String valueOf() in Java
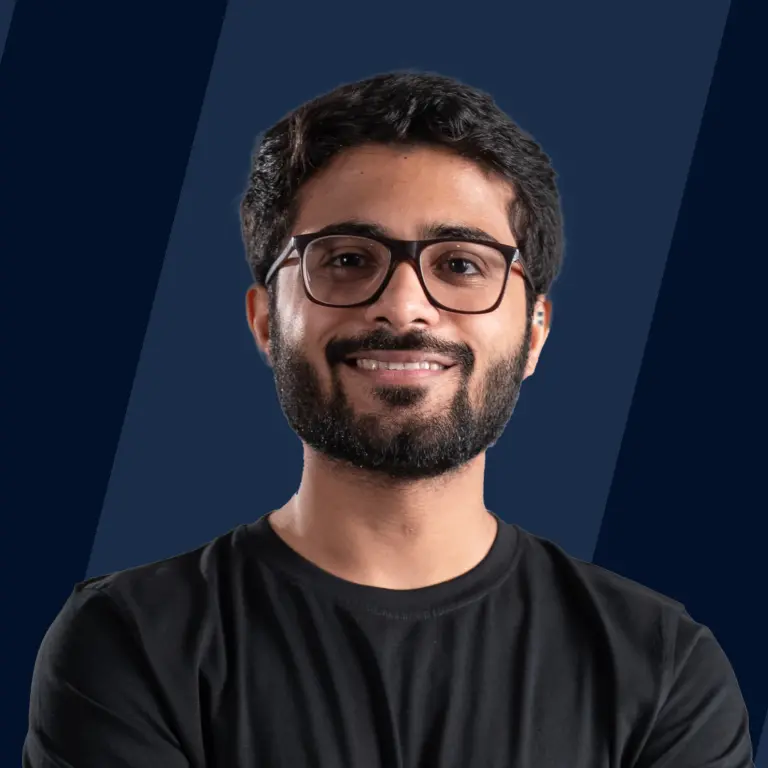
String valueOf() method is present in the String class of java.lang package. valueOf() in Java is used to convert any non-String variable or Object such as int, double, char, and others to a newly created String object. It returns the string representation of the argument passed.
Syntax of valueOf() in Java
The following two signatures are possible:
The first signature takes in a data type like int and returns a String object. The other possible data types of T are explained in the parameters section.
The second signature takes in a character array and two other integers, which we will discuss in the parameters section.
The syntax of valueOf() is as follows:
Parameters of valueOf() in Java
As per the first method signature, T can be:
- boolean
- int
- long
- float
- double
- char
- char[]
- Object
For the second method-signature parameters are char array, offset and count.
- offset is the starting index in the case of a char array
- count is the number of characters to be considered in the sub-array starting with offset
Return Values of valueOf() in Java
The return type is a String class object.
Exceptions of valueOf() in Java
The only exception valueOf() can throw is IndexOutOfBoundsException in the second signature if:
- offset is negative
- count is negative
- count + offset is greater than the length of the char array
If, instead of int, some other data type is passed, or the number of parameters is less or more than expected, we will get a compile-time error.
Example of valueof() in Java
Output:
What is valueOf() in Java?
String valueOf() is very useful in cases where we want to concatenate non-string variables.
For example, consider a function that gives us the version of a file in x.y format where x is the major version and y is the minor version.
Imagine a Version class as follows:
We can use the valueOf() operator to get the concatenated version as follows:
Output:
How does valueOf() work?
valueOf() works by invoking the toString() method of the respective data type passed to valueOf().
Thus, any object can be passed to valueOf(), and we would not get any error, as internally, the toString() method would be invoked on that object.
Note: The toString() method is declared in the Object class, and every class in Java inherits the Object class.
Example 1: Java String valueOf() for Numbers
Output:
Example 2: Convert Char Array to String
To understand the conversion, let us look at the example given below. Here, a character array is converted to a string.
Output:
Example 3: Convert Char Subarray to String
To convert the subarray of the char array, we use the second signature of valueOf, which takes in an offset and length.
Output:
Example 4: Convert List Object to String
We can use valueOf to convert a list of strings to a single string.
Output:
Conclusion
- valueOf() is a static method present in the String class.
- It is used to convert any data type, such as int, double, char, or char array, to its string representation by creating a new String object.
- We can also use valueOf() to convert a char array or a subarray of a char array to a String.
- Return type of valueOf() is String.