Strong Number In C
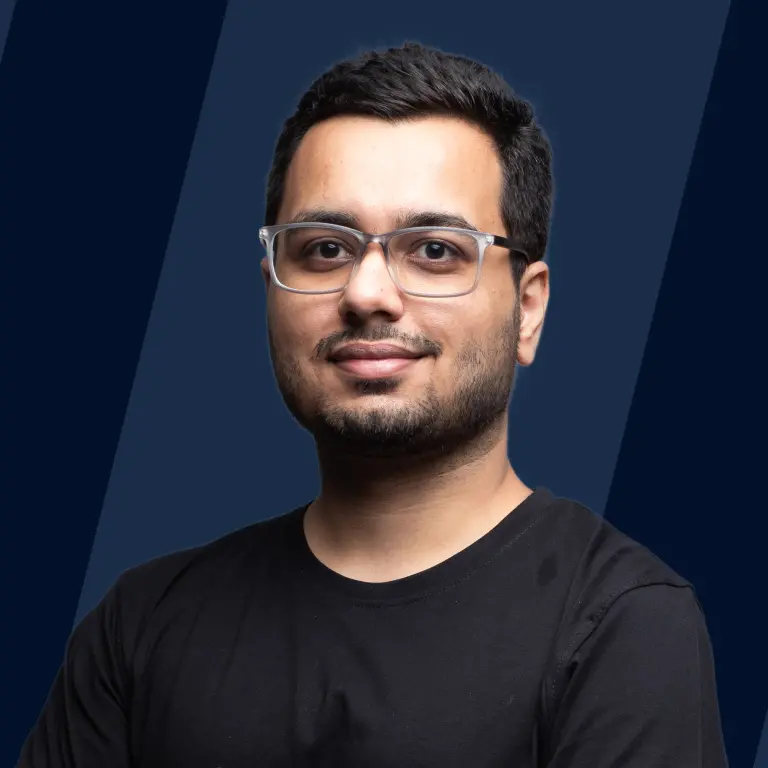
Overview
In this article we are going to know about what is strong number in C programming language, So strong number in C is defined as when the factorial of individual number sums to the original number then it is known as a Strong number.
What is a Strong Number in C?
In C, a Strong number is defined as when the factorial of each digit of a number is equal to the sum of the original number.
Example 145 is a strong number.
- First compute the factorial of each digit of the number
- 1! + 4! + 5! = 1 + 24 + 120 = 145
- Since the number computer and the original number are the same then it is a strong number.
Algorithm to Check if a Number is a Strong Number or Not in C
- Take a number from the user and store it in a variable named "num" and copy it in a temporary variable named "ori_num" we have stored it in another variable to perform certain calculations.
- Now take a variable "sum=0" to store the sum of factorial of digits.
- Find the last digit of the num and store it in a variable named "last_digit = num%10".
- Now find the factorial of last_digit and then store it in a variable named "factorial".
- Now, add the factorial to the sum i.e. sum = sum + factorial.
- Remove the last digit from num because it will not be used further.
- Now repeat the above steps until num > 0.
- Check the condition for a strong number after the loop. The supplied number is strong if sum == original; else, it is not.
Examples of Programs to Find if a Number is a Strong Number or Not in C
Using Functions
In this method, we will check whether the number is strong or not by using functions.
Let's take a code and understand its concept of it:
Code
Output
Explanation In the above code first, we have taken input from the user and stored it in a temporary variable "ori_num" and then every time compute the last digit of the number and call the "factorial" for the last digit and add the number to the sum. Then at last check, the condition of the strong number that is original number is equal to the sum computed if it is equal then it is a strong number otherwise it is not.
Using for Loop
In this method, we will check whether the number is strong or not by using for loop.
Let's take a code and understand its concept of it:
Code
Output
Explanation
In the above code first, we have taken input from the user and stored it in a temporary variable "temp" and then using for a loop every time compute the last digit of the number and then compute the factorial of a number using the for loop and add the number to the sum. Then at last check, the condition of a strong number that is original number is equal to the sum computed if it is equal then it is a strong number otherwise it is not.
Using While Loop
In this method, we will check whether the number is strong or not by using a while loop.
Let's take a code and understand its concept of it:
Code
Output
Explanation
In the above code first, we have taken input from the user and stored it in a temporary variable "temp" and then used a while loop every time to compute the last digit of the number and then compute the factorial of a number using the while loop and add the number to the sum. Then at last check, the condition of a strong number that is original number is equal to the sum computed if it is equal then it is a strong number otherwise it is not.
C Program to Find Strong Numbers Between the 1 to N
In this program, we will find the strong numbers from 1 to n.
Output
Explanation
In the above code firs,t we have taken input from the user as the upper limit and then run a loop from 1 to the upper limit then using the while loop every time compute the last digit of the number and then compute the factorial of a number using the for loop and add the number to the sum. Then at last check,k the condition of the strong number that is the original number is equal to the sum computed if it is equal then it is a strong number otherwise it is not.
Conclusion
- Strong Number is the sum of the factorial of each number which results in the original number.
- Strong numbers can be implemented in several ways such as using functions, using for loops, and using a while loop.
- Strong number has the majority of the computation of factorial and sum and then if its results in the original number then it is a strong number.
- We can also find the strong number between a given range.
- Some examples of strong numbers are 1, 2, 145, and 40585