strncpy() Function in C
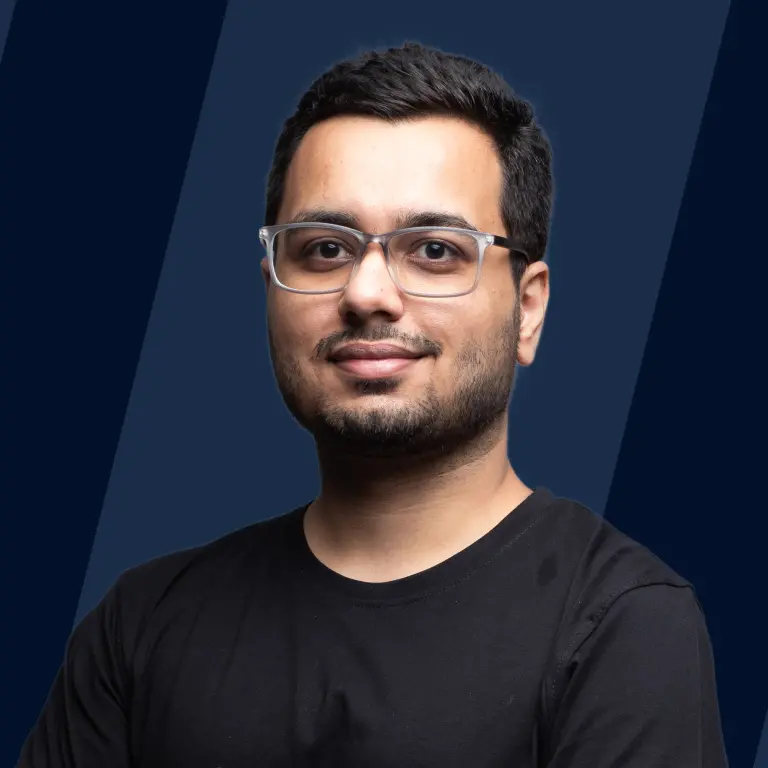
Overview
The strncpy function in C is used to copy the specified number of characters from one string to another string contiguously. The function will not modify the source string from which the value is copied.
Syntax of strncpy() Function in C
The header string.h must be included to use the library in which the strncpy function is present.
The syntax of the strncpy() function according to C99 standards is,
The syntax of the strncpy() function before the introduction of C99 standards is,
Parameters of strncpy() Function in C
There are three parameters for the strncpy function in C,
- The destination specifies a pointer that contains the address of the first character of the character array to which the string is to be copied.
- The source specifies a pointer that contains the address of the first character of the character array from which the string is to be copied.
- The size denotes the number of characters that should be copied from source to destination.
The data types and keywords used in the syntax are,
- The const keyword is used to specify a constant variable. Once a value is assigned to a variable of type const, we can't modify or reassign that value.
- The size_t is a data type used to store the size of data types. It can only store positive integer values and is of type unsigned int.
- The restrict keyword is used to define the address stored by the pointer as an address unique to that pointer alone. It helps to solve the problem of memory overlapping.
Return Value of strncpy() Function in C
The strncpy() function returns a character pointer(char*) which stores the address of the first character in the destination character array.
This character pointer can be used to access the whole string as data are stored in a consecutive memory location in an array. We can get the second character in that string by incrementing the character pointer by 1.
Example
The following example illustrates the working of strncpy(),
In the above example, a character array, source is copied to another character array, destination.
The output for the above example is,
The number of characters to be copied can be given in three ways,
- Using the strlen() function. The strlen function returns the length of the string in the character array passed as a parameter.
We add 1 to the results of the strlen function because the function doesn't include the /0 or null character.
The null character marks the end of characters in the character array and is represented by /0. There are 12 characters in the string strncpy in C, but the size of the character array will be 13 including the /0 character.
- Using the sizeof() operator. The sizeof function returns the sizeof the data type or data variable passed as a parameter. In our example, the sizeof operator will return 13 and will include the size for the /0 character.
- We can also manually enter the length of the source string+1. This 1 is for the null character.
The following code shows a comparison between strlen and sizeof methods,
Note: The %zu is the access modifier used to represent data of type size_t.
Exceptions of strncpy() Function in C
There is a possibility of buffer overflow when using the strncpy function. This occurs when the specified size parameter is larger than the size of the destination character array or when a size is a negative number. Consider the following example,
The output for the above example is,
The problem here is that the size of the string to be copied to the destination character array is too large than the size of that array.
This causes buffer overflow as the function strncpy only copies directly to the memory and does not know about the size of the array. Therefore, it completely overwrites the contents of the memory.
- The printf() function will print the characters until the null character, \0 is reached.
Since the only null character is present at the end of the string Buffer overflow, the complete string is printed even though the size of the string is greater than the size of the array.
The above example also has another character array, buffer declared just next to the destination character array. The C compiler performs sequential memory allocation for the character array as per the diagram given below,
So after the space required for the character array, destination is allocated; the space for the character array, buffer is allocated just next to the space allocated for the destination array.
Due to this, when the strncpy function overwrites the first 5 spaces in the destination character array, it will move into the next address which denotes the buffer character array. Due to this, the characters after the first 5 characters are overwritten in the buffer array.
This is dangerous as this can easily overwrite confidential information or can be used to store malicious code inside the memory.
To prevent this we can use the alternative function for strncpy called strncpy_s. The syntax of this function is,
This function prevents buffer overflow by adding an extra parameter size_of_destination which denotes the maximum size of the destination memory. If the size exceeds the size_of_destination, an error number is returned and the program stops execution. The data type errno_t is used to denote the error number returned. When data is copied successfully, the value 0 is returned.
What is strncpy() Function in C?
- The function strncpy() is used to copy a specified length of characters from a source character array to a destination character array.
- Pointers are used to store the address of destination and source character arrays.
- The function returns a pointer storing the address of the first data in the destination character array.
More Examples
We can partially copy an array to another array. This is similar to taking a substring from a string. The following code illustrates this,
The strncpy function in the above example, only copies the first 7 characters from the character array, source, and places it in the destination array.
It is important to assign the last character of the destination array to /0 so that no garbage values will be printed when using the printf() function.
The output will be,
We can also concatenate two strings into a single string using the strncpy function. The following code illustrates this,
In the above example, the first strncpy function copies all the characters of source1 excluding the null character to the memory location of destination.
The second strncpy function, then copies all the characters including the null character to the memory location next to where the previous data was copied. This memory location is reached by adding the length of source1 to starting address location represented by destination.
The output for the above example will be,
Conclusion
- The function strncpy is used to copy the specified number of characters from one string to another string.
- This function can cause undefined behavior when the specified size is greater than the space of the destination array.
- We can perform functions like concatenating two strings and finding the substring using the strncpy function.