StringTokenizer in Java
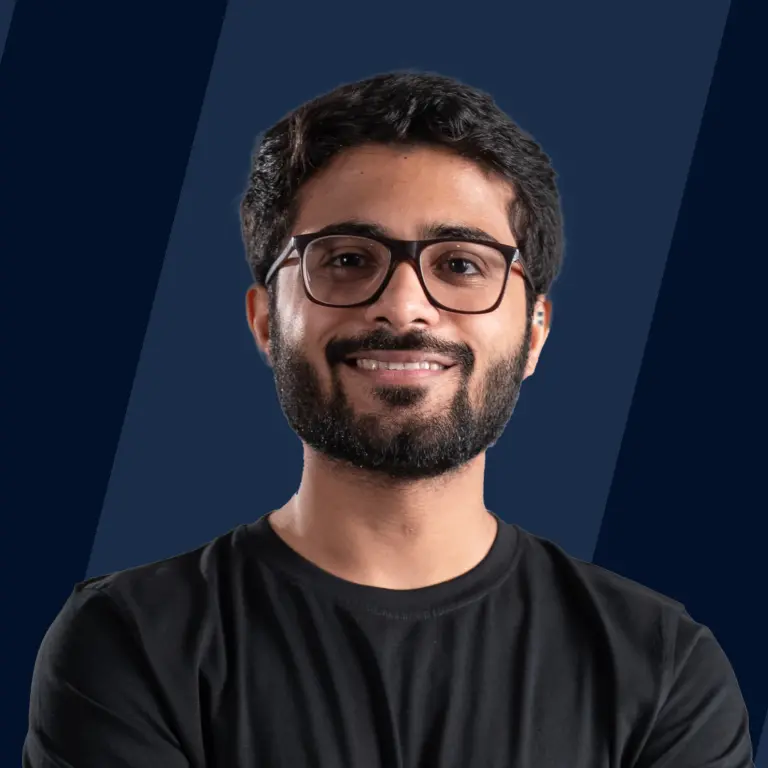
Overview
A string can be divided into tokens(Java tokens are the text (words) that are produced when the Java compiler splits a line of code. The Java program's simplest component is this.) using StringTokenizer in Java.
Internally, a StringTokenizer object keeps track of where it is in the string that has to be tokenized. Some procedures move this place ahead of the currently processed characters. By extracting a substring from the string that was used to generate the StringTokenizer object, a token is returned. It offers the initial stage of the parsing procedure, often known as the lexer or scanner.
The StringTokenizer in Java enables applications to tokenize strings. The Enumeration interface is implemented by it. Data parsing is done using this class. We must specify an input string and a string with delimiters in order to use the String Tokenizer class. Tokens are divided by delimiters, which are characters. The delimiter string's characters are all accepted as delimiters. New line, space, tab, and whitespace are the default delimiters.
Introduction to Stringtokenizer in Java
The java.util package has a class called StringTokenizer that is used to tokenize strings.
To put it another way, we can break a sentence up into its words and conduct several operations, such as counting the amount of tokens or splitting a sentence up into tokens.
This StringTokenizer includes constructors and methods that allow us to separate a sentence into tokens. StringTokenizer, tokenize the string on the basis of the delimiters provided to the String tokenizer class object.
Whitespaces, tab, newlines, carriage returns, and form feeds are considered common delimiters.
These delimiters are used by default, however users can define their own delimiters by including them as arguments in the parameter.
Constructors of the StringTokenizer Class
Three different function types are commonly described by StringTokenizer:
- StringTokenizer(String str) Tokenization of a certain string that is supplied in the parameter is accomplished by this constructor.
All of the standard delimiters specified in the StringTokenizer class description are taken by constructor.
WhiteSpaces, newlines, tabs, carriage returns ("r"), line feeds ("n"), and form feeds ("f") are the delimiters.
2.StringTokenizer(String str, String delimiter) Based on the delimiter the user specifies in the input, this constructor implements string tokenization.
3.StringTokenizer(String str, String delimiter, boolean flag) This constructor has the ability to display the delimiter in addition to doing string tokenization based on the delimiter.
Methods of the StringTokenizer Class
Method | Description |
---|---|
boolean hasMoreTokens() | Return True if more token are available else return False. |
String nextToken() | Return next token of StringTokenizer Object |
String nextToken(String delim) | Return Next token based on delimeter. |
boolean hasMoreElements() | Same as hasMoreTokens() |
Object nextElement() | same as nextToken() but its return Object |
int countTokens() | count Total number of Token |
1. int countTokens()
The countToken() method returns the number of tokens in the input string that are separated by any white space.
The number of tokens in the String can be determined using this approach, and we can easily process the entire string by using this number of tokens as a loop parameter.
2. nextToken()
The StringTokenizer class's nextToken() method returns the next token as a String. The first time we use it, it returns the following token as the string's first token.
3. String nextToken(String delimiter)
The only difference between the nextToken(String delimiter) and nextToken() methods is that the former returns the next token based on the delimiter we pass as an argument to the latter.
Any delimiter, including a symbol, a number, or a character, is acceptable.
4. boolean hasMoreTokens()
This method merely determines if the String contains any additional tokens. If a token is available, it returns true; otherwise, it returns false.
It can be used in the while loop to process the entire string up until there are no more tokens left.
5. Object nextElement()
The nextElement() method is comparable to the StringTokenizer class's nextToken() method;
The distinction is that, as opposed to the nextToken() method, which returns a String, it returns the value in the form of an Object.
Java Program to Illustrate StringTokenizer Class
Example1: In Example Below we are printing each tokens of StringTokenizer st.
Output:
Code Explanation
In Above code String tokenizer Object is created by passing string to Constructor.Each Token/Word is printed is using while loop.
Example2: In this code we are going to use String Tokenizer class to create Tokens with delimeter "," and print each tokens.
Output:
Code Explanation
In Above code as Tokens are created on based of delimeter passed in constructor(,) so 2 tokens are created.Each token is printed using while loop.
Example3: In this example we are going to create StringTokenizer Object and print each of Tokens with delimeter.
Output:
Code Explanation
In Above Code there is string,delimeter and Boolean parameter are passed.This boolean parameter(True) ensure that delimeter is printed.If False is passed then delimeter will not be printed.
StringTokenizer in a Real Use Case
Let's try utilising StringTokenizer in a practical scenario now.
In some cases, we attempt to read data from CSV files and parse it using the user-specified delimiter.
StringTokenizer makes it simple to reach the following:
Example: In this example we are going to read a csv file and convert it into StrinTokenizer Object and print each token of each line.
Output:
Code Explanation
In Above code We have creted a Object of StringBuffer class by passing example.csv file then read each line of csv using br.readline() and printed each token of each line using while loop.
Conclusion
- The java.util package has a class called StringTokenizer that is used to tokenize strings.
- we can break a sentence up into its words and conduct several operations, such as counting the amount of tokens or splitting a sentence up into tokens using StrinTokenizer in java
- StrinTokenizer in java provides different constructor by which we can pass string,delimeter and if wants to return delimeter or not.
- StrinTokenizer in java provides different methods as well by which we can perform different action on StringTokenizer Object.