Static Variable in C
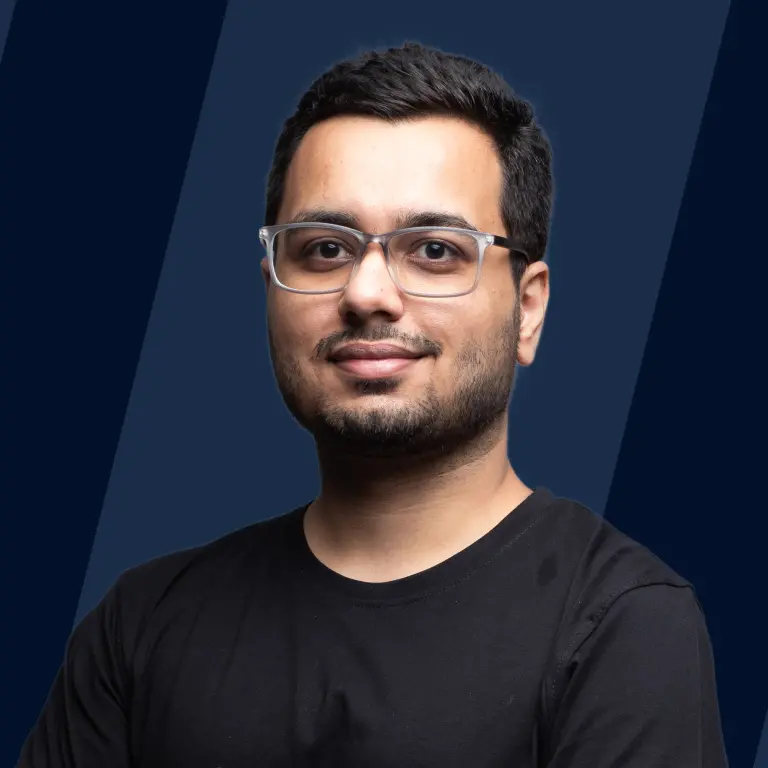
Static variables are the variables which once declared, get destroyed only when the program has completed its execution. They have the property of retaining their previous scope value if they are already declared once in the program. They are different from normal variables because normal variables do not retain their previous value. Normal variables get destroyed once they go out of scope. But when static variables are initialized, they get destroyed only after the whole program gets executed.
Before reading this article, you should have some understanding of the following C Programming topics:
Introduction to Static Variables in C
Consider a scenario where you have two different functions, and you have to separately keep track of the number of calls made to these two functions. Using a normal variable to keep the count of these function calls will not work since at every function call, the count variables will reinitialize their values. This can be understood better by the help of the code given below.
In order to count the function calls separately, we can use static variables since the static variables have a property to retain the value of their previous scope. Given below is the code to print the count of the function calls is given below.
Static variables are the variables that have the property to preserve their value from their previous scope. It means that its value does not get re-initialized every time it is declared. It is different from normal variables because normal variables get destroyed as soon as the function in which it is declared completes its execution. While the memory of normal variables is allocated in the stack segment, the memory of static variables is allocated in the data segment. That is the reason why they are able to preserve the value of their previous scope.
Syntax of Static Variable in C
The syntax for declaring a static variable in the C programming language is given below.
We can also initialize the value of the static variable while declaring it. The syntax for initializing the value of the static variable in C programming language is given below.
Note: The value of a static variable can be reinitialized wherever its scope exists. The below code explains how we can reinitialize the value of a static variable.
Difference between static variables and global variables
Here are some differences between the static and the global variables in the C programming language.
- Static variables can be declared both inside and outside the main function, while global variables are always declared outside the main function.
- To declare a static variable, we have to use the keyword static while in the case of global variable, if the variable is declared outside the main function without any special keyword, the compiler interprets it as a global variable. Below is the code explaining the above statement.
- If we call a function in which a static variable is declared as well as initialized, calling this function again will not reinitialize the value of this static variable again. If this function is called multiple times, the static variable will retain its previous scope value instead of reinitializing its value. This is explained with the help of the code written below. If we want to keep a count of the number of students, we can use a static variable inside a function as given in the code below.
The first function call prints 1, and the second function call prints 2.
But if we have a global variable with the name count declared outside the main function and if we have a function with a variable having the name count, then changing the value of the count inside the function will not alter the value of the global variable count. This is also explained with the help of the code written below.
The output of the first function call is 1, and the output of the second function call is also 1.
Primary uses of a static keyword
Use of static keyword inside the function call
If we want to use a common value of a variable at every function call, we can declare a static variable inside the function. When a static keyword is used inside a function (for example, a static variable is declared and initialized inside a function), it prevents the reinitialization of the variable on multiple function calls. Let us say that a function has a static variable having name as variable_name and its value is initialized with 10. Also, the function increments the value of variable_name on every function call. The function definition is given below.
On calling this function for the first time, the variable_name will have its value as 10 and after incrementation, its value will become 11. So, the function will print 11. Now, if we call this function again, the value of variable_name will not be re-initialized to 10. It will extract its value from its previous scope, which is 11 and after incrementation, it will become 12. So this time, the function will print 12.
Use of static variable to declare a global variable
Static variables can also be declared global. This means that we can have the advantages of a static as well as global variable in a single variable. The difference between a static variable and a global variable lies in their scope. A global variable can be accessed from anywhere inside the program while a static variable only has a block scope. So, the benefit of using a static variable as a global variable is that it can be accessed from anywhere inside the program since it is declared globally. Below is the code which shows how it is declared.
Facts about static variables in C programming language
Here are some facts about the static variables in the C programming language.
Default value of static variable
When we declare a static variable without initializing it with a value, by default, its value is set to 0.
The output for the above code is 0.
Assigning values to static variables
A static variable can only be assigned a constant value. This means that if we try to assign a non-constant value to a static variable, it will give us a compilation error. For example, if we have an integer variable having its name as variable1 having its value equal to 10 and we have a static integer variable having its name as variable2, then the following code will give us a compilation error because variable1 is a variable that does not have a constant value.
The reason for the above compilation error is that static objects needs to be initialized before the execution of the main function. In the above code, the value of the variable variable2 is not known during the compiler's translation time. That is why it is giving a compilation error.
However, the following code will not give any such error since a constant value is assigned to the static variable.
Difference between static global and static local variables
Here are some differences between static global and static local variables in the C programming language.
- Static global variable is always declared outside the main function, while the static local variable is declared inside the main or any block element (for example inside a function, inside a loop etc.). Below is the code explaining the above statement.
- A static global variable can be used anywhere inside the program while a static local variable can only be used inside the scope in which it is declared. Below is the code explaining the above statement.
Properties of static variable
Here are some properties of the static variable in the C programming language.
- A static variable is destroyed only after the whole program gets executed. It does not depend on the scope of the function in which it is declared.
- A static variable has a property to retain its value from its previous scope. This means that its value does not get re-initialized if the function in which it is declared gets called multiple times.
- If no value is assigned to a static variable, by default, it takes the value 0.
- The memory of the static variable is available throughout the program but its scope is only restricted to the block where it is declared. For example, if we declare a static variable inside a function, we cannot access this static variable from outside of this function.
Conclusion
- Static variables are the variables which have the property to preserve their value from their previous scope.
- Static keywords can be used to declare a variable inside a function as well as to declare a global variable.
- If we have not initialized the value of a static variable, by default, it takes the value 0. Also, a static variable can only be assigned a constant value.
- The difference between static variables and global variables lies in their scope. A static variable only has a block scope while a global variable can be accessed from anywhere inside the program.
- The difference between static global variables and static local variables is that a static global variable can be accessed from anywhere inside the program while a static local variable can be accessed only where its scope exists (i.e block scope).
- A static variable gets destroyed only after the whole program gets executed.