C strcmp()
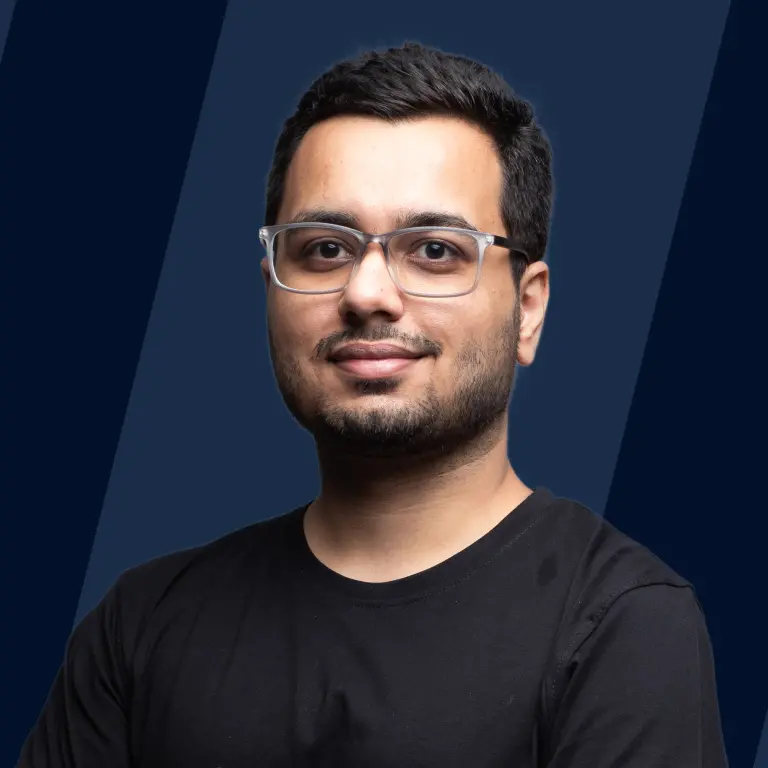
Overview
The strcmp function, a fundamental part of the C language, is used for comparing two character arrays or strings terminated by null value (C-strings) lexicographically. strcmp in C takes two character arrays as parameters and returns an integer value, which signifies whether the strings are equal, or if one is greater or less than the other.
Syntax of strcmp in C
The syntax for using strcmp in C is as follows:
where string1 and string2 are char array variables.
Parameters of C strcmp
The strcmp function in C takes two parameters: string1 and string2, which are pointers to the character arrays that need to be compared.
Return Value of strcmp in C
The strcmp function in C returns an integer value calculated according to the first mismatched character between the two strings. There are three possible return values generated by strcmp:
- Zero (0): The return value is equal to zero if both strings are the same, meaning that elements at the same index in both strings are equivalent.
Example: strcmp in C
Output:
Explanation: In the above code, two strings, string1 and string2, are declared and initialized. They are then passed through the strcmp function. The return value of the function gets stored in the variable rvalue. A conditional statement checks whether the strings are the same by verifying whether rvalue is equal to zero or not.
- Greater than zero (>0): The return value is greater than zero if the ASCII value of the first unmatched character in the left-hand string (string1) is greater than that of the corresponding character in the right-hand string (string2). The result is the difference between the ASCII values of the first unmatched characters, i.e., string1 - string2. Example: strcmp in C
Output:
Explanation: In the above code, the first unmatched character in the strings is found at index 5, where characters in both strings are m and e, respectively. The ASCII values of these characters are 109 and 101, resulting in a difference of 5.
Example
Output:
Explanation: In the above code, two strings string1 and string2 are declared and initialized. They are then passed to a user-defined function, matchStr(). In the matchStr() function, strcmp is called, and the return value gets stored in the variable rvalue. A conditional statement checks whether the strings are the same by verifying whether rvalue is equal to zero.
What is strcmp in C?
The strcmp function is a critical part of the C language, defined and prototyped in the string.h header file. It is used for comparing two character arrays or strings lexicographically. This means that it checks every character at every index in both strings for equality.
The comparison starts from the first character of the strings and continues until an unmatched or NULL character is encountered.
How to Use strcmp in C
In C, strcmp is used to compare two strings. It returns an integer value indicating whether the strings are equal or if one is greater or less than the other. Here's how to use strcmp in C:
- If strcmp returns 0, it means the two strings are equal.
- If strcmp returns a negative value, the first string is lexicographically less than the second.
- If strcmp returns a positive value, the first string is lexicographically greater than the second.
Output:
More Examples of strcmp in C
Example 1: Comparing Two Equal Strings
Output:
Example 2: Comparing a String with a Lexicographically Greater String
Output:
Example 3: Comparing a String with a Lexicographically Lesser String
Output:
Conclusion
- strcmp is a built-in library function of C defined in the string.h header file.
- It takes two character arrays as parameters and returns an integer value that signifies whether the strings are equal or if one is greater or less than the other.
- When strcmp returns 0, it means the strings are equal. A negative value indicates that the first string is lexicographically less than the second, and a positive value means the first string is lexicographically greater than the second.