Sort Hashmap by Value
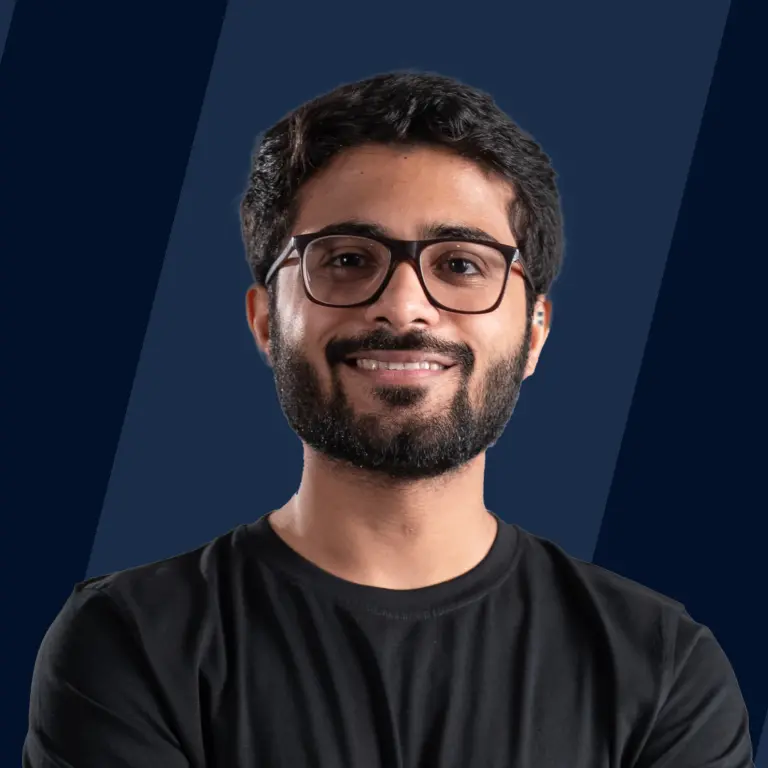
Overview
HashMap implements the Map interface of Java. It stores data in (Key, Value) pairs, and can be accessed by an index of another type. HashMap can be Sorted by values by using Collections.sort(), TreeMap, Java 8, Lambdas, Streams in Java 8.
Introduction
The Map interface, which lets us store key and value pairs with unique keys, is implemented by the Java HashMap class. The element of the relevant key will be replaced if you attempt to insert the duplicate key. Utilizing the key index makes actions like updating, deleting, etc. simple. The java.util package contains the HashMap class.
Similar to the classic Hashtable class in Java, HashMap lacks synchronisation. Although there should only be one null key, it enables us to store the null components as well. It is referred to as HashMap<K,V> since Java 5, where K stands for key and V for value. It implements the Map interface and derives from the AbstractMap class.
HashMap can be sorted by values using
- Collections.sort()
- TreeMap
- Lambdas
- Stream
Method 1: Java Collections.sort() Method
Collections.sort()
syntax
The java.util.Collections class has the java.util.Collections.sort() function. The elements in the supplied list of the Collection are sorted in ascending order using this method.
It functions similarly to the java.util.Arrays.sort() method, but it is superior because it can sort both the linked list and queue elements as well as the array's own elements.
Comparator
In order to rank the objects of user-defined classes, a comparator interface is utilised. A comparator object can compare two objects that belong to the same class.
Steps to Sort Hashmapby value using Collections.sort()
- Create a Hashmap
- Copy Elements of Hashmap into ArrayList
- Sort ArrayList using Collections.Sort()
- Transfer the elements back to Hashmap
Code
Output
Code Explanation
In the create hashmap() function, we first built a HashMap and entered values in it. Then, in transfer elements we transferred every element from the hash map into an array list. After that, the ArrayList was sorted based on the value of the HashMap Node. At the end we have printed all of the items in a hash map and transferred all the elements to it.
Method 2: Using a TreeMap
TreeMap is an implementation of NavigableMap based on Red-Black trees. It is arranged in the order that its keys naturally appear. Similar to the HashMap class, the TreeMap class implements the Map interface. The primary distinction between both is that TreeMap is sorted in the ascending order of its keys, whereas HashMap is an unordered collection. TreeMap is an unsynchronized collection class, hence unless explicitly synchronised, it cannot be used for thread-safe operations.
Steps to sort hashmap by values using TreeMap
- Create a TreeMap with custom comparator
- put all key value to TreeMap
- return TreeMap
Output
Code Explanation
In the Above code, we have first we have created a hashmap and added some data into it. Then called a function sort_by_values that will take hashmap as a parameter and create a Treemap with custom comparator and return that Treemap.
Method 3: Using Java 8 Lambdas
Lambda expressions are a brand-new and crucial feature that were added to Java SE 8. It offers a simple and direct approach to use an expression to represent one method interface. In a library's collection, it is quite helpful. It is advantageous to filter, iterate, and extract data from a collection.
The implementation of an interface with a functional interface is provided using the Lambda expression. Quite a bit of code is saved. In the case of a lambda expression, the method does not need to be defined again in order to provide the implementation. Just the implementation code is written here.
This is same as Collection.sort() but we will be using lambdas.
Output
Method 4: Using Streams in Java 8
The Stream API, introduced in Java 8, is used to process collections of objects. A stream is a collection of objects that can support several operations and be pipelined to create the desired outcome.
The capabilities of Java stream include:
-
A stream accepts input from Collections, Arrays, or I/O channels; it is not a data structure.
-
Streams just deliver the results of the pipelined operations; they don't alter the underlying data structure in any way.
-
Stream is lazy and only evaluates code when it's necessary.
-
During the course of a stream's life, the elements are only encountered once. Similar to an iterator, a new stream must be created in order to return to the same source material.
Here, streams will be used to sort Hashmap by values. We will first utilize the stream() method to obtain the stream of the entrySet, sort the stream using a lambda expression within the sorted() function, and then convert the stream into a map using the toMap() method. To keep the map's sorted order, we use the LinkedHashMap::new method reference inside the toMap() method.
Output
Code Explanation Our Above Code uses stream() function, which creates a sequential stream, then sorts based on value, and then uses Collectors.toMap(KeyMapper,ValueMapper,mergefunction,mapSupplier) that Returns a Collector that accumulates elements into a Map .Where KeyMapper(Map.Entry::getKey) a mapping function that produce keys ValueMapper(Map.Entry:getValue) a mapping function that produce values,mergefunction((e1, e2) -> e1) in case of duplicate keys this function will be used to resolve for example if e1 and e2 are equal we will be going to use e1,mapSupplier(LinkedHashMap::new) result will be stored in a LinkedHashMap.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
- HashMap implements the Map interface of Java . It stores data in (Key, Value) pairs, and can be accessed by a map.
- HashMap can be sorted on the basis of Values Collections.sort(),TreeMap,Lambdas,Stream
- In java, Collection.sort() sorts Lists, so by converting a HashMap into a List, a HashMap can be sorted using Collection.sort().
- By Using TreeMap with custom comparator HashMap can be sorted
- By using Lambdas HashMap can be sorted
- By Converting Hashset to sequential stream HashMap can be sorted.