sprintf() in C
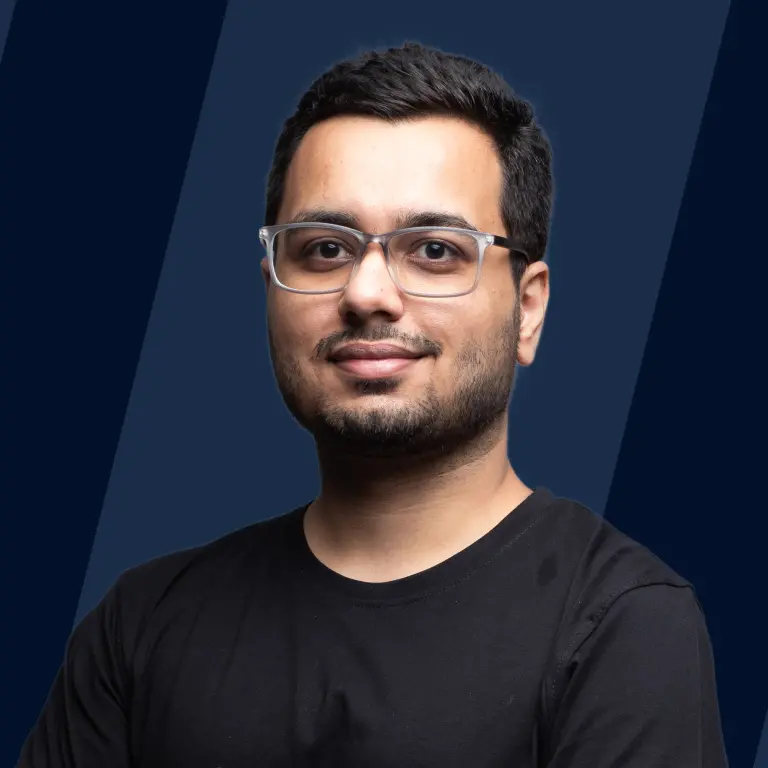
sprintf() in C programming language is a function that stands for “string print”. sprint() function in C is used to store the formatted string in the character buffer which is specified by the user in the sprint() function and provides the functionality to store the string instead of printing it, so that we can print it whenever required also, can perform various function on the buffer string. sprint() in C programming is defined in the <stdio.h> header file, it returns the integer value which indicates the length of the final string buffer after replacing all placeholders with provided values.
Syntax
Syntax of the sprintf() function in C consists of the string buffer, which is nothing but a character array used to store the formatted string after replacing all the placeholders and it also consists of arguments to be placed in the placeholder.
Above mentioned syntax of sprintf in C is similar to the syntax of printf in C with only an extra argument of the str_buffer to store the output.
Parameters
The number of parameters of sprintf() in C depends upon the number of placeholders present in the formatted string but a minimum of two parameters will be present there.
-
str_buffer: This is a pointer to an array of characters where the resulting C string is stored.
-
format: This is a string containing the text to be written to the buffer. It can also include format tags, which are replaced by values specified in additional arguments and formatted as requested. The format tags have the following prototype: [flags][width][.precision][length]specifier. These tags are explained below:
- flags: Flags that control various formatting options.
- width: Specifies the minimum width for the output.
- .precision: Indicates the number of decimal places or characters to be printed.
- length: Specifies the size of the data type.
- specifier: Defines the type of data to be formatted (e.g., %d for integers, %s for strings).
Return Values
If the sprintf() in the C function runs without any error and successfully assigns the value of the formatted string to the string buffer then this function returns the final length of the buffer string excluding the null character at last and if the function call becomes unsuccessful then sprintf() in C will return -1.
Example
Until now we have seen the syntax of sprintf in C, parameters, and the return value of sprintf in C. Let’s take an example to show the working of the sprint() function in C. Code:
Output:
Explanation: In the above code, we have shown the use of the sprintf() function in C. First, we have created the character array or C-type string name buffer of a maximum size of 50. After that, assign it a formatted string that contains the format specifiers which are replaced by the further provided arguments. At last, print the return value of sprintf() function and final buffer string.
Exceptions
sprintf() function in C throws an exception when the size of the final formatted string after all the replacements exceed the size of the buffer string. Let’s see an example to show the above-defined exception case: Code:
Output:
Explanation: In the above code, the length of the formatted string is greater than the length of the buffer string which leads to an error when the sprintf() function tries to assign the buffer the value of the formatted string.
More Examples
Until now we have seen every single detail of the sprintf in C like syntax, parameters, and return value of sprintf in C with uses and detailed explanations.
In this section, we are going to discuss some examples of the sprintf() function to cover it’s all cases.
Example 1
In this example, we will try to add the different types of primitive data type variables to the string buffer.
Code:
Output:
Explanation: In the above code, we have added different types of data types into the character arrays using the appropriate format specifier. Initially, we declared the three-character arrays to contain the value then with the help of sprintf() in C we added string by using the ‘%s’ format specifier, integer value using the ‘%d’ format specifier, float value using ‘%f’ format specifier and similarly double and character value using ‘%lf’ and ‘%c’ format specifiers. At last, print the value of each string.
Example 2
In this example, we will show the case in which the sprintf() function in C returns the negative value.
Code:
Output:
Explanation: In the above code, instead of providing any formatted string, we provided the NULL pointer (it is valid to pass as an argument because the first character of the character array is also a pointer). As we have nothing to assign in the buffer string, this function will return -1, which we have stored in the integer variable 'result' and printed in the last.
Example 3
In this example, we will discuss the benefits of the sprintf() function in C.
Code:
Output:
Explanation: In the above code, we have converted an integer value to the C-type string value which shows this is the simplest method to convert integer, double, float, or character variable to string variable.
Note: C-type strings are just the one-dimensional character array that contains an extra null('\0') character at the end. By including <string.h> header file user can perform various string operations on C-type strings.
Conclusion
- sprintf() in C programming language is a function that stands for “string print” and sprint() in C programming defined in the <stdio.h> header file.
- sprint() function in C is similar to the printf() function but the only difference is it stores the results rather than printing them to the console.
- The first parameter of sprintf() in C is a character array that will store the final string. The second parameter is a formatted string that contains the placeholder. At last, some arguments are present according to the formatted string.
- sprintf() in C returns the final length of the string provided by the user to store the result. Also, the return value excludes the null character present in the end.
- sprintf() function in C throws an exception when the size of the final formatted string after all the replacements exceed the size of the buffer string.
- sprintf() function is the simplest method to convert the integer, float, character, and double value to a string value.