Strict Mode in JavaScript
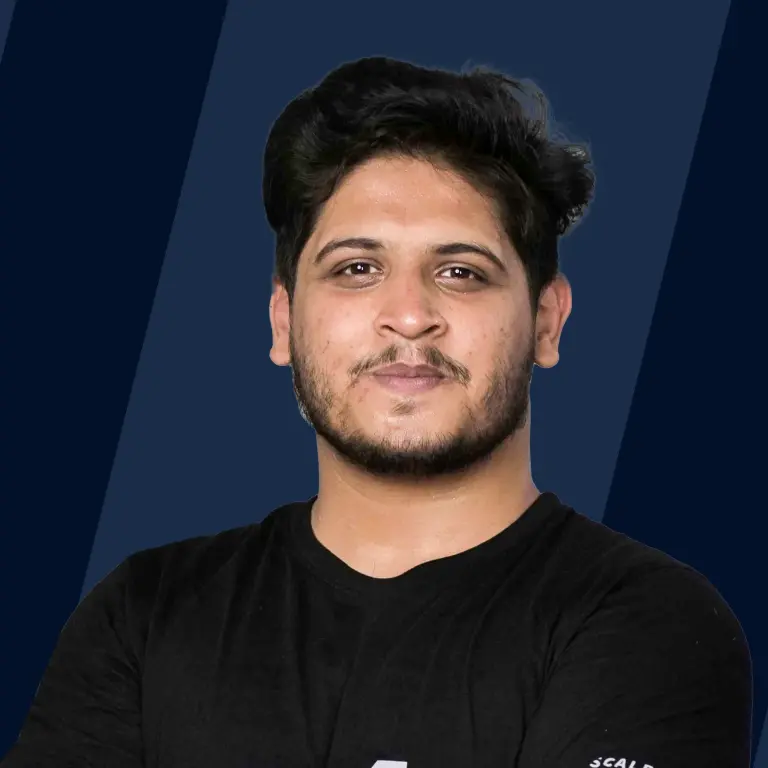
Overview
Strict mode in JavaScript is a powerful tool that helps developers write cleaner and more reliable code by catching common mistakes and preventing the use of problematic features. Strict mode helps detect common coding mistakes by turning them into runtime errors. This feature serves as a safeguard against silent errors that could lead to unexpected behavior in non-strict mode.
What is Strict Mode in JavaScript?
Strict mode is a set of rules and restrictions that can be applied to JavaScript code. It was introduced in ECMAScript 5 (ES5) to help developers write more robust and error-free code. When enabled, strict mode identifies and disallows certain actions and behaviors that would otherwise be silently ignored or executed differently in non-strict mode.
Benefits of Using JavaScript Strict Mode
Error Detection and Prevention:
- Prevents accidental global variable creation by requiring variable declarations with var, let, or const.
Safer Code:
- Disallows the use of problematic features, such as the this keyword referring to the global object, ensuring safer, more predictable code.
Optimized Performance:
- May lead to faster, more efficient code execution.
- Reduces error handling overhead, aiding runtime efficiency.
- Modern JavaScript engines, like V8 (Chrome), SpiderMonkey (Firefox), and Chakra (Microsoft Edge), are optimize for performance in strict mode.
Prevents Silent Failures:
- Catches errors and typos, preventing silent failures.
Enhanced Security:
- Enhances application security by curbing risky operations.
Improved Code Quality:
- Encourages cleaner, maintainable code for higher quality projects.
How to Use Strict Mode in JavaScript
There are two ways to enable strict mode in JavaScript:
- For an entire script
- For a specific function
The differences between both these modes are:
Aspect | Strict Mode for Entire Script | Strict Mode for Specific Function |
---|---|---|
Scope | Entire script. | Only within the function. |
Activation | Enabled with "use strict"; at the script's start. | Enabled with "use strict"; at the function's start. |
Variable Declaration | Requires explicit var, let, or const. | Requires explicit var, let, or const within the function. |
Global Variable Prevention | Prevents global variable creation accidentally. | Doesn't prevent global variable creation outside the function. |
Deleting Variables and Functions | Throws errors when deleting variables or functions. | Throws errors within the function's scope. |
Octal Literal Syntax | Disallows octal literals with a leading zero. | Disallows octal literals within the function. |
this Binding Behavior | May affect this at the script level. | May affect this within the function. |
Impact on Other Functions and Scripts | Affects the entire script and called functions. | Isolates strict mode to specific functions. |
Error Handling and Debugging | Enhances error detection and debugging globally. | Enhances error detection and debugging within the function. |
Flexibility and Selectivity | Applies universally to all script code. | Enables selective strict mode for specific functions. |
Strict Mode For Entire Script in JavaScript
Strict mode can be applied to an entire JavaScript script by adding the "use strict"; statement at the top of the script. It informs the JavaScript engine to interpret the script with a stricter set of rules, catching common coding mistakes and preventing problematic behaviors.
Syntax:
To enable strict mode for the entire script, place "use strict"; at the beginning of your JavaScript file:
Example:
Let's consider an example to understand how strict mode for the entire script works:
Explanation:
- "use strict"; enables strict mode for the entire code.
- Assigning a value to x without declaring it with var, let, or const results in an error in strict mode. This is because strict mode demands variable declarations before use.
Benefits:
- Enables strict mode for the entire script, ensuring all code follows strict rules.
- Reducing the chance of silent failures by catching common mistakes.
Trade-offs:
- May introduce numerous errors in existing code, requiring substantial adjustments.
- While rare, global strict mode may lead to slight performance overhead due to increased error handling.
JavaScript Strict Mode for Functions
Strict mode in JavaScript can also be applied to individual functions by placing the "use strict"; statement at the beginning of a function. This applies strict mode rules exclusively to that function's scope.
Syntax:
To enable strict mode for a specific function, insert use strict; at the beginning of the function:
Example:
Let's explore an example to illustrate the working of strict mode for functions:
Explanation:
- strictFunction is defined with "use strict";, enabling strict mode.
- Variable y is assigned 20 without being declared, violating strict mode rules and triggering an error.
Benefits:
- Provides precise control.
- Facilitates a smooth transition by selectively integrating strict mode, minimizing immediate disruptions.
Trade-offs:
- Strict mode applies only to explicitly enabled functions, potentially leaving other code areas without its benefits.
- Demands developers to pinpoint and designate specific functions for strict mode, introducing some complexity to the codebase.
Examples of Using Strict Mode in JavaScript
Mistyping a Variable Name
Explanation:
In strict mode, mistyping a variable name without proper declaration results in an error. This helps catch common typographical mistakes and prevents the creation of accidental global variables.
Example:
Output:
Variable Without Declaring It
Explanation:
Strict mode requires all variables to be explicitly declared with var, let, or const. Attempting to use a variable without declaring it first will trigger an error.
Example:
Output:
Deleting a Variable (or Object) and a Function
Explanation:
In strict mode, attempting to delete variables, objects, or functions is not allowed. It results in an error, promoting safer coding practices.
Example:
Output:
Duplicating a Parameter Name
Explanation:
Strict mode disallows the use of duplicate parameter names in function declarations, promoting code clarity and preventing potential bugs.
Example:
Output:
Octal Numeric Literals:
Explanation:
Octal numeric literals with a leading zero are not allowed in strict mode to prevent ambiguity and confusion with decimal numbers.
Example:
Output:
Escape Characters
Explanation:
In strict mode, certain escape characters that were previously allowed in non-strict mode are disallowed, improving code consistency and clarity.
Example:
Output:
Writing to a Read-Only or Get-Only Property
Explanation:
Strict mode prevents attempts to write to read-only properties, ensuring the integrity of objects and their properties.
Example:
Output:
Deleting an Undeletable Property
Explanation:
Strict mode prevents attempts to delete undeletable properties, maintaining the stability of objects.
Example:
Output:
Restricted Variable Names
Explanation:
Certain variable names like eval and arguments are reserved and cannot be used as variable names in strict mode, preventing potential conflicts.
Example:
Output:
The with Statement is Not Allowed
Explanation:
The use of the 'with' statement, which can create ambiguity and potential issues, is not allowed in strict mode.
Example:
Output:
Transfering an existing codebase to strict mode
When transitioning to strict mode:
- Gradual Adoption: Enable strict mode in smaller sections to identify and address immediate issues.
- Linting Tool: Use tools like ESLint or JSHint to automatically identify potential issues and enforce strict mode rules.
- Refactor with and this Statements: Remove with statements and explicitly reference objects to avoid potential issues. Being cautious with this as strict mode may affect its behavior.
FAQs
Q: What happens if I enable strict mode in an existing script?
A: Enabling strict mode may unveil new errors. Thorough testing and error-fixing are crucial.
Q: Can I enable strict mode selectively for specific functions?
A: Yes, strict mode can be applied to individual functions, ensuring stricter rules only where needed.
Q: Does strict mode impact JavaScript performance significantly?
A: Strict mode itself doesn't heavily impact performance. However, it aids certain engines in optimizing code, potentially boosting performance.
Q: Are there compatibility concerns with strict mode?
A: Strict mode is supported in modern environments. While compatibility shouldn't be a major worry, it's wise to test in your target environments for assurance.
Conclusion
- Strict mode in JavaScript, introduced in ECMAScript 5 (ES5), enforces stricter rules for improved code quality.
- Enabling it using using "use strict"; catches common mistakes and prevents problematic behaviors.
- Benefits include better error detection, safer coding, potential performance gains, and enhanced code quality.
- Examples include catching typos, requiring variable declarations, disallowing deletions, preventing duplicate parameter names, and banning octal literals.
- It enhances security by prohibiting risky features and encouraging best practices.