String to Float in Java
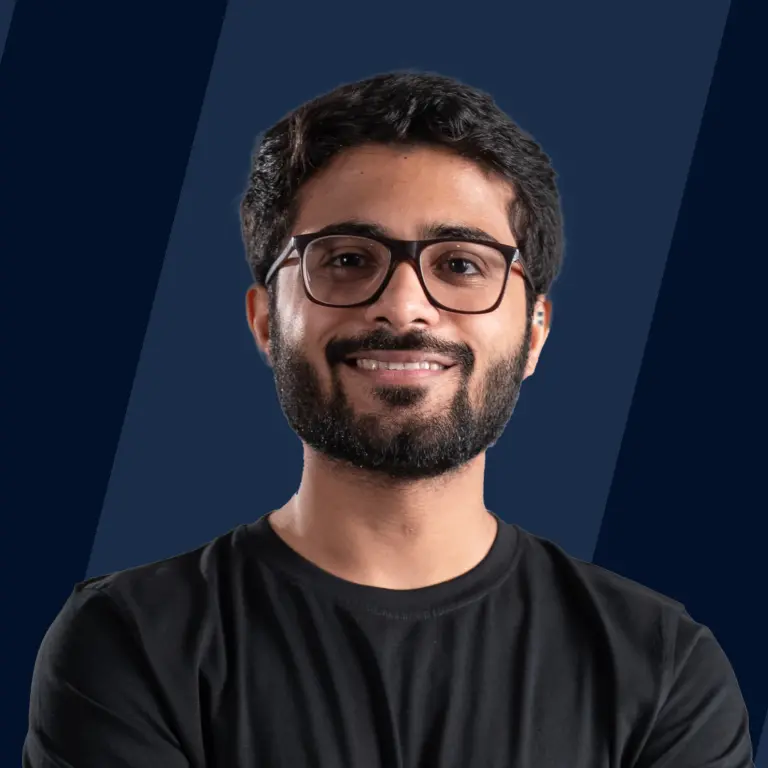
Overview
In Java, we can convert string to floating point numbers using various techniques. The different techniques to convert string to float in java are:
- valueOf() and parseFloat() methods
- Constructors
- DecimalFormat class.
Introduction
We usually convert string to float in Java when we have to perform mathematical operations on string data containing floating-point numbers. The different ways to convert strings to float are:
- valueOf() method
- parseFloat() method
- Constructors
- DecimalFormat class
Let us learn each of these methods with the following examples.
Examples of Java String to float
Example 1: Java String to Float Using Float.valueOf() method
The valueOf() method is a static method in the java.lang.Float class that is used to convert string to float in java. It accepts a string as input and converts it to a float value.
Output of the above program:
Explanation:
In the above example, we used the valueOf() method to convert a string value to a float value.
If the string passed as input to the valueOf() method is not a valid number, the compiler will throw NumberFormatException, and if null is passed as the input, the compiler will throw NullPointerException.
Example 2: Java String to Float Using Float.parseFloat() method
The parseFloat() method is a static method of Float class used to convert string to float in java. It takes the string to be parsed as its input and returns the float type of the string. If the string can not be converted to float, it throws a runtime exception.
Note: In the code below, we have used try-catch block to show the exceptions thrown by parseFloat() method in Java.
Code:
Output of the above program:
Explanation:
- In the above example, we used the parseFloat() method to convert a string value to a float value.
- We tried to convert three different strings into float values using the parseFloat() function. Because parseFloat() was unable to convert null and "five" to float, it generated an exception.
- When input string is "five", NumberFormatException is generated. When null is provided as input, NullPointerException is thrown.
- We used the try-catch statement to catch and print the exception message generated by parseFloat().
Difference Between Float.parseFloat() and Float.valueOf() Methods:
The parseFloat() method returns a float value by parsing a string as input. On the other hand, the valueOf() method returns a float object that is initialized with the value provided as the input.
Example 3: Java String to Float Using Constructor
In the java.lang.Float class, we have a constructor that takes a string value as its argument and creates a Float object of this string.
Output of the above program:
In the above example, we used the Float class constructor to convert a string to a float value.
Example 4: Java String to Float using java.text.DecimalFormat Class
The DecimalFormat class can be used to convert string to floats as well.
Output:
Explanation:
- In the above example, we used the DecimalFormat class to convert string to float.
- We first created an instance df of this class. Then, we parsed the string value into the instance df and used the floatValue() method to convert the string into float.
Conclusion
- Strings can be converted to floating point numbers using different methods in Java.
- The different methods used to convert strings to float are: the valueOf() method, the parseFloat() method, Float class constructor, and the DecimalFormat class.
- The parseFloat() method returns a float value, whereas the valueOf() method returns a Float object.