python has a clear objective of catching the maximum number of fruits without hitting the cross border or the wall as they say. With the help of the Pygame library, we shall be learning building the Snake game in Python from scratch.
What We are Building?
You might have played the most loved arcade - Snake game in Python when you were young. With the below article, we shall learn how to make the Snake game in Python and see the 'Snake' make moves to our beats!!
The snake game in Python is played with the prime objective to catch the maximum number of fruits without hitting the cross border or the wall as they say. It was created by Gremlin Industries company and later on published by Sega in October 1976. The snake game in Python has gained wide popularity among all age groups for many generations. The Snake game in Python is controlled with the four direction buttons on our keyboard concerning the direction in which the snake runs with the clear goal of eating maximum food and eventually scoring maximum points until the snake strikes the wall or itself.
While we shall be building the Snake game in Python by following along with this article it's important to understand the prime objective of the Snake game in Python along with the various prerequisites and also visualize the Snake game in Python to help us connect and understand the flow better.
Let's dive in!
Pre-Requisites
The major topics that we should refresh before we jump straight towards building the Snake game in Python are as follows:
- User-defined functions in Python.
- Python pygame.Color() function
- Python pygame.time.Clock() function
- Python random.randrange(start, stop, step) method
- Python pygame.display.set_caption() method
- Python pygame.display.set_mode() function
- Pygame pygame.render() method
- Pygame .get_rect() function
- flip() method in Pygame
- Pygame .blit() method
- time.sleep() in Python
- pygame.quit() in Pygame.
- pygame .midtop() function
- for loop in Python
- if statements in Python.
- if- else loop in Python
- .insert() in Pygame
- .fill() in Pygame
How are We Going to Build the Snake Game Using Python?
The algorithm that would help us visualize the whole logical flow of building the Snake game in Python is explained below:
- We start by importing the necessary libraries like pygame, time module and random module. Then we head to defining the snake_speed as 10 and the width and height of the game window as 960 and 720 respectively.
- The second step includes the initialization of the Pygame and controls the FPS (frame per second) to make the speed of the snake flexible by using the pygame.init() function and implementing the pygame.time.Clock() to control the frames per second.
- Then we start initializing the Snake positions, Food positions, and direction of Snake on the game window. We create the snake by assigning 5 blocks to the snake body and allocate the food for the snake in a random manner within the game window using the random.randrange(start, stop, step) function in Python. We also assign the direction to the snake at the onset of the game.
- Now we head towards, creating UDF (user defined function) for displaying the score earned while playing the snake game in Python where we start by declaring the starting score as 0, followed by creating the UDF function score_metrics for displaying the score earned inside which we are choosing the font and its related size as well.
- Our fifth step includes building the UDF - end_gameover() for the scenario when the snake shall hit the wall or eat itself and the Game is OVER! We also give a 5-second wait time by implementing the sleep() function from the time module in Python.
- The final step is to create the main function for the entire Snake game in Python to work where we make sure the following scenarios are checked:
-
To validate the keys responsible for the movement of the snake using the for loop in Python and if statements in Python.
-
As we don't want our snake to move in the opposite direction that is RIGHT in our case, instantaneously we shall be implementing the if statements in Python.
-
Snake body growing mechanism - When the snake and its food collide the snake body will grow for which we shall increment the score by 15 and enable the new food to be spanned.
-
If the condition around the game getting 'OVER' once the snake in the snake game in Python hits the wall is validated then the end_gameover() function is supposed to be called.
-
If the condition around the game getting 'OVER' once the snake in the snake game in Python eats itself is validated then the end_gameover() function is supposed to be called.
-
Displaying the scores using the score_metrics function.
-
Final Output
Well! it's better understood when we see things rather than just listening or reading about them. With that being said, below is what our Snake game in Python would look like when we have created it from scratch.
Below is the image of what our program might look like:
Requirements
The requirement for building the snake game in Python are listed as follows:
- Preinstalled Python 3.0 for building the project on your machine.
- Pygame: To build the Snake game in Python we shall be using the open-source library specifically designed for creating video games as it contains inbuilt graphics and sound libraries. This is very beginner-friendly and cross-platform.
- Time module: For tracking the number of seconds that have elapsed as compared to the previous time, we make use of the time module.
- Random module: With the random module we can generate numbers randomly which will be used as we follow along with the article to build the snake game in Python.
- Text editor: We can use either VSCode Python 3 installed on your machine or we can implement the source code with any online Python compiler as well.
- Turtle Library: By implementing the turtle library which comes pre-installed with Python. To create shapes and pictures the turtle library is wide as it provides a virtual canvas.
Building Snake Game Using Python
Now as we are equipped with all the libraries, modules, and other requirements needed for the project and we have also refreshed our knowledge of all the topics marked as pre-requisites for building the snake game in Python, let's get our hands dirty and build the Snake game in Python from scratch.
Step 1: Always Import all the Important and Necessary Libraries along with Defining the Game Board
Open the command line interface or any local terminal to install Pygame by using the below code. You also need to install certain important libraries that we shall implement for building the Snake game in Python:
Once our modules are installed we shall start to define the width and height of the window where we shall be playing our game. For displaying the text that will be used in the game, we will define the color in RGB format using the pygame.color() function.
Code:
Explanation:
As seen above we are ready with our libraries and module and now we can start building the fun and cool snake game in Python. We also defined the width and height of the text that will be written. Also, we defined the colors for the text that will be visible on the game board by using the pygame.color().
Step 2: Starting to Initialize the Pygame and Control the FPS (frame per second) to Make the Speed of the Snake Flexible
Now we shall start to initialize the Pygame by using the pygame.init() function. After creating the game window as we did in the previous step where we pre-defined the width and height we shall now move towards using the pygame.time.Clock() function to make the speed of the snake flexible enough to adjust to changes in the speed of the snake.
Code:
Explanation: In the above code snippet we have initialized the pygame by simply using the pygame.init() function. After which we initialize the window of the game by displaying the caption - Snakes game in Python using the pygame.display.set_caption() function where we also created a variable named window_snakegame to set the mode with the width and height of the pre-defined game window. We then control the speed of the snake, by controlling the frame per second using the pygame.time.Clock() function.
Step 3: Initializing the Snake Positions, Food Positions, and Direction of Snake on the Game Window
With this step, we shall be initializing the snake's position in the game window. After that is done, we also need to initialize the food's position random manner in the defined width and height of the game window. Also, when the game starts we need the snake to move in a particular direction so that every time the user runs the program of the snake game in Python, the snake moves in that particular set direction. For this code base, we are setting the direction of the snake as 'LEFT'.
Code:
Explanation: As viewed from the snippet of the code, we have first defined the snake's position on the game window by the variable - position_snake. Then we created the snake body length by allocating 5 blocks from the game window as can be seen from the code for the variable - body_snake. Now, for placing the food in a random manner inside the game window, we made use of the random module function random.randrange(start, stop, step) from which we select a random number within the specified range. As we want to set the direction of the snake to be in LEFT so that every time a user starts the game the snake starts to travel from the LEFT side of the game window.
Step 4: Creating UDF for Displaying Score Earned While Playing the Snake Game in Python.
In the fourth step, we shall be creating a UDF(user-defined function) that will build a font object where we shall define the font color with an appropriate size allocated for the score variable. Then we shall make use of the render function in Python to build the background surface which will change as the score updates. As the text gets refreshed, we need to create a rectangular object referencing the text surface object by implementing the .get_rect() function. Using the blit() function we will display the score that is earned while playing the snake game in Python. For the screen.blit(background,(x,y)) function usually takes two arguments where (x,y) is the position inside the window where we want the top left of the surface to be. The function tells to take the background surface and draw it onto the screen by positioning it at (x,y).
A simple example:
Code:
Explanation:
The code snippet illustrates the UDF score_metrics created for the score and its related metrics that are being earned while playing the snake game in Python. The score earned could be displayed by calling the score_metrics UDF and the updated score is displayed.
Step 5: Building the UDF for a Scenario When the Snake shall Hit the Wall or Eat itself and the Game is OVER!
We shall be creating a UDF for defining the condition around the game getting 'OVER' once the snake in the snake game in Python either hits the wall or eats itself. For doing so, we shall start by first creating a font object which will display the scores earned so far. To render scores, we create a text surface for the text to be drawn, for which we again create a rectangular object for the text surface object.
As we want the message of "GAME OVER" to appear in the middle of the game window of the snake game in Python, we set the position of the text surface object in the middle. Then as we need to show the text on screen we use the blit() function. As the game goes on, the score updates which we shall display by updating the surface by implementing the flip() function. Before we can close the game window, we give a 5-second wait time by implementing the sleep() function and eventually quit the game by using pygame.quit() function.
Code:
Explanation:
With the code written above, we created the UDF end_gameover that covers the scenario when the snake shall hit the wall or eat itself and the Game is OVER! We have declared variable and implemented functions like variable font_displayscore for creating a font object which will display the scores earned so far, variable surface_gameover for text surface object on which the text for scoring and all will be written, variable rect_gameover to create a rectangular object for the text surface object, window_snakegame.blit() function to show the text on screen we using the blit() function and pygame.display.flip() function for updating the score by updating the surface using flip() function. Eventually, we want to give a 5-second wait time by implementing the sleep() function and finally quit the game by using pygame.quit() function.
Step 6: Creating the Main Function for the entire Snake Game in Python to Work.
Creating the main function will allow the snake game in Python to do the following listed points:
- To validate the keys responsible for the movement of the snake using the for loop in Python and if statements in Python.
- As we don't want our snake to move in the opposite direction that is RIGHT in our case, instantaneously we shall be implementing the if statements in Python to resolve this.
- We want to make sure that when the snake and its food collide the snake body will grow for which we shall increment the score by '15' and enable the new food to be spanned. We shall do this by using the if- else loop in Python, .insert() in Pygame , .fill() in Pygame and random.randrange() in Python.
- Checking if the condition around the game getting 'OVER' once the snake in the snake game in Python either hits the wall or eats itself is validated then the end_gameover() function is supposed to be called.
- Displaying the scores using the score_metrics function.
Code:
Explanation: As can be remarked from the code written above, we have created the main function that will help us to play the snake game in Python keeping in mind the rules and objective of the game. We validated the keys responsible for the movement of the snake using the for loop in Python and if statements in Python. We also checked if our snake moves in the opposite direction that is, RIGHT in our case, instantaneously we shall be implementing the if statements in Python to resolve this. When the snake and its food collide the snake body will grow for which we shall increment the score by 15 and enable the new food to be spanned. We shall do this by using the if- else loop in Python, .insert() in Pygame , .fill() in Pygame and random.randrange() in Python. If the condition around the game getting 'OVER' once the snake in the snake game in Python either hits the wall or eats itself is validated then the end_gameover() function is supposed to be called. Lastly, we are displaying the scores using the score_metrics function.
It's Gameplay Time!
We shall now combine all the code snippets that we just created and see how the snake game in Python works.
Code:
Output:
Extra Features That Can Be Added To The Game
Hurray! Now that you have to build your snake game in Python by following along with the article, we can now start to challenge the technicality and play around with Python by adding a few new features in the snake game in Python. For doing so, we can use the pre-installed Turtle library to implement the snake game in Python. By providing the user with a virtual canvas, the turtle library allows its users to print patterns and images.
You can try experimenting with the turtle library in Python to explore more features in the snake game in Python.
Conclusion
-
The Snake game in Python is controlled with the four direction buttons on our keyboard concerning the direction in which the snake runs with the clear goal of eating maximum food and eventually scoring maximum points until the snake strikes the wall or itself.
-
It's recommended to refresh the pre-requisites section before you start to build the snake game in Python.
-
While building any game all the scenario that may arise while playing the game needs to be handled and hence we provided the algorithm before jumping into the coding part where the foundation of the logical flow of the snake game in Python was laid out.