Spread Operator in JavaScript
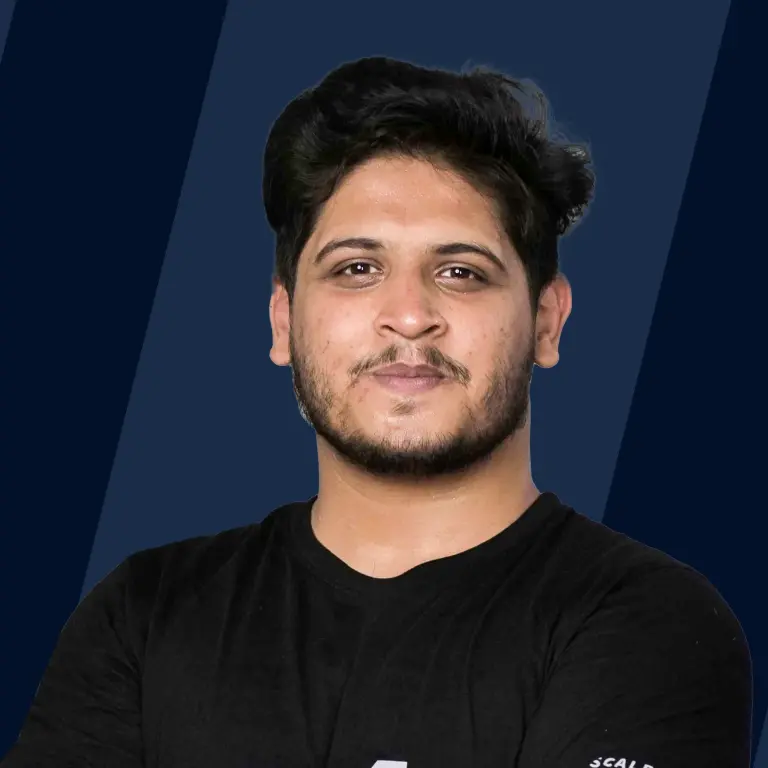
The spread operator is provided by ES6 and consists of three dots (...). A spread operator in JavaScript lets you spread elements from an iterable object, like an array, map, or set.
Syntax of the Spread Operator
The spread operator in JavaScript can be denoted as :
Copy the Array Using Spread Operator
Output:
In the above example of spread operator in JavaScript, the array1 elements will be copied to array2. Any changes made to one array do not affect the other, unlike in the case of assignment operators.
Expand the Array Using Spread Operator
Output:
In the above example of spread operator in JavaScript, we construct the array as shown above. The spread operator expands each character of the string XY into individual items and assigns it to the array1.
Using spread operator with Math Object
Output:
In the above example of the spread operator in JavaScript, we create a function that takes three arguments and performs an addition operation, and prints the answer to the console. Also, there is an array that contains three elements. As you can see, the spread operator is used to pass the arguments into the function.
Spread operators allow array elements to be passed as individual arguments instead of having to pass them individually, such as numbers[0], numbers[1], etc.
Passing elements of the array as arguments to the Math Object
Output:
In Javascript, the Math object does not take a single array as an argument, but by using the spread operator, the array can be expanded into individual arguments. This increases the readability of the code.
Example of Spread Operator with Objects
Output:
Here, in this example, we use the spread operator with object literals. Both the object1 and object2 are added to the object3 using the spread operator.
Copy Array Using Spread Operator
Output:
As we have already learned that the, spread operator in JavaScript can be used to copy the elements of an array into another array. In this case, any change made to one array does not reflect in another array.
But what if we use an assignment operator instead of using the spread operator?
Let's see: JavaScript objects are assigned by reference instead of by value.
Output:
Here, both array1 and array2 point to the same array. This means that changing one variable will change the other.
Now comes the use spread operator when you don't want to change other copied arrays. This means that changing one variable will not change the other.
Output:
Rest Parameter
Rest syntax looks very similar to spread syntax. Essentially, it is the opposite of spread syntax. The spread syntax allows you to "expand" an array into its elements, while the rest syntax permits you to "condense" those elements into a single element.
Output:
The spread operator works for any number of arguments. In the first case, only one argument is given, and in the second case, the spread operator accepts three arguments.
In the below example, only the first three elements are added.
Output:
In the case of multiple arguments to a function, the spread operator takes the required number of parameters and ignores the rest.
Conclusion
-
The spread operator in JavaScript is denoted by three dots (...)
-
An iterable object such as an array, a set, or a map is unpacked into a list by the spread operator.
-
The rest parameter is also denoted by three dots (...). In contrast, it packs every argument of a function into an array.
-
The spread operator can be used to copy an array into another. Unlike the assignment operator, using the spread operator, the original array and copied array are independent of one another.