JavaScript String.prototype.split()
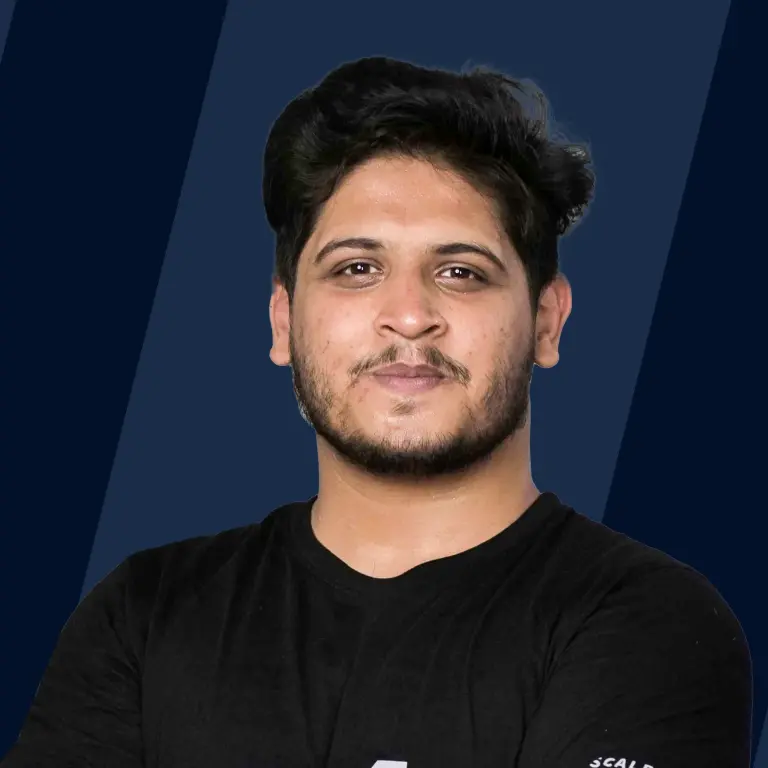
Overview
To separate or split a string into an ordered list or an array of strings according to the provided separator, we use a method called split in javascript. The split in javascript takes two optional parameters, namely: separator and limit. The separator can be a simple string or a regular expression. The separator resembles the point or pattern where each split should take place. The limit is a non-negative integer that defines the higher limit of the number of pieces to split the given string into.
Syntax of String split() in Javascript
The syntax of a split in javascript is as follows:
Here, the string is invoking the split() method.
Parameters of String split() in Javascript
As we know, we can use the split in javascript to split the string into an array of strings as per the separator provided. So, we need to provide the separator as one of the parameters in a split in javascript.
The split in javascript takes two optional parameters. The two optional parameters of a split in javascript are separator and limit.
-
separator: separator is an optional parameter. The separator can be a simple string or a regular expression. The separator resembles the point or pattern where each split should take place. If the separator has more than one character, then the entire sequence of characters should be present in the invoking string. If the provided separator is not present in the invoking string, then the entire string becomes a single array of the element. Even if the separator is present at the beginning of the end of the string, the split in javascript works.
-
limit: the limit is also an optional parameter. The limit is a non-negative integer that is used to define the higher limit of the number of pieces to split the given string into. Whenever we provide a limit along with the separator, the split works until the limit entries have been placed in the array.
Note:
- An array can contain fewer entries than the provided limit in cases when the end of the string is reached before the limit is reached.
- If the separator is an empty string (""), then the string is converted into an array of each of its UTF-16 characters.
Return Value of String split() in Javascript
Return value: ordered list or array of strings.
The split in javascript does not change the original string. The split in javascript returns an ordered list or an array of strings formed after splitting the method invoking a string at each point where the separator has occurred.
Note: When the split() method calling string is empty, the split() method does not return an empty array. The split() method returns the array with an empty string case of an empty string. The empty array is returned when both the string and the separator are empty strings.
Exceptions of String split() in Javascript
The split() method in javascript does not raise an error or exception if we use the correct syntax. Since both the parameters of the split in javascript are optional hence, we can or cannot provide the separator and the limit.
The split() method in javascript does not raise any exception or error even if the method calling string is empty. The split() method returns the array with an empty string in case of an empty string but does not return an empty array.
The split() method in javascript returns an empty array only when both the string and the separator are empty strings.
Example of String split() in JavaScript
Now, we know what is split in javascript and how the split() method works in javascript. Let us take a few examples to understand the method better.
Example:
Output:
As we can see in the example above, the split in javascript returns an array of strings by splitting the method calling string (s) wherever (space) occurs.
What is String split() in Javascript?
To separate or split a string into an ordered list or an array of strings according to the provided separator, we use a method called split in javascript. The split in javascript takes two optional parameters, namely: separator and limit. The separator can be a simple string or a regular expression. The separator resembles the point or pattern where each split should take place. The limit is a non-negative integer that is used to define the higher limit of the number of pieces to split the given string into.
The split in javascript does not change the original string but returns an array of strings formed after splitting the string at each point where the separator has occurred. If the separator is an empty string (""), then the string is converted into an array of each of its UTF-16 characters.
The split() method returns the array with an empty string in case of an empty string but does not return an empty array. The split() method in javascript returns an empty array only when both the string and the separator are empty strings.
More Examples
Let us take some examples and understand the working of a split in javascript in depth.
1. Using split()
Let us take a string and use a regular expression as a separator to see how the split in javascript works with regular expressions.
Output:
2. Removing spaces from a string
Let us take a sentence with spaces as an example and use split in javascript.
Output:
3. Using limit
So far, we have seen examples where the separator is provided. Let us take an example by providing the limit parameter in the split method in javascript.
Output:
As we can see in the output of the above code, we got only ['Welcome', 'to', 'the', 'Scaler', 'Topics'] rather than ['Welcome', 'to', 'the', 'Scaler', 'Topics', 'platform'] because the limit was set to 5.
Conclusion
- To separate or split a string into an ordered list or an array of strings according to the provided separator, we use a method called split in javascript.
- The split in javascript takes two optional parameters, namely: separator and limit. The syntax of a split in javascript is string.split(separator, limit);
- The separator resembles the point or pattern where each split should take place. The limit is a non-negative integer that is used to define the higher limit of the number of pieces to split the given string into.
- An array can contain fewer entries than the provided limit in cases when the end of the string is reached before the limit is reached.
- If the separator is an empty string (""), then the string is converted into an array of each of its UTF-16 "characters".
- The split() method returns the array with an empty string case of an empty string. The empty array is returned when both the string and the separator are empty strings.
See Also
refer to the articles below to learn about various topics of Javascript on our platform.