stdlib.h in c
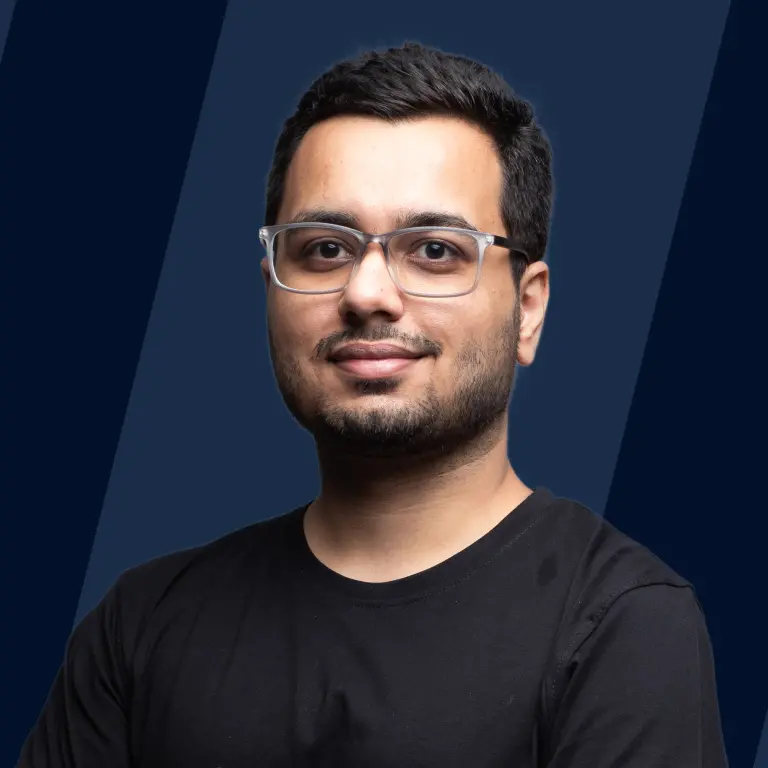
Overview
In C language, stdlib.h defines the standard library utilities. In a typical Linux Operating System, we can find this file at the path /usr/include/stdlib.h. This file defines a variety of functions, data types, and macros.
What is stdlib.h in C?
stdlib.h is a header file and also the Standard Library of C programming language that declares various utility functions for type conversions, memory allocation, algorithms, and other similar use cases. It also has multiple data types and macros defined in the header. In the Linux operating system, this header file is located at: /usr/include/stdlib.h.
This header can be added with the following line at the top of the program.
stdlib.h Library Functions
Function | Description |
---|---|
void *malloc(size_t size) | This function allocates the requested memory on the heap and returns a pointer to it. It is to be noted that, memory is not initialized. |
void *calloc(size_t nmemb, size_t size); | This function is similar to malloc(), but memory is set to zero and then the pointer is returned. |
void *realloc(void *ptr, size_t size); | This function is used to change the size of the memory block. If the new size is less than the old size, the right end is shrunk leaving the left end unchanged. Otherwise, new memory is allocated and it will not be initialized. |
void free(void *ptr); | This function frees the memory allocated by either malloc(), calloc() or realloc(). |
int abs(int j); | This function computes the absolute value of the integer argument j. |
div_t div(int numerator, int denominator); | This function divides numerator with denominator and returns the quotient and remainder in a structure named div_t (discussed below). The quotient is rounded down. |
void abort(void); | This function raises the SIGABRT signal, causing abnormal termination of the calling process unless the SIGABRT signal is caught. |
void exit(int status); | This function causes normal process termination. |
int system(const char *command); | This function creates a child process and executes the command specified by command. |
int atoi(const char *nptr);, long atol(const char *nptr);, double atof(const char *nptr); | This function converts the initial portion of the string pointed to by nptr to int. atol(), atof() functions behave the same, but they convert to long and float respectively. It is noted that these functions do not handle errors. |
double strtod(const char *nptr, char **endptr);, float strtof(const char *nptr, char **endptr);, long double strtold(const char *nptr, char **endptr); | These functions are used to convert the string representation to double, float, and long double datatypes, respectively. Unlike atoi(), proper error handling is included in these functions. |
long strtol(const char *nptr, char **endptr, int base); | Similar to the above functions, this function also converts the string to long, but according to the given base. The base must be between 2 and 36 inclusive, or be the special value 0. |
char *getenv(const char *name); | This function searches the environment list to find the environment variable name, and returns a pointer to the corresponding value string. |
int setenv(const char *name, const char *value, int overwrite); | This function adds the variable name to the environment with the value value, if name does not already exist. If name does exist in the environment, it gets overwritten based on the value of overwrite. |
int putenv(char *string); | Similar to setenv(), this function also adds or changes the value of environment variables. The argument string should be formatted like name=value. setenv() is recommended over this function, due to its flaws. |
void perror(const char *s); | This function prints the string s, to stderr describing the error encountered during a system call or library function. |
int rand(void); | This function returns a pseudo-random number that is in the range [0, RAND_MAX]. |
void qsort(void *base, size_t nmemb, size_t size, int (*compar)(const void *, const void *)); | This function is used to sort an array with nmemb number of elements of size size. The base argument specifies the start of the array. The qsort_r() function behaves the same as qsort() except that the comparison function compar accepts a third argument, enabling us to pass arbitrary arguments. |
stdlib.h C Variables
Datatype | Description |
---|---|
div_t, ldiv_t, lldiv_t | This is the return type of div() function. This contains two integers named quot and rem. |
size_t | This is the return type of the sizeof operator. |
wchar_t | Size of a wide character constant is represented by this datatype. |
stdlib.h Macros
Macro | Description |
---|---|
NULL | This is a special pointer that does not point to any valid data. It is used to |
EXIT_FAILURE | In case of failure, this is the return value for the exit function. |
EXIT_SUCCESS | In case of success, this is the return value for the exit function |
RAND_MAX | This represents the maximum value returned by the rand() function. |
MB_CUR_MAX | There can be no more bytes in a multi-byte character set than MB_LEN_MAX. |
Examples/Usage of stdlib.h in C
Sorting the array
Output
In this example, we will take an array of arbitrary size as user input, sort the array with qsort() and print the array back to the user.
First, we must import the header at the top to use the functions in stdlib.h. We first need to determine the size of the array before allocating the required memory. In the next step, malloc() allocates the memory for n integers.
We must free this memory after use to avoid memory leaks. (or just before the program's termination). We're using atexit() to register the free_arr(), so it gets called just before the program's termination.
If the memory allocation is failed, the perror() function prints the error to stderr stream, and the program is terminated with the EXIT_FAILURE status code.
Otherwise, we prompt the user to enter the elements of arr. qsort() sorts the array according to the given comparator() function. In the end, we iterate over the array and print the (sorted)elements.
The comparator function should return:
- negative value if the first value is less than the second value
- 0 if the first value is equal to the second value
- positive value if the first value is greater than the second value
Just before the program's termination, memory allocated for the arr is freed by the free_arr() function call. The last line in the output confirms that free_arr() is called at the end.
Conclusion
- stdlib in C, declares various utility functions for type conversions, memory allocation, algorithms, and other similar use cases.
- stdlib.h can be imported by adding #include <stdlib.h> at the top of the program.
- stdlib in C, is compatible with cstdlib in C++.
- In Linux Operating System, stdlib.h is located at /usr/include/stdlib.h.