C++ String Formatting
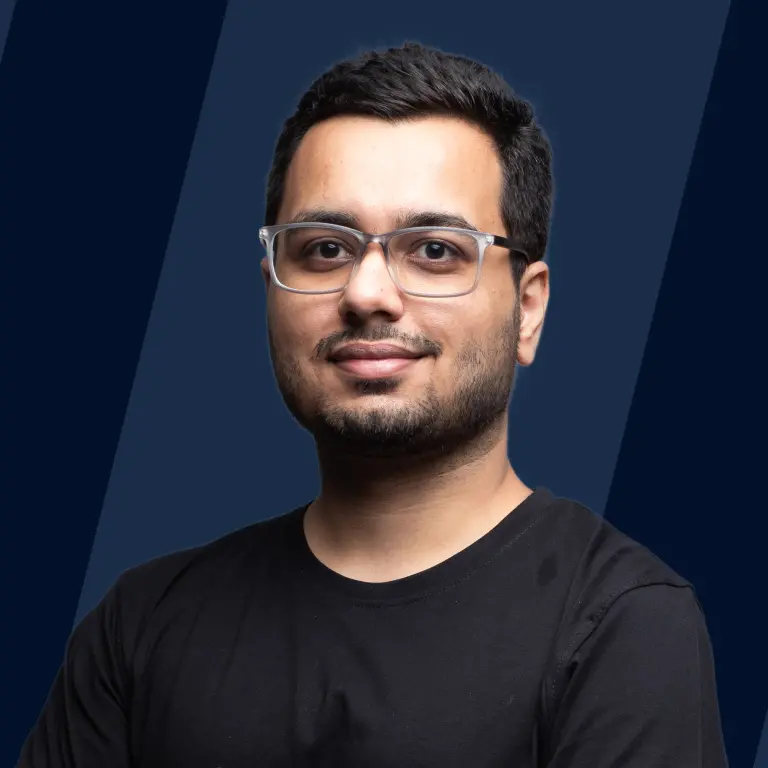
Overview
String formatting refers to placing specific characters within a string in a specific sequence and position. C++ has made numerous "tries" to incorporate text formatting throughout its 40-year history.
The printf() family of functions, inherited from C, came first. It undoubtedly does the job because it is brief, well-known, and "quick". Due to its widespread use, it was so effective that "printf-driven-debugging" became a thing, to the point where debuggers and IDEs now incorporate printf()-like APIs.
A new text formatting API called "format" will be introduced in C++20. It attempts to solve the problems with streams while maintaining printf() simplicity which we will study in this article known as the sprintf Function in C++.
Syntax of C++ String Formatting Function
Parameters of C++ String Formatting Function
- str: This is a pointer to an array of char elements that will hold the final C string.
- format: This string will contain the text that will be put into the buffer. It may optionally include embedded format tags, then formatted as necessary by replacing them with the values from later extra arguments. Prototype format tags: %[flags] [width][.precision] [length] specifier as described below-
Sr.No | Specifier and Ouput |
---|---|
1 | c (Writes a single character to stdout) |
2 | d or i (Signed decimal integer) |
3 | e (Transforms a floating-point number to a decimal exponent notation) |
4 | E (Transforms a floating-point number to a decimal exponent notation) |
5 | f (Turns a floating-point number into a decimal representation) |
6 | o (Displays an unsigned integer using an octal representation) |
7 | G (Displays a floating-point number using decimal or decimal exponent notation) |
8 | g (Displays a floating-point number using decimal or decimal exponent notation) |
9 | u (Displays an unsigned integer using a decimal representation) |
10 | s (Writes a string to stdout) |
11 | X (Transforms an unsigned integer to a hexadecimal representation) |
12 | x (Transforms an unsigned integer to a hexadecimal representation) |
13 | % (Prints the % sign) |
14 | n (Returns the number of characters written by far using this call function. It writes the result to the value pointed by the argument) |
15 | p (A pointer that points to the implementation-defined character sequence) |
Sr.No | Flags & Description |
---|---|
1 | - : (within the specified field's width, with the default being right justification (see width sub-specifier)). |
2 | + : (forces the outcome, even for positive numbers, to be preceded by a plus or negative sign (+ or -). Only negative numbers are by default denoted with a -ve symbol). |
3 | (space) : (A blank space is added before the value if no sign is going to be written.) |
4 | #: (Values other than zero are prefixed with 0, 0x, or 0X when used with the o, x, or X specifiers. When used with e, E, and f, it causes a decimal point to appear in the textual output even if no digits would naturally follow. By default, no decimal point is written without subsequent digits. The outcome is the same whether g or G is used. However, trailing zeros are not eliminated.) |
5 | 0 : (when padding is supplied, replace spaces with zeroes (0) on the left (see width sub-specifier)). |
Sr.No | Width & Description |
---|---|
1 | (number) : Minimum characters that must be printed. The output is padded with blank spaces if the value to be printed is less than this amount. Even if the result is bigger, the value is not truncated. |
2 | * : The width is provided as a separate integer value parameter before the argument that has to be formatted, not in the format string itself. |
Sr.no | Length & Description |
---|---|
1 | h : The argument's short int or unsigned short int status is determined (only applies to integer specifiers: i, d, o, u, x, and X). |
2 | I : For integer specifiers I d, o, u, x, and X), the argument is translated as a long int or unsigned long int; for specifiers c and s, it is treated as a wide character or wide-character string. |
3 | L : For floating point specifiers e, E, f, g, and G alone, the argument is read as a long double. |
Return Value of C++ String Formatting Function
The entire number of characters written, except the null character appended at the end of the string, is returned if the operation is successful; otherwise, a negative value is returned.
sprintf Function in C++ stands for "string print". It stores output on char buffers provided in sprintf rather than sending it to the terminal.
Examples
Example 1
A program example to show the sprintf Function in C++
Output
Explanation:
In the above program, we have taken an array of char types of size 100 which will store the final result of the program. After that, we have taken three integer variables named x,y, and z. In the next step, we performed the addition operation, and then we used sprintf(), which will store the result in the buffer, and at the last statement, we have to print the buffer to get the final output.
Complexity
- Time Complexity: O(n)
- Auxiliary Space: O(n)
Example 2
A program example to show the sprintf Function in C++
Output
Explanation
In the above code, first, we imported the math library of C++. Then, we took one char type array and size 100 named mat. After that, we used sprintf() in which we stored the value of pi using mathematical constants (M_PI), which is present in the math library of C++. Then, we have to print the mat showing the final output.
Conclusion
- The term "string formatting" describes arranging particular characters in a string in a particular order and position.
- In the history of C++, printf() family was introduced first.
- Later sprintf Function in C++ was introduced, which does not print the string on the console. Instead, it stores the value in the buffer.
- sprintf Function in C++ stand for string print.
- sprintf Function in C++ can also accept more than two arguments.
- If successful, the sprintf Function in C++ returns the total number of characters written in the string; if not, it returns a negative value.