C strcpy()
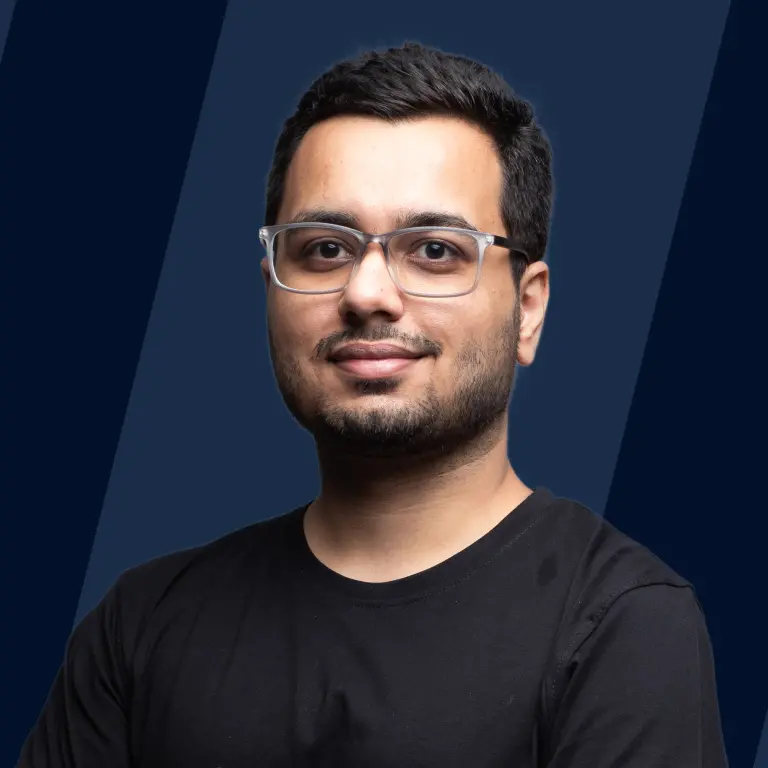
Overview
The strcpy() is a library function available in string library in C, and is used to copy the content of one string to another. The strcpy() function doesn't create a string(character array), it only copies a string(character array).
To perform string copying operation using strcpy() function, we must add <string.h> header file using #include.
#include<string.h>
What is C strcpy()?
The strcpy() is a library function available in string library in C. It is used to copy the character array pointed by the source to the location pointed by the destination. Or in easy terms, it copies the source string(character array) to the destination string(character array).
The strcpy() in C, doesn't perform any comparison of the length of the two strings given. So the length of the destination string(character array) must be greater or equal to the length of the source string(character array).
Suppose in any situation you want to copy the content of one string to another string without deleting the original string. You can use the strcpy() function instead of any looping statements. It will save your time and the complexity of the code.
How to use C strcpy() Function?
To use the strcpy in C, you need to first use header file #include<string.h> and then you will need two strings (one from which the characters will be copied and one on which the characters will be copied).
Syntax of C strcpy()
strcpy ( string2 , string1 ) ;
The above code will copy the content of string1 to string2.
Here, string 1 is the source (original) string from which the content will be copied and string 2 is the destination string where the content of string 1 will be pasted. We cannot change or modify the content of source string.
Internal Working of C strcpy()
The image below explains the working of the strcpy() in C. The content of String1 will be copied to string2.
Initially, string2 is empty and string1 is having content.
After we apply strcpy() in C, the content of string1 starts to be copied to string2.
As every string is terminated with a null character ('\0'), The strcpy() in C, runs a while loop until it finds the null character('\0') and inside the while loop the content of the source string(character array) is copied to the destination string(character array) in character by character. And as the loop finds the null character('\0'), the loop terminates.
As it was mentioned earlier in the article, in strcpy() in C, either the destination string(character array) should have the greater size or both source and destination should have the same size. The strcpy() in C, will not check If the source string(character array) will fit inside the destination string(character array) and it will lead to undefined behavior
Parameter of C strcpy()
The strcpy() function in C takes two parameters:
-
Destination String (char array): This is where the content of the source string will be copied.
-
Source String (char array): This is the original string from which the content will be copied to the destination string.
These parameters should be properly null-terminated character arrays to ensure safe string copying.
Return Values of C strcpy()
-
If you display the return value of the strcpy() function, you will see the value of the destination string (character array) in which the content of the source string (character array) has been copied.
-
The function returns a pointer to the destination string, allowing you to work with the modified destination string.
Conditions for True and False:
-
True: The return value of strcpy() is the same as the address of the destination string if the copy operation is successful.
-
False: If an error occurs during the copy operation (e.g., insufficient memory, buffer overflow), strcpy() can return unexpected results or may lead to undefined behavior. Therefore, the return value should be used cautiously, and it's generally not reliable for error checking.
Exceptions of C strcpy()
potential issues to be aware of when using the strcpy() function in C:
- Buffer Overflow: strcpy() doesn't check for sufficient space in the destination string, so buffer overflows can occur if the source string is too large.
- Null Termination: Both source and destination strings should be properly null-terminated for safe usage.
- Undefined Behavior: If not used properly, strcpy() can lead to undefined behavior, making the program's behavior unpredictable.
- No Error Reporting:strcpy() doesn't provide error codes or exceptions to indicate problems.
To avoid issues, always ensure proper sizing of strings and consider safer alternatives like strncpy() for bounded string copying.
Example
To copy strings using the strcpy() function first use the function name as strcpy, and in parameters, use the two strings, first will be the one on which the content will be copied, and the second will be the original string.
Example:
In this example, we have taken 3 strings(character array) s1,s2,s3. first, we copied s1--> s2 Then for a different example, we created a string(character array) using a double quotation and then copied its value to s3.
The third strcpy() function explains how a strcpy() function can be inserted inside another strcpy() function.
The fourth strcpy() function explains what will be the return value of strcpy(), as we have directly printed it.
In the fifth strcpy() function the content of string s1 is copied to the memory location where the char pointer s4 is pointing.
Output
Points to note: The strcpy() function will not check If the source string(character array) will fit inside the destination string(character array) and it will lead to undefined behavior.
Now the question arises, what will be the output if the source string will have a greater size?? To solve this issue we will use strncpy() function. The syntax of strncpy(): strncpy(destination,source,sizeof(destination));
Conclusion
- The strcpy() function can be used to copy the content of one string(character array) to another.
- <string.h> header file must be included to use strcpy() function.
- The strcpy() function will return the pointer to the destination string.
- The strcpy() function will lead to undefined behavior if the length of the source string(character array) will be greater.
- The strcpy() function can be used in the situation where you want to keep the content of one string(character array) by copying it to another string(character array) and later changing the original string(character array).
See Also: