String Array in Java
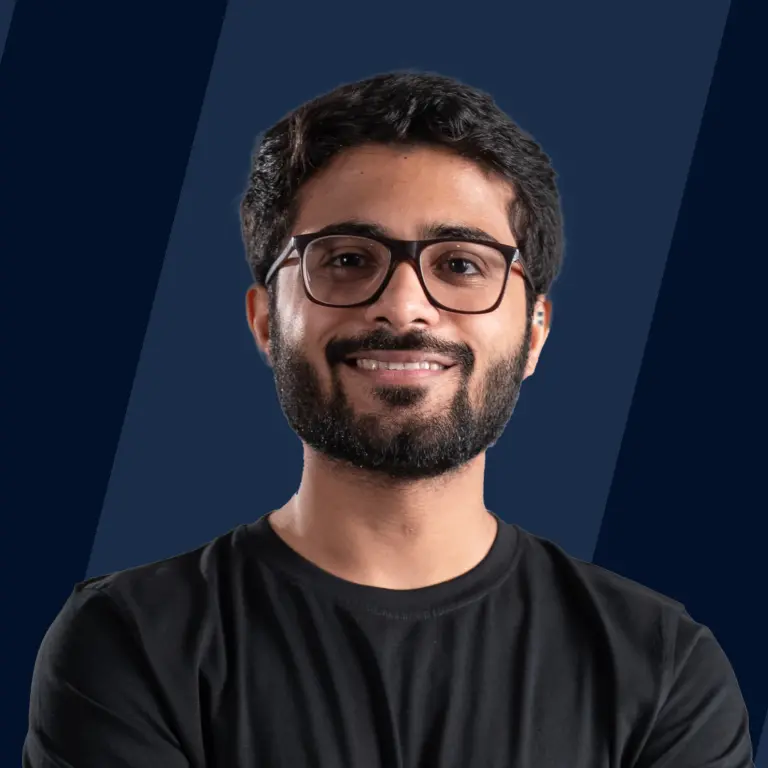
Strings are a sequence of characters. Therefore, we need some sort of structure to store such elements for which we use the string array. A string Array is an array that contains values of string type. Also, the string array stores a fixed number of values.
What is a String Array in Java?
An array is a collection of similar types of values stored at contiguous memory locations, whereas a string stores a sequence of characters. However, a string is considered an immutable object; that is, its values cannot be changed.
Therefore, a string array is an array of fixed elements that contain string type values. Since the strings are immutable objects, string arrays also work similarly.
For example:
The above example shows a string array str, which contains string values.
Declaration
Declaring a variable refers to defining the data type and the variable's name so that the compiler can recognize at which memory location this variable will be stored while using it in a program.
Declaring a variable creates a space in the memory to store the variable's value in that memory location.
You can declare a string array by defining the type and the name of the array. However, the declaration of an array can be as follows: specifying the size of the array.
The string array declaration specifying the size of the array is given below:
The above statement declares a string array of size five where arr is the name of the array and String is the data type for the array.
However, you can also write the above declaration as -
Both of the above declarations can be used. However, out of the three declarations, the first one is more efficient and is suggested for use.
Initialization
Initialization means to put a value into the variable. However, the data types in Java are, by default, initialized by their default values as soon as they are declared. The default value for the string type is null. Therefore, by default, the string array will be initialized by null.
Let us see this with a program:
Output:
As seen above, we have not provided any values to our string array; therefore, they are initialized by their default values.
However, we can initialize the string array in the following ways.
-
Inline Initialization: You can initialize an array in the same line while declaring it as shown below.
Both of the above ways would initialize the same values into the variable arr. Therefore, you can use any of them.
-
Initialization after declaring the array with a specific size: You can initialize the variables using the indexes of an array after its declaration.
Let us see it with an example:
However, in the above method, if you try to initialize more values than the size of the array then it will throw an error stating Java.lang.ArrayIndexOutOfBoundsException exception.
Therefore, the above three methods can be used to initialize a string array.
Iteration
Iteration is a technique in which a block of code is repeatedly executed until a certain specified condition is true. It is done using the for, while, and do-while loops in Java.
However, we use the for and foreach loops in Java to iterate through a string array in Java.
Let us see the code for iterating through a string array using the for loop in Java. The below code basically iterates and prints the elements present in the string array defined:
Output:
Let us see the code for iterating through a string array using the foreach loop in Java:
Output:
Adding Elements to a String Array
We can add the elements in a string array using the following three methods in Java.
- Using Pre-Allocation of the Array
- Using the Array List
- By Creating a New Array
1. Using Pre-Allocation of the Array
In this method, we pre-allocate a size bigger than what we actually need of the array to easily expand it in the near future if needed.
Suppose we have a string array that contains the names of fruits. At a certain point in time, we remember the names of only some of the fruits, so we store them in the string array. However, after some time, when we figure out the other names, we cannot store these names because an array has a fixed size, which is defined while declaring an array.
Therefore, we need to pre-allocate a larger size so that if we need to store elements after the creation of an array, we can do so without any problem.
Let us see an example of the same:
Output:
The time complexity for adding an element in a pre-allocated size array is constant, that is, O(1), whereas the space complexity is the order of N, that is, O(N), where N is the size of the array.
2. Using the Array List
ArrayList is a class of Collections framework in Java that is present in java.util package. It is used to create dynamic arrays. Therefore, any element can be deleted at any time you want. There is no need to pre-allocate the size in an ArrayList as it changes its size based on adding and removing elements.
It can be used as an intermediate data structure to add the elements in a string array. First, the string array is converted to an ArrayList using the asList() method, and then the remaining elements are added to it.
Let us see the code to see the use of ArrayList in adding the elements to a string array:
Output:
The time complexity for adding an element in an ArrayList is constant, O(1), whereas the space complexity is the order of N, O(N).
3. By Creating a New Array
You can also create a new array if the elements cannot be inserted into the first array. This new array can be bigger, and you can copy the contents of the first array and then add the remaining elements after them.
Let us see the code in which we have created a new array:
Output:
Since the elements can be added by accessing the particular index, therefore, its time complexity will be constant, O(1) whereas since we are using an extra array to store the remaining elements therefore, the space complexity will be in the order of N, that is, O(N).
These were the three ways in which we can add the elements into a string array.
Searching in String Array
Searching in an array means finding a particular element among other elements in an array. We use a for loop to search for an element.
Let us understand how can we search in a string array:
In the above code, we are iterating through the elements of the string array and comparing each element with the element that we need to find; if found, print "Element found"; otherwise, print "Not found". The flag variable is used to keep track of whether the element is found or not.
Sorting in String Array
Sorting an array means sorting the array elements in an increasing or decreasing order. Sorting in an array is quite easy. However, certain sorting techniques, like the Bubble sort, Selection Sort, etc., can be used to sort the array elements. We will be using an in-built method sort() in Java for sorting our string array.
Let us see the code for sorting the string array using the sort() function of the Collections framework:
Output:
Therefore, the array elements are sorted in the order in which they will come in a dictionary, i.e., lexicographically.
Convert String Array to String
Sometimes, some operations can't be performed on the string arrays but they can be performed on strings without any problem. Therefore, we prefer to convert the string arrays to the string type in Java.
There are various methods for converting them from an array to a string. Let us discuss them one by one.
1. Using the toString() Method
Java's toString() method takes an array and returns its string representation.
Let us do this with a code:
Output:
The Arrays.toString(arr) converts the string array into a string that can be used for performing various operations.
2. Using StringBuilder.append() Method
StringBuilder is a Java class used to create mutable string objects. They are an alternative to the String class in Java as the String creates immutable string objects, meaning the string cannot change its value.
StringBuilder.append() method is used to append a string to the existing sequence of characters whereas the StringBuilder.toString() is used to return the string representation of the sequence in the StringBuilder object.
Let us see the code to understand it better:
Output:
In the above program, we have created a StringBuilder object. After this, we iterate over the string array and add every element to the StringBuilder object using the append() method.
We then use the toString(), which converts the StringBuilder object to its string representation.
3. Using Join Operation
The join() method in Java is used to return a new String composed of the string array elements joined together with the help of a delimiter.
It is present in the Java String class.
Let us see the code to understand it better:
Output:
In the above program, the delimiter specified is whitespace. Therefore, the join() method adds whitespace in between the string array elements and returns them as a string.
Convert String to the String Array
Sometimes, we need to convert a string to a string array to perform some operations easily. There are two methods of converting a string to a string array: the split() and the regex pattern methods.
Let us see them in detail.
1. Using Split Operation
The split() method in Java is used to split a string based on a particular delimiter specified in the method as an argument.
It separates the string into individual strings; therefore, we can store them directly in a string array.
Let us understand it with the help of code:
Output:
2. Using Regex Pattern
Regular expressions or Regex in Java are used to search for and manipulate strings. They are in Java's java.util.regex package. However, we can also use them to convert a string to a string array in Java.
Let us see it with the help of a code:
The above code compiles the string s using the compile method present in the regex pattern and then splits the string using the split method in Java, after which the individual strings are stored in a string array arr. We have provided whitespace as a delimiter split the string at those points.
Conclusion
- A string array is an array that contains a fixed number of string-type values.
- We can add the elements in a string array in three ways: pre-allocation of the array in which we set the size of the array bigger to add the values afterwards, the second is the creation of a new array, and the other one is to use of the ArrayList class of the Collections Framework in Java.
- We can perform searching in a string array using a for loop in Java
- In Java, we can sort the elements of the string array using the Arrays.sort() method.
- We can also convert a string array to a string and vice-versa.
- There are multiple methods for doing so. The split() and' regex' methods help convert a string to a string array, whereas the join() and toString() methods help convert a string array to a string.