Static Import in Java
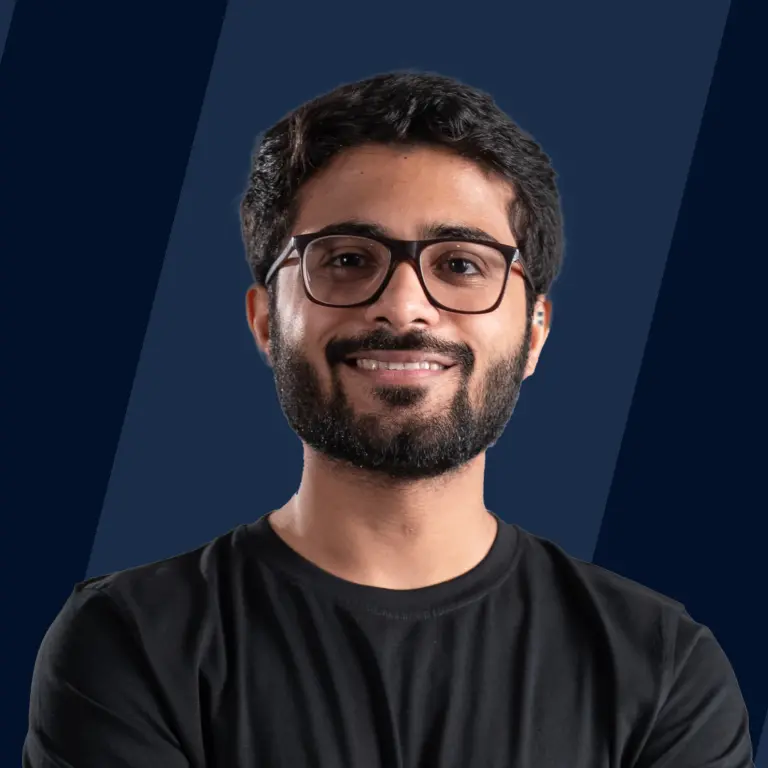
Overview
Java 5.0 introduced static import, also known as the tiger version, as a new language feature. Static variables and static members can now be accessed directly without having to use class names.
We had normal import statements for every java class before static import was introduced; we used class names to call methods and classes. Below is an example of a normal import for the List class.
Static Import in Java
Java's static import feature allows you to import static members of a class and use them since they are declared within the same class. Java 5 introduces static import as well as generics, enums, auto-boxing and unboxing, and variable argument methods. In the minds of many programmers, static import reduces code size and allows you to use static fields from an external class without prefixing the name of the class. By using static import, you can import Integer. MAX_VALUE and refer to it as MAX_VALUE, while without static import you will access the static constant MAX_VALUE of the Integer class as Integer. MAX_VALUE. Static import also allows wildcard * to import all static members of a class, just as it does for regular import statements.
Ambiguity in Static Import
Whenever the compiler encounters two static members of the same name imported from different classes, an error will occur, as the compiler cannot determine which member to use in the absence of class qualifications.
The above code, for example, imports both Integer and Long static members using static imports. As the static members valueOf(String) and MAX_VALUE exist in both classes, there is an ambiguity when using them.
Advantages of Static Import in Java
- Static import is advantageous because it allows you to save keystrokes (e.g.: if you are frequently using System.out.println statements, but dislike manually typing them, you can statically import System.out and System.* and then do out.println() in your code.
- Importing constants as statics simplifies the code and makes it easier to read.
- The static members can be called without using the class name in our code.
- The use of static imports in your code will reduce the amount of code that you need to write when you use members from more than one class
Disadvantage of Static Import in Java
- It makes code harder to read and maintain. Those who did not create the class may get confused if they fail to refer imports at the top, and they may waste time searching for the same member, which is now unqualified.
- The static import mechanism can also cause ambiguity if the same name is used in two different classes.
- Some programmers tend to use an asterik(*) after their static import statements to import all the static members of a class. Doing so significantly reduces the readability of the code.
Difference between Import and Static Import
Import | Static import |
---|---|
With the import feature, the Java programmer can access classes in a package without qualifying as a package | The static import feature allows access to static members of a class without qualifying as a class. |
Imports provide access to classes and interfaces | Static imports provide access to static members of classes |
Import is applied to all types | Static import is applied only to static members |
Example 1: Without Static Imports
Let us look at an example without using static imports
Explanation:
Our above program calculates the square root and tan theta value of variables 1 and 2 and prints them without using the static imports
Output:
Example 2: Using Static Imports
Let us look at an example of using static imports
**Explanation: **
Our above program calculates the square root and tan theta value of variables 1 and 2 and prints them by using the static imports
Output:
When to Use Static Imports?
When you plan on using static variables and methods a lot, you can use static imports. You might want to use static import for example if you want to write code with many mathematical calculations.
Use it sparingly, though! It should be used only when you are tempted to declare local copies of constants or to abuse inheritance. Basically, you should use it when you need to access a static member of a class frequently. By overusing the static import feature, you can end up polluting the namespace of your program with all of the static members you import
Conclusion
- In the code, static import statements should be written as "import static" and not "static import".
- If you explicitly import two static fields with the same name, for example, Long.MAX_VALUE and Integer.MAX_VALUE, Java will throw a compile-time error.
- Static import does not improve readability as expected, since many Java programmers prefer Integer.MAX_VALUE, which indicates which MAX_VALUE is concerned.
- In Java, you can use static import statements on both static fields and static methods.