strrev() Function in C
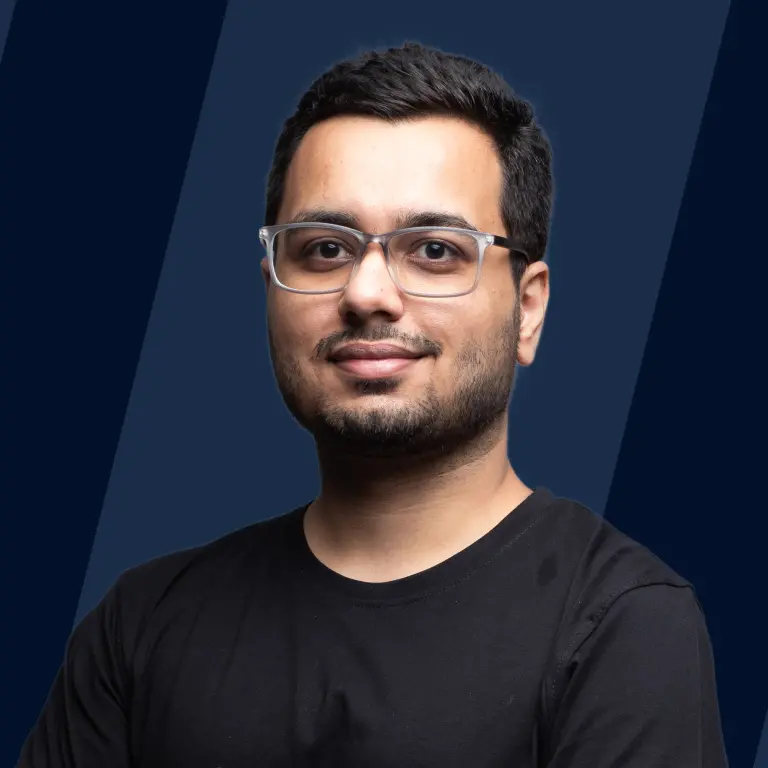
Overview
Sometimes in our program, we are in a use case to reverse a string, In C language we can use the function strrev() present in the header file string.h to reverse any given string, strrev() is a non-standard string library function ie. it is not standardly available with all the compilers, only some specific compilers support it, but we can write our own implementation of a function to a reverse a string, if the function strrev() is not available.
Syntax of the Function strrev in C
Parameters of the Function strrev in C
strrev() function in C only takes a single parameter which is a pointer to a character array which we have to reverse.
Return Value of the Function strrev in C
Function strrev() returns the same string in reverse order, ie. it does not creates a new string, instead, it reverses the original string and returns the same.
Example
Output:
Explanation:
- We are initializing a char array with "Hello World" and printing it in its original form.
- Then after, we are calling the strrev() function to reverse the above array.
- And finally printing the reversed array.
Exceptions of the Function strrev in C
There are as such no specific exceptions to the function strrev().
What is strrev() Function in C?
The function strrev() is a non-standard string library function in C language that we can use to reverse a string, being non-standard indicates, that it is not a standardly available function, meaning it may not be available with all the compilers and only specific ones will support it. We can write our own implementation of the function strrev() as well if it is not available.
Note -
- strrev() does not creates a new array, instead, it reverses and replaces the original array itself.
- We can run strrev() two times to get our original array.
More Examples
1. Taking User Input and Reversing it Using the Function strrev in c
Input: Hello World
Output:
Explanation:
- We are declaring a char array str with size 20.
- Then after, we are asking the user to enter a string and storing that value into str.
- Now we are printing the array in its original form.
- Then after, calling the strrev() function to reverse the string given by the user.
- And finally printing the reversed string.
2. Writing our own Implementation to Reverse a String if strrev() is not Found
As we know the function strrev() is a non-standard string library function, meaning it is not necessary, that it will be present on every machine, so we can write our own implementation methods to reverse any given string, Let's see how
Input: Hello World
Output:
Explanation:
- We are declaring a char array str with size 100, and are asking the user to enter a string, storing that into the array str and are printing it in its original form.
- After that we are calculating the length of the given array using a while loop, by iterating it through the passed array, till it reaches the null character ie. the end of the string
- Then using a for loop, we are reversing the array by interchanging the first and last pair of terms, and after lenght/2 iterations, the whole array will be reversed. What if we iterate till complete length ?, In that case, our array will be reversed till the initial length/2 iterations, and then again it will be reversed in the next length/2 iteration, meaning we will get the same array again.
- Once reversed, we are finally printing the reversed array.
To read more about reversing a string in C visit here.
Conclusion
- strrev() is a non-standard string library function in C language, which we can use to reverse a string.
- The reversed string is stored in the same string ie, strrev() does not creates a new string, it reverses and replaces the original string itself.
- Syntax : char *strrev(char *str);
- *char str : is the pointer to the char array which we have to reverse.
- The function strrev in c accepts only one parameter of type char pointer and it returns a character pointer as well.
- It is non-standard, meaning it is not necessary that it will be available in every compiler, and if it is not available, then we can write our own implementation methods to reverse a given string.