Strong Number in Python
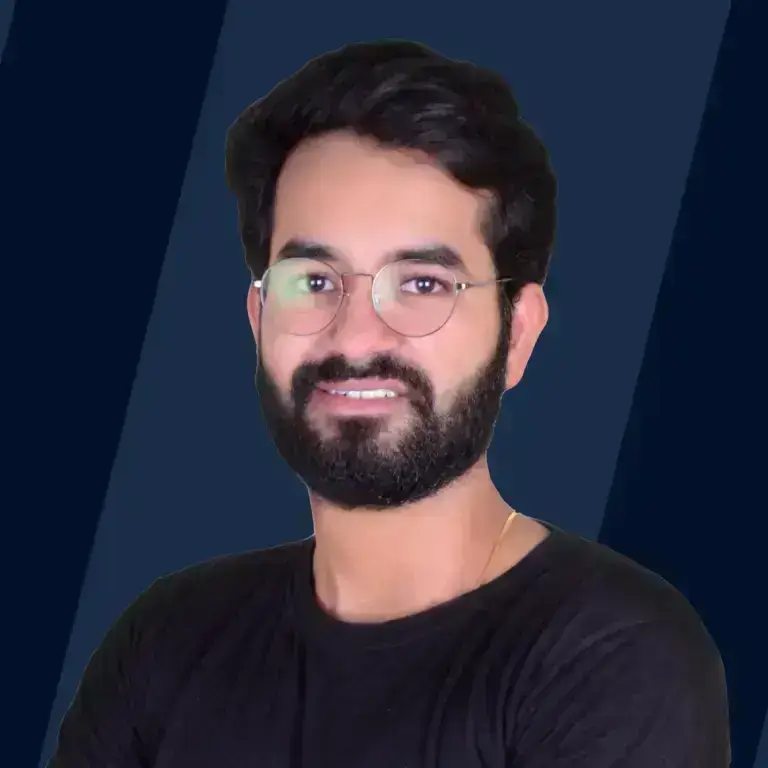
Overview
Ever heard about Strong Numbers? They are extremely rare numbers that are equal to the sum of the factorial of all their digits.
In Python, we can check for strong numbers using different methods like using a while loop, for loop and hashing, recursion, and inbuilt math factorial() function.
What is a Strong Number?
If the sum of the factorial of all the digits of a number is equal to the number itself, that number is called a Strong Number.
Confused? Okay, I'll find a better way to explain this.
Take any number, for instance, let us take . This number contains the digits , , and , their factorials will be , , and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is equal to the number itself. Therefore, the number is a strong number.
Now, let us look at another example for clarity.
This time, we will take the number . This number contains the digits , , and , their factorials will be , , and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is not equal to the number itself. Therefore, the number is not a strong number :disappointed:.
Note: The factorial of a number is the product of all the numbers from to .
It is denoted by the "!" (exclamation) sign.
Factorial of
For example,
Examples of Strong Numbers
- : It is a strong number as the sum of the factorial of all its digits () is equal to the number itself ().
- : It is a strong number as the sum of the factorial of all its digits () is equal to the number itself ().
- : It is a strong number as the sum of the factorial of all its digits () is equal to the number itself ().
The Problem
Create a Python Program to check if a given number is a strong number or not.
Approach to the Problem
- Ask the user to enter an integer number.
- Find the factorial of each digit in the given number and add them.
- Check if the sum of the factorials is equal to the inputted number.
- If yes, return "True", else return "False".
- Print the output.
- Exit.
Programs to Check if the Given Number is a Strong number in Python
In Python, there are various methods to check if a number is a strong number or not. Let us look at each of them:
Python Program to Check for Strong Number Using a While Loop
In this program, we will check for strong numbers using a while loop nested inside another while loop. The parent while loop is used to check for the strong number, and the nested while loop is used to calculate the factorial of a digit.
Steps of the Algorithm
- Initialize variable to be .
- Initialize variable to be .
- Run a while loop till is not .
- Inside the while loop, assign to be , initialize variables and to be .
- Run a nested while loop while is less than or equal to inside the main while loop.
- Inside the nested while loop, multiply by and increment by in each iteration.
- After the end of the nested while loop, add to and divide by .
- End the main while loop.
- Check if is equal to , if yes, return True.
- Return false.
Implementation
Output 1
Output 2
Explanation
In output 1, the inputted number is . This number contains the digits , , and , their factorials will be , , and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is equal to the number itself. Therefore, the number is a strong number, as can be seen in the output.
In output 2, the inputted number is . This number contains the digits , , and , their factorials will be , , and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is equal to the number itself. Therefore, the number is not a strong number, as can be seen in the output.
Python Program to Check for Strong Number Using a For Loop and a Dictionary (Pre-Determined Factorials)
In this program, we will check for strong numbers using a for loop and a dictionary.
The for loop will be used for checking strong numbers and the dictionary will be used to store the factorial of digits from to .
Steps of the Algorithm
At first, create a dictionary to store the factorial of digits from to , where the key will be the digit and the value will be its factorial.
The isStrong() function
- Initialize variable to be .
- Iterate over the number (by typecasting it into a string) using a for loop, add facto[int(x)] to sum in each iteration.
- Check if is equal to , if yes, return True.
- Return false.
Implementation
Output 1
Output 2
Explanation
In output 1, the inputted number is . This number contains a single digit , its factorial will be , and the total sum of the factorials would be . The sum of the factorial of all the digits is equal to the number itself. Therefore, the number is a strong number, as can be seen in the output.
In output 2, the inputted number is . This number contains the digits , and , their factorials will be and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is not equal to the number itself. Therefore, the number is not a strong number, as can be seen in the output.
Python Program to Check for Strong Number Using Recursion
In this program, we will check for a strong number using recursion. There will be two recursive functions in this program, isStrong() for checking strong numbers and factorial() for calculating the factorial of a number.
Steps of the Algorithm
The function It returns the factorial of the number passed to it.
- Check if is less than or equal to , if yes, return .
- If no, return .
Initialize a global variable to be .
The function
It returns or for a given number is a strong number or not.
- Check if is not equal to , if true, assign to be , to be , add to , and lastly, call the function by passing to it.
- Check if is equal to , if yes, return True.
- Return false.
Implementation
Output 1
Output 2
Explanation
In output 1, the inputted number is . This number contains the digits , , , , and , their factorials will be , , , , and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is equal to the number itself. Therefore, the number is a strong number, as can be seen in the output.
In output 2, the inputted number is . This number contains the digits , , , , and , their factorials will be , , , , and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is not equal to the number itself. Therefore, the number is not a strong number, as can be seen in the output.
Python Program to Check for Strong Number Using the Math factorial() Function
In this program, we will check for the strong numbers using a while loop, inside which we will use the math factorial() function to calculate the factorial of a digit.
Steps of the Algorithm
Import math module.
The function
- Initialize variable to be .
- Initialize variable to be .
- Run a while loop till is not .
- Inside the while loop, assign to be .
- Assign to be .
- Add to and divide by .
- End the while loop.
- Check if is equal to , if yes, return True.
- Return False.
Implementation
Output 1
Output 2
Explanation
In output 1, the inputted number is . This number contains a single digit , its factorial will be , and the total sum of the factorials would be . The sum of the factorial of all the digits is equal to the number itself. Therefore, the number is a strong number, as can be seen in the output.
In output 2, the inputted number is . This number contains the digits and , their factorials will be and respectively, and the total sum of the factorials would be . The sum of the factorial of all the digits is not equal to the number itself. Therefore, the number is not a strong number, as can be seen in the output.
Check out this article to learn about Math Module in Python.
Conclusion
- Strong numbers are integer numbers whose sum of the factorial of all their digits is equal to them.
- Factorial of a number is equal to .
- The math factorial() function returns the factorial of the number passed to it.
- There are 4 ways to check if the given number is a strong number or not: Using While loops, Using For loop, Using Recursion, and Using the factorial() function.