4 Ways to Concatenate Strings in JavaScript
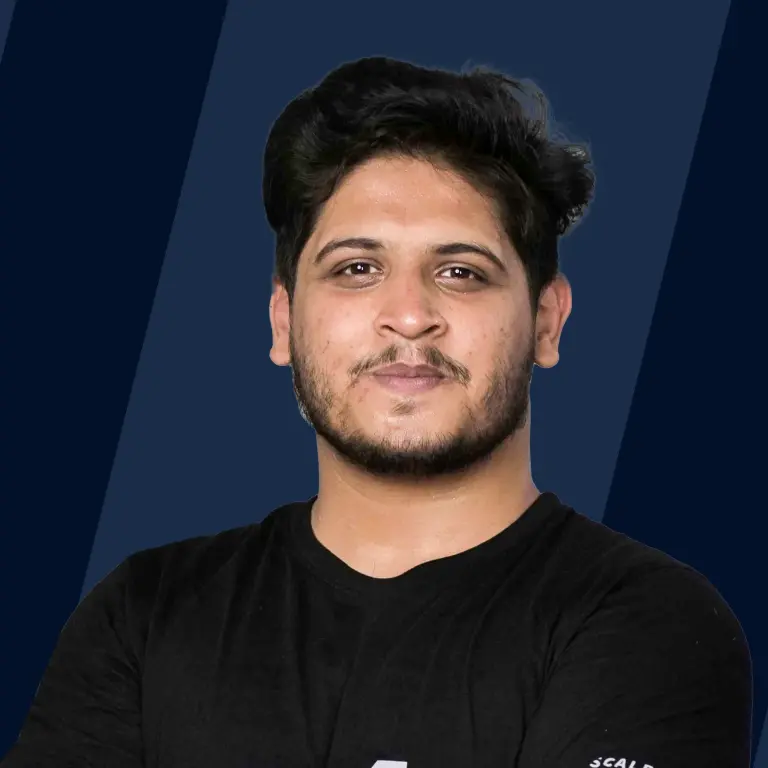
String Concatenation in JavaScript simply means appending one or more strings to the end of another string.
For example,
Concatenating the string "World" at the end of the string "Hello" makes the final string "Hello World". There are 4 methods and ways by which we can concatenate strings in JavaScript:
- Using the concat() method
- Using the '+' operator
- Using the array join() method
- Using template literals
String Concatenation Using concat() Method
String.concat() method accepts a list of strings as parameters and returns a new string after concatenation i.e. combination of all the strings. If the parameters are not strings, the concat() method first converts them into strings, and then concatenation occurs. As we know strings in JavaScript are immutable, so the concat() method doesn't modify the input strings but returns a new string that has all the input strings concatenated.
Syntax for concat() Method
Here, string1 is a variable name for the string on whose end, all the other passed strings will be concatenated. If string1 here has either a null value or it is not a string, then the concat() method will throw TypeError. It necessarily needs to be a string value whereas passed arguments i.e. value1, value2... can be either a string or a non-string as discussed above.
Example 1. (Concatenating Strings)
In this JavaScript example, we have a string temp as "Good Morning". We have passed two more new strings - ", " and "Hava a nice day!" in temp.concat() functional method which will concatenate all the strings and return a new string - message as "Good Morning, Have a nice day!".
Output
The resultant string message after concatenation is displayed as output.
Example 2. (Concatenating an Array of Strings)
Using JavaSript, we can also concatenate items of an array to the end of a string. In this example, we are trying to concatenate items of an array - colors to the end of an empty string using the spread operator in JavaScript (...colors) that unpacks all the items of the array as parameters inside concat() method.
Output
After concatenation of array string items to the end of an empty string, we print the result message as output.
Example 3. (Concatenating Non-String Arguments)
As discussed earlier, we can also pass non-string arguments inside the concat() function that are converted and treated as string parameters. In this JavaScript example, we have passed integer constants - 1,2,3 and a string parameter inside the concat() method that will be concatenated and the returned string will be stored as a result in variable str.
Output
After the conversion of integer constants to string parameters and finally the concatenation, we have printed the result of the str variable as the output.
Example 4. (TypeError)
In this example, we have taken the str variable as a non-string (integer) and we are trying to concatenate the "Hello" string to the end of that non-string variable str. This clearly violates the rules and hence, will generate TypeError in the output console window.
Note: str variable must be of string type for using concat().
Output
TypeError message generated in output console window stating that Integer.concat() function doesn't exists.
Your journey to becoming a certified JavaScript developer starts here. Enroll in our JavaScript Course now and set yourself on the path to a rewarding coding career.
String Concatenation Using '+' Operator
The '+' operator has the same functionality just like we use it to add two numbers, we can also add two or more strings in JavaScript using it. We can either create a new string using the '+' operator or we can use an existing string by appending to the end of it, using the '+=' operator.
Let's understand this concept using some example codes:
Non-Mutative Concatenation ('+' Operator)
In this JavaScript example, we are using '+' operator to simply concatenate the strings. We have initialized three strings at the start and then using '+' operator, we have concatenated them at the end of string - "I am avilable on " and stored the newly generated string in the result variable.
Example 1
Output:
The value of the result variable is printed as output indicating the use-case of the '+' operator.
Mutative Concatenation ('+=' Operator)
Example 2
In this example, we have initialized four strings at the start, and then using the '+=' operator, we are appending strings - Instagram, Twitter, and Facebook to the end of the string result, and the final result string will again be stored in the result variable. This is very useful in case we need to append something to the result string at a later stage, we don't have to write again and again & we can simply use the '+=' operator to append in the already declared result string.
Output:
Example 3
While using concat() functional method, the starting variable (on whose end, we are concatenating) must be of string type for further concatenation but if we use '+' or '+=' operator, then we can concatenate on the end of any object, string or constant variable.
Output:
String Concatenation Using Array join() Method
The join() method of JavaScript is used to concatenate all the elements present in an array by converting them into a single string. These elements are separated by a default separator i.e. comma(,) but we have the flexibility of providing our own customized separator as an argument inside the join() function. One thing to be noted is that this join() functional method has no effect on the original array. Suppose if we need to concatenate any new string or object, we can push that in the already declared array and then use the join() method again.
Syntax for Using join() Method
Here, the separator can be user-customized and by default, if not specified, it uses the (,) comma as a separator. Hence, we get to know that the separator parameter is optional to include.
Let's understand this concept using some example codes:
Example 1
In this JavaScript example, we have initialized an array consisting of some string values. After that, we have used array.join() functional method along with a customized separator - (", ") comma along with space. This will combine all the string values of the array separated by comma and space & the newly generated string will be stored in final_str variable.
Output: The newly returned string after using the join() method will be displayed in the output console where array items are separated by comma and space.
Example 2
Modifying the above example, in case we wish to concatenate another string value at a later stage, then we can simply push it into the array and use join() method again.
Output: Pushing string - "template literals" in the original array and then using the join() method will display the same output as in above Example 1.
String Concatenation Using Template Literals
Before actually understanding the concept of Template Literals, let me draw your attention to the common mistake that we all encounter while concatenating the strings i.e. problem of missing space in string concatenation.
For Example:
Output: In the above example, we haven't put the spaces required between some words to separate them. As a result, our output will look messy and not the desired one!
The concept of string literals helps us to resolve the above issue. Template literals are string literals that allow the use of embedded expressions within the string quoted with the help of back-ticks. Using these template literals, we can also use multi-line strings.
Syntax for Using Template Literals
Here, with the help of $ and curly braces, we can embed a variable in our string quoted under back-ticks, indicating string concatenation :)
Let's discuss this concept using an example code:
Example 1
In this JavaScript example, we have initialized three string variables at the start, and then with the concept of template literals, we have embedded str1, str2, and str3 in the string - final_str quoted under back-ticks. In this way, we can write exactly how we want our string to appear as output and also, it becomes easy to spot the missing spaces between the words.
Output:
Escaping Characters in Strings
When we use special characters in our string, we need to escape them while concatenation to avoid unnecessary bugs.
Let's understand this situation by going through different scenarios:
1. Escaping Single Quotes (' ')
In JavaScript, we can use both single quotes and double quotes while initializing a string. But sometimes, we need to use single quotes within the string literal (Example: I'm writing), and in this case, we must initialize our string with double quotes to avoid confusion for the compiler in interpretation.
Example
Here, we have initialized the result string with single quotes, and also within the string literal, we have single quotes (I'm). This will lead to syntax errors at run-time.
Output:
To resolve the above issue within the JavaScript code, we could have initialized Line 2 within double quotes as:
Alternative Approach:(Using Back-slash)
We can also use the backslash operator to escape single-quote characters. This will generate the same desired output but we prefer not to use it, due to readability issues.
Output:
2.Escaping Double Quotes (" ")
Similar to escaping single quotes, we can use a similar technique for escaping double quotes. This time, we will initialize our string with single quotes to differentiate with the string literal inside, having double quotes.
Example
Output: The output will display "Mayank" as a string word in double quotes. Also, we can use the concept of Back-slash that we have seen above as an alternative here :)
3. Escaping Back Ticks (` `)
Since we use back-ticks for defining template literals, have you ever wondered what if we want to use back-ticks as a symbol within our string? This can be resolved using the back-slash operator.
Let's get clarity about this by seeing an example:
Example
In this example, we have used back-slash operator to display "Good Morning" string quoted within back-ticks on the output console window.
Output:
Supported Browsers
If we talk about browser support then join(), concat(), and '+' operator methods for string concatenation in JavaScript are supported by all modern browsers while Template Literals method of string concatenation is not supported by Internet Explorer. These methods work differently on different browsers and one should choose it depending on the requirements and the purpose.
For example: While using the Google Chrome browser, normal string append is faster than the array join() method but while using the Firefox browser, it is vice-versa.
Conclusion
- String Concatenation in JavaScript simply means appending one or more strings to the end of another string.
- There are four methods in JavaScript for string concatenation: using the concat() method, using the '+' operator, using the array join() method, and using template literals.
- concat() method accepts a list of strings as parameters and returns a new string after concatenation.
- Syntax: string1.concat(value1, value2, ... value_n);
- join() method is used to concatenate all the elements of an array by converting them into a single string. These elements are separated by a default separator i.e. comma(,) but we can also provide our own customized separator as an argument inside the join() function.
- Syntax for using join() method: array.join(separator);
- Template literals are string literals that allow the use of embedded expressions within the string quoted with the help of back-ticks. This helps to reduce the problem of missing spaces between the words.