Strings Concatenation in C
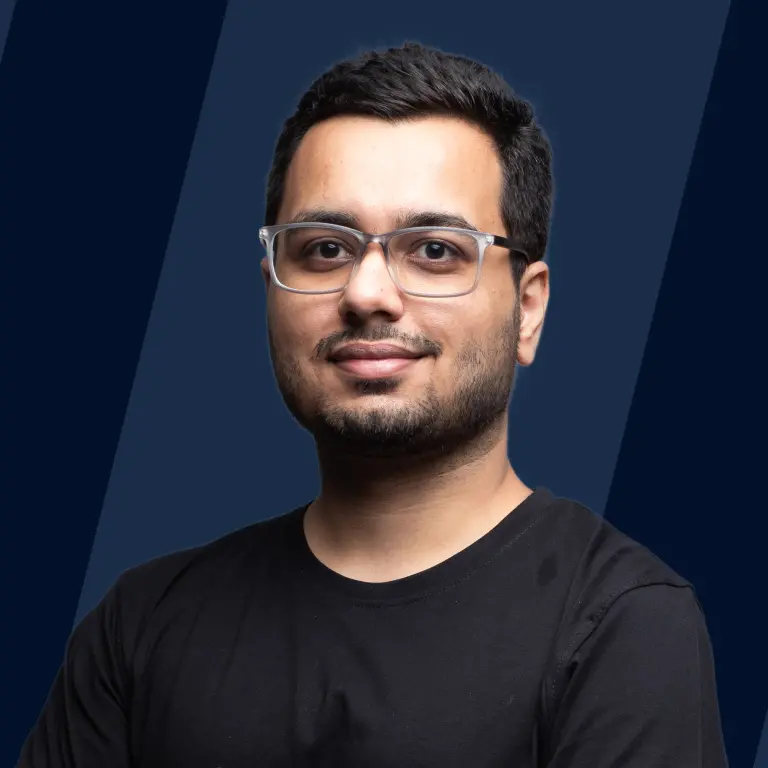
Overview
String concatenation in C can be simply defined as the addition of two strings or updating them. In C language we can add two or more strings end to end or can update them using the concatenation process. The concatenation helps to combine two or more strings together and provide them as a single string value. Suppose we have two strings, the first one being Scaler and the second one - academy and we want to combine these two strings as a single string, so we use String Concatenation and the result will be: Scaleracademy.
How to Append One String to the End of Another
Strings and Character arrays can be used interchangeably. Before going to methods of string concatenation, let us take a brief look over what does strings and concatenation means in c language.
String: String can be defined as the one-dimensional array of characters that terminates by a null ('\0') character. It is used to store and manipulate texts and sentences. Strings are sequences of characters that are enclosed in double quotation marks and it automatically appends a null character \0 at the end by default. You can initialize strings in the following ways:
Now, let us discuss Concatenation.
Concatenation: The term concatenation refers to the merging of two things together. And this merging of two things will together form a new result. In computer programming concatenation also does the same thing. It merges two things end to end or we can say that the second thing is added up to the first thing and provides a new result.
Now, let's learn about the various ways that are there for string concatenation in C language. There are various ways in which we can concatenate strings in the C language. All the methods are described below with suitable examples:
Concatenate Two Strings Using Loop
Let us first learn how we can concatenate two strings using for loop by taking an example.
Example:
Output:
Explanation In the above example, we have described how to concatenate two strings using a loop. Two strings are declared named name1 and name2 and their values are taken by the user as input. Then we concatenated these two values and stored them in the first string named name1.
A loop is initialized and the loop will start from i=0 until the null character of name1 character array meets. After execution of the loop, the value of 'i' will be one more than the actual length of the string. Again a new loop will be initialized and one character is added in every iteration, and iteration will continue until the null character of name2 meets. In this way, the concatenation of strings using a loop will be executed.
Concatenate Two Strings Using Pointer
We store the address of two strings in pointers. Using the loop, we reach the end of the first string (null character), and after that, we append the characters of the second string at the end of this first string one by one. Let us now take an example and see how we can concatenate two strings using pointer.
Output:
Explanation: In the above example, two variables of the character array are declared namely first_string and second_string. The user's input is stored as a value of these variable strings. Then, two char pointers str1 and str2 are declared. The address of first_string and second_string will be stored in str1 and str2 respectively and a loop will continue to execute until the null character of first_string meets. Then, the pointer will point at the location that is just after the last character of the string. Again a new loop will continue to execute until the last character of second_string meets. After every iteration, the character stored at **j**th location of second_string is appended to **i**th location of first_string. In this way concatenation of strings can be done using pointers in C language.
Concatenate Two Strings Using Strcat() Function
strcat() function is the easiest way to concatenate two strings in C language. This function takes two arguments for work. The first one is 'destination string' and the second is 'source string'. The two strings will be concatenated and will be stored in the destination string as a result.
Syntax:
The strcat() function concatenates the string_1 and the string_2, and the result is stored in the string_1.
Output:
Explanation: In the above example, two character arrays are declared. The values of strings are taken as input from the user and stored in 'string_1' and 'string_2' respectively. Then, the strcat() function is used to combine the values of string_1 and string_2, and the result is stored in the destination string. We will get the concatenated string as output.
NOTE: It is important to keep in mind that the length of the destination string should be enough to store all the characters of a concatenated string. Otherwise, the output will be unexpected.
Concatenate Two Strings without Using Strcat()
Although there are many methods to concatenate strings we can also concatenate two strings manually without using any function or method. In it, values taken by the user are stored in string_1 and string_2 and a resultant string is taken. First, the string_1 is inserted into the resultant string and then the string_2 is inserted into the resultant string. Let us see an example.
Output:
Explanation: In the above example, we can see how the concatenation of strings can be done without using strcat() function. The value of strings is taken as input from the user and stored in 'string1' and 'string2'. The value of the first string is inserted into a new string and the value of the second string is inserted into a new string. The loop checks whether string1[i] does not equal to '\0' or not, and increments the value of i by 1. A while loop is used after this which checks whether string[i] is not equal to '\0', if the condition becomes true, the loop will get executed. And then printf is used to print the concatenated strings as output.
Conclusion
- Strings are a two-dimensional array that stores text and can be manipulated as per need. String concatenation in C language is defined as the process of appending two strings end to end and producing a new string.
- In string concatenation using a loop, we iterate over the first string using a loop until it reaches the null character of the first string. Now, we insert the characters of the second string one by one at the end of the first string.
- In string concatenation using pointers, we declare two pointers that will store the address of these strings. Loops are executed until they reached the null character of the first strings and we insert the characters of the second string one by one at the end of the first string.
- We can also append two strings using strcat() function. This function simply adds the values provided by the user as a string and shows a new result.
- We can also concatenate two strings without using strcat() function but you have to include 'stdio header file using include.