startsWith() in Java
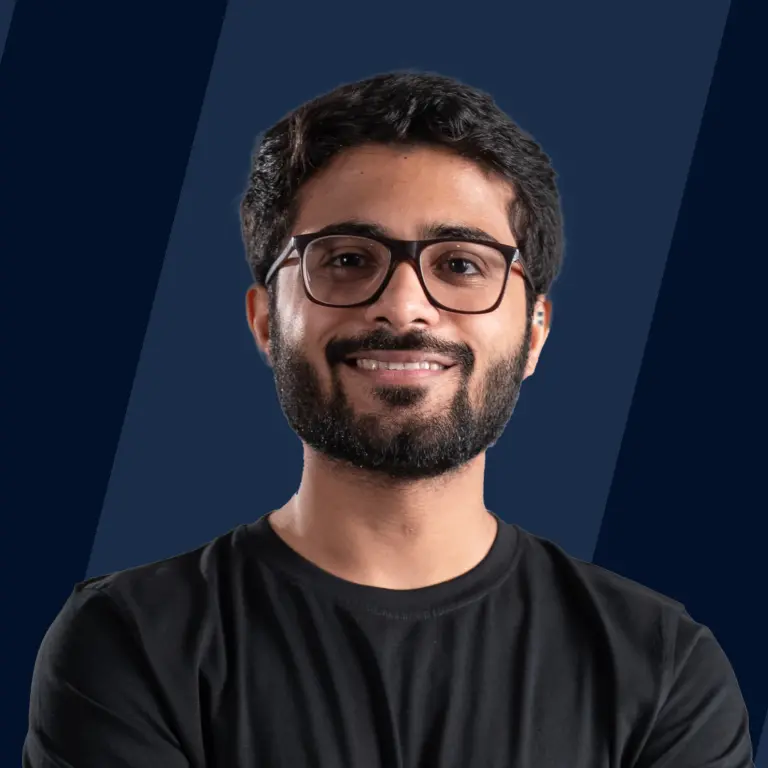
Overview
The startsWith() method in Java is used to check if the string starts with the given prefix. The prefix that we want to check is also in the form of a string which is passed as a parameter to the function. The prefix can be a single character also. If the string starts with the given prefix, it returns true, or else it returns false.
Syntax of startsWith() in Java
The way of writing the syntax for the startsWith in Java is as follows:
The prefix parameter is compulsory. It checks whether this string(on which the function is called) starts with the given prefix.
The offset parameter is optional, and it checks the substring of a string starting from the given index. Therefore, syntax without offset is also syntactically correct:
Parameters of startsWith() in Java
The Java string startsWith method can take in two parameters.
- String prefix (compulsory) - It is used to check whether "this" string(on which the function is called) starts with the given prefix. It is also a string.
- int offset (optional)- It checks the substring of a string starting from the given index. It checks whether the prefix begins from the given index. This is an optional parameter. If not provided, the default value is considered by the Java compiler.
Return value of startsWith() in Java
Return Type : boolean
- Returns true if the string starts with the specified prefix(argument string).
- Returns false if the string does not start with the specified prefix(argument string).
Exceptions of startsWith() in Java
If we pass null as an argument, it will throw the NullPointerException. It is so because null is not allowed as an argument.
Input
Output
Example of startsWith() in Java
Let's discuss a simple example to understand startsWith() method better.
Output
Explantion
For the first output, the given string str starts with the argument string "Jav"; hence method returns true. It is not true for the second case, "java", because the method is case sensitive.
For the third and fourth outputs, offset is passed as the starting index to compare the prefix string with the given string.
We will discuss detailed examples in the 'More Examples' section of the article.
What is startsWith() in Java?
Have you wondered how actually the search results get rendered when we search names and text starting from certain letters?
We can achieve it effortlessly by using the startsWith() method. We can search using the keyword entered by the user by making it a parameter of the startsWith() method.
Let us now discuss the internal implementation for 'startsWith()' in Java. The below code shows how the method is implemented internally inside the library.
The above code helps to compare the string with the prefix (argument string) character by character, thus returning true or false. Note that internal implementation also takes into account for the offset to get the starting index to start the comparison.
More Examples
1) String startsWith(String str)
Output
Explanation
Here the first startsWith() method checks if str starts with "S" and since it starts with "S", it prints true. This can be a use case where we are searching for the contact details of someone.
2) String startsWith(String str, int offset)
Before we proceed towards the example, it is important to know that indexing of strings is done from the 0th index, i.e., the 1st character has index 0, 2nd character has index 1.. and so on. It is described here as the offset parameter of this method revolves around the indexing concept. The offset is the index of the string also known as the start index from where we have to check.
Here in the above image, the indexing is shown for a string.
Example
Output
Explanation
Here the second argument in the startsWith method indicates the offset value or the index where the prefix has to be checked. In the first statement for startsWith() method, we have not mentioned any offset value, so the default offset value becomes 0. In the second startsWith statement, it returns true as in the given string, "ri" is starting from the 2nd index. In the third startsWith statement, since "method" starts from the 7th index(whitespaces are counted as characters), false is printed. In the fourth startsWith statement, it returns true as "va" begins at 19th index.
If we want to check the sender's name or email content in the inbox, we can use this method.
Output
Explanation
Since the string str starts with "Hi", it prints true.
Conclusion
- The startsWith() method is used to check if the string starts with the given prefix.
- It returns a boolean value. If the string starts with the given prefix, it returns true, or else it returns false.
- The Java string startsWith method can take in two parameters.
- String prefix (compulsory)-It is used to check whether "this" string starts with the given prefix. It is also a string.
- int offset (optional)- It checks the substring of a string starting from the given index.
- We use this function to filter parameters like names that start with a particular character or letters.