Struct in Python
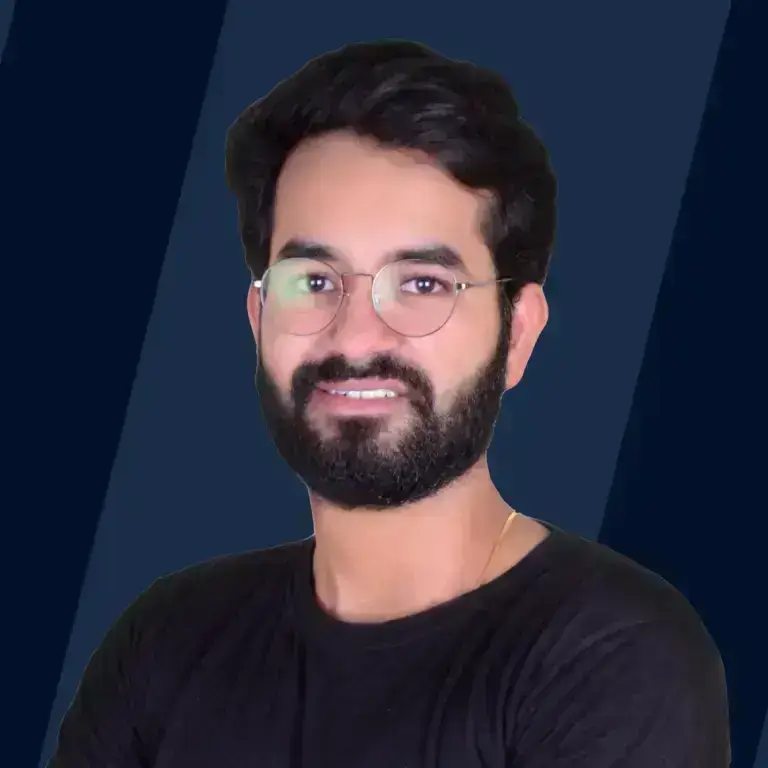
Overview
The struct in Python is used to perform the conversion of the C struct (which is represented as a Python byte object) into a Python string of bytes and vice versa. The struct in Python is used for file handling in the case of binary-stored data. The conversion of C structs into Python form is done using format strings.
Struct in Python
As discussed earlier, struct in Python performs the conversion of Python values and C structs that are represented in Python byte object form.
General Syntax of Struct in Python
- The module refers to the module used for conversion among C structs and Python values.
- The struct in Python makes the use of format string as compact descriptions of the layout of the C structs and the intended conversion to/from Python values.
What are format strings?
Format strings are the mechanism used to specify the expected layout when packing and unpacking data. They are built up from Format Characters, which define the type of data being packed/unpacked. In addition, there are special characters for controlling the Byte Order, Size, and Alignment.
- The buffer is passed as an argument to multiple structs in Python. The buffer is used to refer to the object which is used in the implementation of the buffer protocols. These objects are used to implement the buffer protocols in readable or read-writable form.
Struct Module in Python
In this section, we will go through some struct modules in Python:
struct.pack()
The struct.pack() module in Python is used to return an object of bytes that consists of the values that are loaded based on the format string manner.
The arguments must match the values required by the format exactly.
Syntax:
struct.unpack()
The struct.unpack() module in Python is used for unpacking of buffer from the start at position offset by the format string manner. The struct.unpack() module returns a tuple.
The buffer’s size in bytes, starting at position offset, must be at least the size required by the format, as reflected by calcsize().
Syntax:
struct.calcsize()
The struct.calcsize() module is used to return the struct's size that corresponds to the format string manner. The struct.calcsize() can be used with modules like struct.unpack_from() or struct.pack_into() that need buffer as well as offset values.
Syntax:
Exception struct.error
The struct.error is used to raise exceptions. It describes what is the problem with arguments that are passed.
struct.pack_into()
The struct.pack_into() is used for packing values according to the format string manner. The packed values are written into the writable buffer from the start at the position offset.
offset is a required argument
Syntax:
struct.unpack_from()
The struct.unpack_from() is used for unpacking the buffer from the start at position offset according to the format string manner. The struct.unpack_from() module returns a tuple.
The buffer’s size in bytes, starting at position offset, must be at least the size required by the format, as reflected by calcsize()
Syntax:
Examples for Understanding Struct Module in Python
In this section we will go through some examples to understand Python modules:
struct.pack()
In this example, we will learn how to use the struct in Python to pack any data into its binary form.
Code:
Output:
Explanation of the example:
In the above example, we are importing the struct module. The format string 'i 4s f' is passed to the struck.pack() method and the object of bytes that are loaded with values are stored in the packed variable. Thus the output displays b'\n\x00\x00\x00John\x00@\x1cE'.
struct.unpack()
In this example, we will learn how to use the struck in Python to unpack any data from its binary form.
Code:
Output:
Explanation of the example:
In the above example, we are importing the struct module. The format string 'i 4s f' is passed to the struck.unpack() method along with the object of bytes that loaded with values is stored in the packed variable. The struct.unpack() is returning the tuple associated with the format string and the packed values thus the output is (10, b'John', 2500.0)
struct.calcsize()
In this example, we will learn how to use the struck in Python to calculate the size of the given struct's string representation.
Code:
Output:
Explanation of the example:
In the above example, we are importing the struct module. The format string 'i 4s f' is passed to the struct.calcsize() method which will calculate the size of the format string. The size is calculated in bytes and stored in the size variable. Thus the output is 12 bytes.
struct.pack_into()
In this example, we will learn how to work with the pack_into() method of struck in Python.
Code:
Output:
Explanation of the example:
In the above example, firstly we will import struct and ctypes modules since this example uses functions that need these modules. Here we have created a buffer size string with the name Buffer which is of size 8 and we are getting our packed values in the buffer string. The buffer string when passed into the struct.pack_into() method along with other parameters will return packed data which outputs b'\x01\x00\x00\x00\x02\x00\x00\x00'.
struct.unpack_from()
Output:
Explanation of the example:
In the above example, firstly we will import struct and ctypes modules since this example uses functions that need these modules. Here we have created a buffer size string with the name Buffer which is of size 11 and we are getting our packed values in the buffer string. The buffer string when passed into the struct.pack_into() method along with other parameters will return packed data.
Now we will pass that packed data to our struct.unpack_from() function which will return (1, 2, 3).
Conclusion
- The struct in Python performs the conversion of Python values and C structs.
- The module of struct in Python refers to the module used for conversion among C structs and Python values.
- The struct in Python uses format string for conversion of C structs to Python values and vice versa.
- The buffer is used to refer to the object which is used in the implementation of the buffer protocols.