String to long in Java
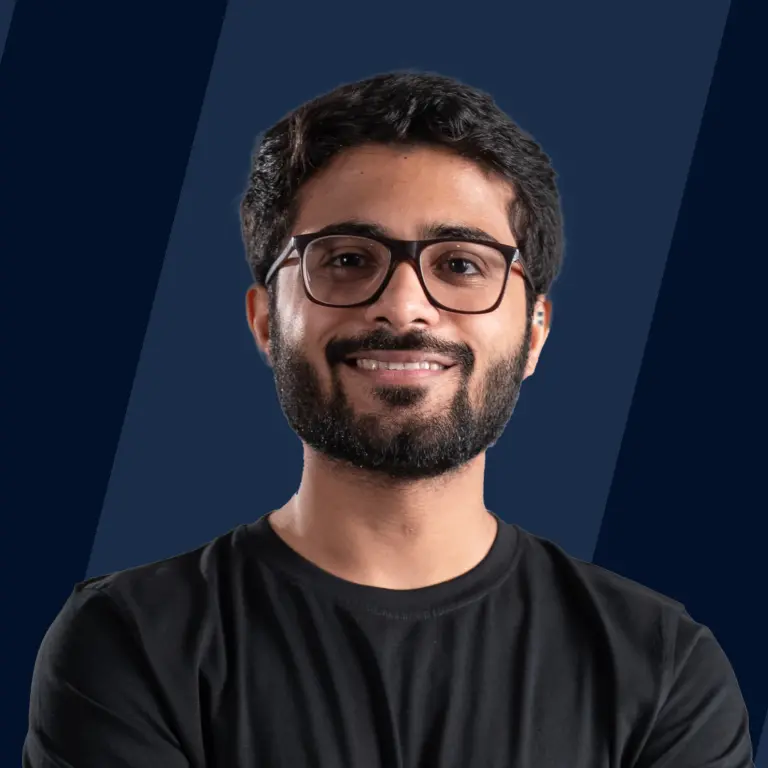
Overview
Generally, the data received from a text field or another file is in the form of a string. In order to perform mathematical operations on the string containing numbers, we need to convert strings to long or int values. This can be done using different in-built methods in Java.
How to Convert string to long in Java?
The long data type is usually used when the numerical values are greater than the range of values provided by int. The default value of a long variable is 0. We can convert a string to long in Java using different methods. In this article, we will learn how to convert using the following methods:
- Using Long.parseLong
- Using Long.valueOf(String)
- Using the constructor of the Long Class
Method 1 - Convert String to long using Long.parseLong()
Long.parseLong() takes a string as its input. All characters in the string must be digits except the first character. The first character could be either a digit or a minus sign ("-"). It does not even allow numbers with decimals in the string.
If the string contains anything except digits (or a minus sign at the beginning), the compiler raises a NumberFormatException.
Syntax:
Here str is a string of digits.
For example:
Output:
In the above example, we used the parseLong() function to convert a string composed of digits into a Long.
Method 2 - Convert String to long using Long.valueOf(String)
The valueOf() method also converts string to Long. Like the parseLong() method, this method also needs a string of digits as its input and it allows a minus sign at the start of the string. If the string contains anything except digits (or a minus sign at the beginning), the compiler raises a NumberFormatException.
Syntax:
For example:
Output:
In the above example, we used the valueOf() method to convert a string of digits to Long.
Method 3 - Convert String to long using the constructor of Long class
The Long class in Java contains a constructor that allows us to convert a string to Long by creating a Long object. The new Long object created by the constructor has the equivalent value of the string containing digits.
The string that is passed as the input, must be composed only of digits and/or a minus sign in the beginning. If the string contains anything else, a NumberFormatException exception will be raised.
For example:
Output:
In the above example, we used a constructor of the Long class to convert a string of digits to a Long value.
Conclusion
- We can convert a string to long in Java using three different methods - Long.parseLong(), Long.valueOf(), and the constructor of the Long class.
- These methods can only convert those strings that contain positive or negative integer numbers.
- If the string that will be converted to long contains anything except digits (or a minus sign at the beginning), the compiler throws a NumberFormatException exception.