Structure in C++
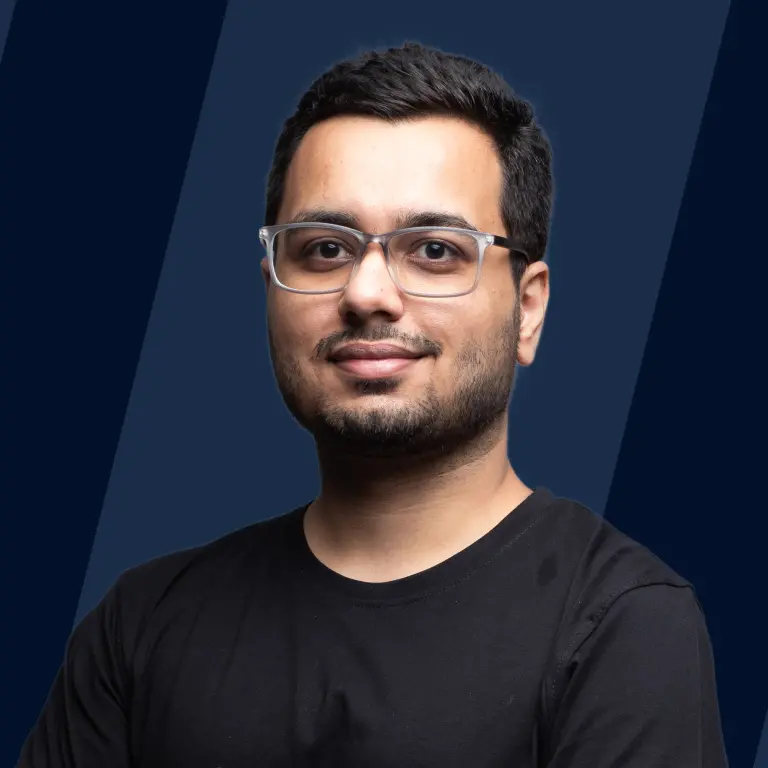
Structure in C++ group related data, making code easier to manage. They're great for combining different data types, like student details, improving code clarity and ease of use. Structures show how to effectively handle complex data sets.
What is a Structure in C++?
Structure in C++ lets you group different pieces of data together into one unit. This is handy when you have related data that you want to keep together, like a student's name, roll number, and marks. Instead of using separate arrays for each piece of information, which can get complicated, structures make it simpler to work with all these details at once, improving organization and making your code easier to understand and manage.
How to Create a Structure?
To create a structure in C++, use the struct keyword, followed by the structure name and a set of curly braces containing the data members' definitions. Each member definition consists of a data type followed by a member name. End the structure definition with a semicolon.
Syntax:
Types of Members in a Structure?
In a structure in C++, there are primarily two types of members:
-
Data Members: These are the variables that hold data. Each data member can have a different data type, such as int, string, float, etc. Data members represent the attributes of the structure.
-
Member Functions (Methods): These are functions defined inside the structure that operate on or manipulate the data members, providing functionality to the structure. Member functions can access both static and non-static data members and can be defined inside or outside the structure definition.
Example:
In this Student structure, name and age are data members, while display is a member function that prints the student's details.
How to Declare Structure Variables in C++?
In C++, we have two ways through which we can declare Struct in c++.
1. Declaration at the time of Structure creation
2. Declaring after the structure creation (like any normal variable)
How to Initialize Structure Members?
We can initialize the structure variables in the following ways
1. We can intialize them with default values for each data member at the time of defining
2. We can initialize them after we have created the structure variable
We can also initialize all the members of a structure at once using {}
How to Access Structure Elements?
We can easily access the structure members using the dot (.) operator
What is an Array of Structures?
As we have understood till now that Struct in c++ are nothing but are just like a normal datatype that we create by ourselves as per our use case. Just like we create arrays for any of the int, char, float, double etc. we can create the array for structures as well
What is a Structure Pointer?
Just like we have pointers (pointer variables) for int, char, float, double etc. Similarly, we have pointers for structure datatypes as well. As pointers are only a variable, a speical type of variable that are used to store the address of another variable and structures are also allocated memory into the RAM, they also have the memory address, so we have pointers for structures too. We access them using the arrow (->) operator
Explanation: As we know to declare an integer pointer we do it like int a; and then int *arr = &a;, Similarly to declare a structure pointer we will do it as Employee e1; and Employee *ptrE1 = &e1;
What is Structure Member Alignment?
Structure member alignment in C++ deals with how the compiler arranges the structure's members in memory. The aim is to align members in a way that matches the hardware's word size, optimizing access speed and reducing padding (unused memory spaces). Misaligned members might cause more memory reads, slowing down the program. The compiler may automatically add padding between members to ensure alignment, which can affect the overall size of the structure. Understanding and managing member alignment can help in optimizing memory usage and performance in C++ applications.
For example, in a structure like Employee with an int and a string, the compiler might align the int on a 4-byte boundary and add padding before the string to align it on an 8-byte boundary for a 64-bit system, depending on the compiler and system architecture.
Conclusion
- Structure in c++ are a custom datatype created by us that stores different datatypes and makes them as one unit
- We can create a Structure using the struct keyword
- We use the dot (.) operator to access the members of a structure
- In the case of structure pointers, we use the arrow (->) operator for accessing the members, structure pointers are just like regular pointers, which are meant to store the address of the structure variables
- We can pass Structure in c++ as a function argument just like we pass any other primitive datatype
Note: Struct in c++ are nothing more than just a datatype like int, char, float, double etc. Wherever you see Employee e1, Student s1 etc. just treat them like int a;, understand it this way and they will become so simple